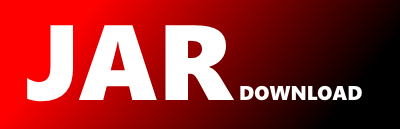
src.net.sf.beanlib.hibernate3.EnumTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beanlib-hibernate Show documentation
Show all versions of beanlib-hibernate Show documentation
Particularly handy when used with Hibernate.
It allows developers to easily reuse the same pojo classes for
both persistence instances and data transfer objects.
/*
* Copyright 2005 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License")
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.sf.beanlib.hibernate3;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotSame;
import static org.junit.Assert.assertSame;
import junit.framework.JUnit4TestAdapter;
import net.sf.beanlib.hibernate.CustomHibernateBeanTransformable;
import net.sf.beanlib.hibernate.HibernateBeanReplicator;
import net.sf.beanlib.hibernate.HibernateBeanTransformableSpi;
import org.junit.Test;
/**
* @author Joe D. Velopar
*/
public class EnumTest {
public static enum Status {
BEGIN, WIP, END;
}
public static class C {
private Status status;
private String testString;
public Status getStatus() {return status;}
public void setStatus(Status status) {this.status = status;}
public String getTestString() {return testString;}
public void setTestString(String testString) {this.testString = testString;}
}
@Test public void testCopy() {
C c = new C();
c.setStatus(Status.BEGIN);
c.setTestString("testStr");
HibernateBeanReplicator replicator = new Hibernate3BeanReplicator().initCustomTransformer(
new CustomHibernateBeanTransformable() {
public boolean isTransformable(
@SuppressWarnings("unused") Object from,
Class toClass,
@SuppressWarnings("unused") HibernateBeanTransformableSpi hibernateBeanTransformer)
{
return toClass.isEnum();
}
@SuppressWarnings("unchecked")
public T transform(Object in, @SuppressWarnings("unused") Class toClass) {return (T)in;}
});
C c2 = (C)replicator.deepCopy(c);
assertNotSame(c2, c);
assertSame(c2.getStatus(), c.getStatus());
assertEquals(c.getTestString(), c2.getTestString());
}
public static junit.framework.Test suite() {
return new JUnit4TestAdapter(EnumTest.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy