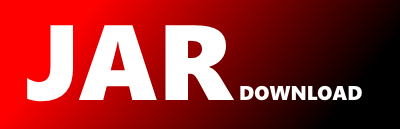
net.sf.aguacate.script.InternalScriptCoupling Maven / Gradle / Ivy
package net.sf.aguacate.script;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.nio.charset.StandardCharsets;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import net.sf.aguacate.script.spi.JavascriptInternalScriptFactory;
import net.sf.aguacate.util.resource.ResourceLocator;
import net.sf.aguacate.util.resource.impl.ResourceLocatorClassImpl;
import net.sf.aguacate.util.resource.impl.ResourceLocatorFileImpl;
public final class InternalScriptCoupling {
private static final Logger LOGGER = LogManager.getLogger(InternalScriptCoupling.class);
private static final String DIRECTORY_DATASOURCE = "DIRECTORY_DATASOURCE";
private static final ResourceLocator LOCATOR;
private static final InternalScriptFactory FACTORY;
private static Map cache;
static {
FACTORY = new JavascriptInternalScriptFactory();
String temp = System.getProperty(DIRECTORY_DATASOURCE);
if (temp == null || temp.isEmpty()) {
temp = System.getenv(DIRECTORY_DATASOURCE);
if (temp == null || temp.isEmpty()) {
LOGGER.info("No " + DIRECTORY_DATASOURCE + " defined, using default");
LOCATOR = new ResourceLocatorClassImpl(InternalScriptCoupling.class);
} else {
LOGGER.info("using " + DIRECTORY_DATASOURCE + " (env): {}", temp);
LOCATOR = new ResourceLocatorFileImpl(temp);
}
} else {
LOGGER.info("using " + DIRECTORY_DATASOURCE + " (prop): {}", temp);
LOCATOR = new ResourceLocatorFileImpl(temp);
}
cache = Collections.emptyMap();
}
private InternalScriptCoupling() {
}
public static final InternalScript load(String scriptName) {
InternalScript result = cache.get(scriptName);
if (result == null) {
synchronized (InternalScriptCoupling.class) {
result = cache.get(scriptName);
if (result == null) {
Map temp = new HashMap<>(cache);
result = load0(scriptName);
temp.put(scriptName, result);
cache = temp;
}
}
}
return result;
}
static InternalScript load0(String scriptName) {
String filename = scriptName.concat(".js");
LOGGER.debug(filename);
try {
InputStream inputStream = LOCATOR.open(filename);
if (inputStream == null) {
return null;
} else {
Reader reader = new InputStreamReader(inputStream, StandardCharsets.UTF_8);
try {
return FACTORY.build(reader);
} finally {
try {
reader.close();
} catch (IOException e) {
LOGGER.warn("On closing resource", e);
}
}
}
} catch (IOException e) {
throw new IllegalStateException(filename, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy