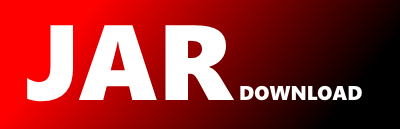
javax.bluetooth.L2CAPConnectionNotifier Maven / Gradle / Ivy
Show all versions of bluecove Show documentation
/**
* BlueCove - Java library for Bluetooth
*
* Java docs licensed under the Apache License, Version 2.0
* http://www.apache.org/licenses/LICENSE-2.0
* (c) Copyright 2001, 2002 Motorola, Inc. ALL RIGHTS RESERVED.
*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*
* @version $Id: L2CAPConnectionNotifier.java 2530 2008-12-09 18:52:53Z skarzhevskyy $
*/
package javax.bluetooth;
import java.io.IOException;
import javax.microedition.io.Connection;
/**
* The L2CAPConnectionNotifier
interface provides
* an L2CAP connection notifier.
*
* To create a server connection, the protocol must be
* btl2cap
. The target contains "localhost:" and the UUID of the
* service. The parameters are ReceiveMTU and TransmitMTU, the same parameters
* used to define a client connection. Here is an example of a valid server connection
* string:
* btl2cap://localhost:3B9FA89520078C303355AAA694238F07;ReceiveMTU=512;TransmitMTU=512
*
* A call to Connector.open() with this string will return a
* javax.bluetooth.L2CAPConnectionNotifier
object. An
* L2CAPConnection
object is obtained from the
* L2CAPConnectionNotifier
by calling the method
* acceptAndOpen()
.
*
*/
public interface L2CAPConnectionNotifier extends Connection {
/**
* Waits for a client to connect to this L2CAP service. Upon connection
* returns an L2CAPConnection
that can be used to communicate
* with this client.
*
*
* A service record associated with this connection will be added to the
* SDDB associated with this L2CAPConnectionNotifier
object if
* one does not exist in the SDDB. This method will put the local device in
* connectable mode so that it may respond to connection attempts by
* clients.
*
*
* The following checks are done to verify that any modifications made by
* the application to the service record after it was created by
* Connector.open()
have not created an invalid service record.
* If any of these checks fail, then a
* ServiceRegistrationException
is thrown.
*
* - ServiceClassIDList and ProtocolDescriptorList, the mandatory service
* attributes for a
btl2cap
service record, must be present in
* the service record.
* - L2CAP must be in the ProtocolDescriptorList.
*
- The PSM value must not have changed in the service record.
*
*
* This method will not ensure that the service record created is a
* completely valid service record. It is the responsibility of the
* application to ensure that the service record follows all of the
* applicable syntactic and semantic rules for service record correctness.
*
* Note : once an application invokes close()
on any
* L2CAPConnectionNotifier
, SessionNotifier
, or
* StreamConnectionNotifer
instance, all pending
* acceptAndOpen()
methods that have been invoked previously on
* that instance MUST throw InterruptedIOException
. This
* mechanism provides an application with the means to cancel any
* outstanding acceptAndOpen()
method calls.
*
* @return a connection to communicate with the client
*
* @exception IOException
* if the notifier is closed before
* acceptAndOpen()
is called
*
* @exception ServiceRegistrationException
* if the structure of the associated service record is
* invalid or if the service record could not be added
* successfully to the local SDDB. The structure of service
* record is invalid if the service record is missing any
* mandatory service attributes, or has changed any of the
* values described above which are fixed and cannot be
* changed. Failures to add the record to the SDDB could be
* due to insufficient disk space, database locks, etc.
*
* @exception BluetoothStateException
* if the server device could not be placed in connectable
* mode because the device user has configured the device to
* be non-connectable.
*
*/
public L2CAPConnection acceptAndOpen() throws IOException;
}