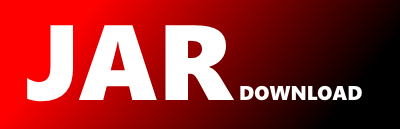
net.sf.ahtutils.jsf.menu.MenuFactory Maven / Gradle / Ivy
package net.sf.ahtutils.jsf.menu;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import net.sf.ahtutils.exception.ejb.UtilsNotFoundException;
import net.sf.ahtutils.xml.access.Access;
import net.sf.ahtutils.xml.access.Category;
import net.sf.ahtutils.xml.access.View;
import net.sf.ahtutils.xml.navigation.Menu;
import net.sf.ahtutils.xml.navigation.MenuItem;
import net.sf.ahtutils.xml.navigation.UrlMapping;
import net.sf.ahtutils.xml.status.Lang;
import net.sf.ahtutils.xml.xpath.AccessXpath;
import net.sf.ahtutils.xml.xpath.NavigationXpath;
import net.sf.exlp.util.exception.ExlpXpathNotFoundException;
import net.sf.exlp.util.exception.ExlpXpathNotUniqueException;
import org.jgrapht.DirectedGraph;
import org.jgrapht.alg.DijkstraShortestPath;
import org.jgrapht.graph.DefaultDirectedGraph;
import org.jgrapht.graph.DefaultEdge;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MenuFactory
{
final static Logger logger = LoggerFactory.getLogger(MenuFactory.class);
private String lang;
private String contextRoot;
public void setContextRoot(String contextRoot) {this.contextRoot = contextRoot;}
private Access access;
private boolean noRestrictions;
private Map mapViewAllowed;
private Map translationsMenu,translationsAccess;
private Map mapView;
private Map mapMenuItems;
private String rootNode;
private DirectedGraph graph;
private int alwaysUpToLevel;
public MenuFactory(Menu menu,String lang){this(menu,null,lang, UUID.randomUUID().toString(),true);}
public MenuFactory(Menu menu,String lang, String rootNode){this(menu,null,lang,rootNode,true);}
public MenuFactory(Menu menu, Access access,String lang){this(menu,access,lang, UUID.randomUUID().toString(),false);}
public MenuFactory(Menu menu, Access access,String lang, String rootNode){this(menu,access,lang, rootNode,false);}
public MenuFactory(Menu menu, Access access,String lang, String rootNode, boolean noRestrictions)
{
this.access=access;
this.lang=lang;
this.rootNode=rootNode;
this.noRestrictions=noRestrictions;
translationsMenu = new Hashtable();
mapMenuItems = new Hashtable();
processMenu(menu);
if(logger.isTraceEnabled())
{
logger.info("Graph: "+graph);
logger.info("mapMenuItems.size()"+mapMenuItems.size());
}
mapView = new Hashtable();
translationsAccess = new Hashtable();
if(access!=null){createAccessMaps();}
alwaysUpToLevel = 1;
}
private void processMenu(Menu menu)
{
graph = new DefaultDirectedGraph(DefaultEdge.class);
graph.addVertex(rootNode);
logger.trace("Added Root: "+rootNode);
for(MenuItem mi : menu.getMenuItem())
{
processMenuItem(rootNode,mi);
}
}
private void processMenuItem(String parentNode, MenuItem mi)
{
graph.addVertex(mi.getCode());
graph.addEdge(parentNode, mi.getCode());
logger.trace("Added Vertex: "+mi.getCode());
if(mi.isSetLangs())
{
for(Lang l : mi.getLangs().getLang())
{
if(l.getKey().equals(lang)){translationsMenu.put(mi.getCode(), l.getTranslation());}
}
}
for(MenuItem miChild : mi.getMenuItem())
{
processMenuItem(mi.getCode(),miChild);
}
mi.getMenuItem().clear();
mapMenuItems.put(mi.getCode(), mi);
}
public void setAlwaysUpToLevel(int alwaysUpToLevel) {this.alwaysUpToLevel = alwaysUpToLevel;}
private void createAccessMaps()
{
for(Category c : access.getCategory())
{
if(c.isSetViews())
{
for(View v : c.getViews().getView())
{
mapView.put(v.getCode(), v);
if(v.isSetLangs())
{
for(Lang l : v.getLangs().getLang())
{
if(l.getKey().equals(lang)){translationsAccess.put(v.getCode(), l.getTranslation());}
}
}
}
}
}
}
public Menu build()
{
noRestrictions=true;
return build(null,rootNode);
}
public Menu build(String codeCurrent) {return build(null,codeCurrent);}
public Menu build(Map mapViewAllowed) {return build(mapViewAllowed,rootNode);}
public Menu build(Map mapViewAllowed, String codeCurrent){return build(mapViewAllowed,codeCurrent,false);}
public Menu build(Map mapViewAllowed, String codeCurrent, boolean isLoggedIn)
{
logger.trace("Building ("+rootNode+"): "+codeCurrent);
this.mapViewAllowed=mapViewAllowed;
Menu result = new Menu();
try {result.getMenuItem().addAll(processChilds(1,rootNode,codeCurrent,isLoggedIn));}
catch (UtilsNotFoundException e) {logger.warn(e.getMessage());}
return result;
}
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy