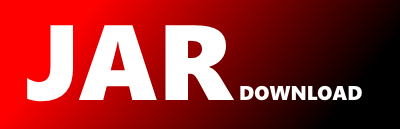
net.sf.buildbox.changes.bean.impl.ChangesDocumentBeanImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of releasator Show documentation
Show all versions of releasator Show documentation
Commandline utility for creating reproducible releases. Minimal parametrization, isolated sandbox for releases. Currently built on top of maven-release-plugin.
The newest version!
/*
* An XML document type.
* Localname: changes
* Namespace: http://buildbox.sf.net/changes/2.0
* Java type: net.sf.buildbox.changes.bean.ChangesDocumentBean
*
* Automatically generated - do not modify.
*/
package net.sf.buildbox.changes.bean.impl;
/**
* A document containing one changes(@http://buildbox.sf.net/changes/2.0) element.
*
* This is a complex type.
*/
public class ChangesDocumentBeanImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.sf.buildbox.changes.bean.ChangesDocumentBean
{
private static final long serialVersionUID = 1L;
public ChangesDocumentBeanImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName CHANGES$0 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "changes");
/**
* Gets the "changes" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes getChanges()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes)get_store().find_element_user(CHANGES$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "changes" element
*/
public void setChanges(net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes changes)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes)get_store().find_element_user(CHANGES$0, 0);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes)get_store().add_element_user(CHANGES$0);
}
target.set(changes);
}
}
/**
* Appends and returns a new empty "changes" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes addNewChanges()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes)get_store().add_element_user(CHANGES$0);
return target;
}
}
/**
* An XML changes(@http://buildbox.sf.net/changes/2.0).
*
* This is a complex type.
*/
public static class ChangesImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes
{
private static final long serialVersionUID = 1L;
public ChangesImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName CONFIGURATION$0 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "configuration");
private static final javax.xml.namespace.QName KNOWNISSUES$2 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "known-issues");
private static final javax.xml.namespace.QName UNRELEASED$4 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "unreleased");
private static final javax.xml.namespace.QName LOCALBUILD$6 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "localbuild");
private static final javax.xml.namespace.QName RELEASE$8 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "release");
/**
* Gets the "configuration" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration getConfiguration()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration)get_store().find_element_user(CONFIGURATION$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "configuration" element
*/
public boolean isSetConfiguration()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(CONFIGURATION$0) != 0;
}
}
/**
* Sets the "configuration" element
*/
public void setConfiguration(net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration configuration)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration)get_store().find_element_user(CONFIGURATION$0, 0);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration)get_store().add_element_user(CONFIGURATION$0);
}
target.set(configuration);
}
}
/**
* Appends and returns a new empty "configuration" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration addNewConfiguration()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration)get_store().add_element_user(CONFIGURATION$0);
return target;
}
}
/**
* Unsets the "configuration" element
*/
public void unsetConfiguration()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(CONFIGURATION$0, 0);
}
}
/**
* Gets the "known-issues" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues getKnownIssues()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues)get_store().find_element_user(KNOWNISSUES$2, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "known-issues" element
*/
public boolean isSetKnownIssues()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(KNOWNISSUES$2) != 0;
}
}
/**
* Sets the "known-issues" element
*/
public void setKnownIssues(net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues knownIssues)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues)get_store().find_element_user(KNOWNISSUES$2, 0);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues)get_store().add_element_user(KNOWNISSUES$2);
}
target.set(knownIssues);
}
}
/**
* Appends and returns a new empty "known-issues" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues addNewKnownIssues()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues)get_store().add_element_user(KNOWNISSUES$2);
return target;
}
}
/**
* Unsets the "known-issues" element
*/
public void unsetKnownIssues()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(KNOWNISSUES$2, 0);
}
}
/**
* Gets the "unreleased" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased getUnreleased()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased)get_store().find_element_user(UNRELEASED$4, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "unreleased" element
*/
public boolean isSetUnreleased()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(UNRELEASED$4) != 0;
}
}
/**
* Sets the "unreleased" element
*/
public void setUnreleased(net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased unreleased)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased)get_store().find_element_user(UNRELEASED$4, 0);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased)get_store().add_element_user(UNRELEASED$4);
}
target.set(unreleased);
}
}
/**
* Appends and returns a new empty "unreleased" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased addNewUnreleased()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased)get_store().add_element_user(UNRELEASED$4);
return target;
}
}
/**
* Unsets the "unreleased" element
*/
public void unsetUnreleased()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(UNRELEASED$4, 0);
}
}
/**
* Gets the "localbuild" element
*/
public net.sf.buildbox.changes.bean.BuiltVersionNotesBean getLocalbuild()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.BuiltVersionNotesBean target = null;
target = (net.sf.buildbox.changes.bean.BuiltVersionNotesBean)get_store().find_element_user(LOCALBUILD$6, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "localbuild" element
*/
public boolean isSetLocalbuild()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(LOCALBUILD$6) != 0;
}
}
/**
* Sets the "localbuild" element
*/
public void setLocalbuild(net.sf.buildbox.changes.bean.BuiltVersionNotesBean localbuild)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.BuiltVersionNotesBean target = null;
target = (net.sf.buildbox.changes.bean.BuiltVersionNotesBean)get_store().find_element_user(LOCALBUILD$6, 0);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.BuiltVersionNotesBean)get_store().add_element_user(LOCALBUILD$6);
}
target.set(localbuild);
}
}
/**
* Appends and returns a new empty "localbuild" element
*/
public net.sf.buildbox.changes.bean.BuiltVersionNotesBean addNewLocalbuild()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.BuiltVersionNotesBean target = null;
target = (net.sf.buildbox.changes.bean.BuiltVersionNotesBean)get_store().add_element_user(LOCALBUILD$6);
return target;
}
}
/**
* Unsets the "localbuild" element
*/
public void unsetLocalbuild()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(LOCALBUILD$6, 0);
}
}
/**
* Gets array of all "release" elements
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release[] getReleaseArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(RELEASE$8, targetList);
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release[] result = new net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "release" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release getReleaseArray(int i)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release)get_store().find_element_user(RELEASE$8, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "release" element
*/
public int sizeOfReleaseArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(RELEASE$8);
}
}
/**
* Sets array of all "release" element
*/
public void setReleaseArray(net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release[] releaseArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(releaseArray, RELEASE$8);
}
}
/**
* Sets ith "release" element
*/
public void setReleaseArray(int i, net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release release)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release)get_store().find_element_user(RELEASE$8, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(release);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "release" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release insertNewRelease(int i)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release)get_store().insert_element_user(RELEASE$8, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "release" element
*/
public net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release addNewRelease()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release target = null;
target = (net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release)get_store().add_element_user(RELEASE$8);
return target;
}
}
/**
* Removes the ith "release" element
*/
public void removeRelease(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(RELEASE$8, i);
}
}
/**
* An XML configuration(@http://buildbox.sf.net/changes/2.0).
*
* This is a complex type.
*/
public static class ConfigurationImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Configuration
{
private static final long serialVersionUID = 1L;
public ConfigurationImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName PROPERTY$0 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "property");
/**
* Gets array of all "property" elements
*/
public net.sf.buildbox.changes.bean.ConfigurationPropertyBean[] getPropertyArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(PROPERTY$0, targetList);
net.sf.buildbox.changes.bean.ConfigurationPropertyBean[] result = new net.sf.buildbox.changes.bean.ConfigurationPropertyBean[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "property" element
*/
public net.sf.buildbox.changes.bean.ConfigurationPropertyBean getPropertyArray(int i)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ConfigurationPropertyBean target = null;
target = (net.sf.buildbox.changes.bean.ConfigurationPropertyBean)get_store().find_element_user(PROPERTY$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "property" element
*/
public int sizeOfPropertyArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(PROPERTY$0);
}
}
/**
* Sets array of all "property" element
*/
public void setPropertyArray(net.sf.buildbox.changes.bean.ConfigurationPropertyBean[] propertyArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(propertyArray, PROPERTY$0);
}
}
/**
* Sets ith "property" element
*/
public void setPropertyArray(int i, net.sf.buildbox.changes.bean.ConfigurationPropertyBean property)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ConfigurationPropertyBean target = null;
target = (net.sf.buildbox.changes.bean.ConfigurationPropertyBean)get_store().find_element_user(PROPERTY$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(property);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "property" element
*/
public net.sf.buildbox.changes.bean.ConfigurationPropertyBean insertNewProperty(int i)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ConfigurationPropertyBean target = null;
target = (net.sf.buildbox.changes.bean.ConfigurationPropertyBean)get_store().insert_element_user(PROPERTY$0, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "property" element
*/
public net.sf.buildbox.changes.bean.ConfigurationPropertyBean addNewProperty()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ConfigurationPropertyBean target = null;
target = (net.sf.buildbox.changes.bean.ConfigurationPropertyBean)get_store().add_element_user(PROPERTY$0);
return target;
}
}
/**
* Removes the ith "property" element
*/
public void removeProperty(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(PROPERTY$0, i);
}
}
}
/**
* An XML known-issues(@http://buildbox.sf.net/changes/2.0).
*
* This is a complex type.
*/
public static class KnownIssuesImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.KnownIssues
{
private static final long serialVersionUID = 1L;
public KnownIssuesImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName ITEM$0 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "item");
/**
* Gets array of all "item" elements
*/
public net.sf.buildbox.changes.bean.ItemBean[] getItemArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(ITEM$0, targetList);
net.sf.buildbox.changes.bean.ItemBean[] result = new net.sf.buildbox.changes.bean.ItemBean[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "item" element
*/
public net.sf.buildbox.changes.bean.ItemBean getItemArray(int i)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean target = null;
target = (net.sf.buildbox.changes.bean.ItemBean)get_store().find_element_user(ITEM$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "item" element
*/
public int sizeOfItemArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(ITEM$0);
}
}
/**
* Sets array of all "item" element
*/
public void setItemArray(net.sf.buildbox.changes.bean.ItemBean[] itemArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(itemArray, ITEM$0);
}
}
/**
* Sets ith "item" element
*/
public void setItemArray(int i, net.sf.buildbox.changes.bean.ItemBean item)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean target = null;
target = (net.sf.buildbox.changes.bean.ItemBean)get_store().find_element_user(ITEM$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(item);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "item" element
*/
public net.sf.buildbox.changes.bean.ItemBean insertNewItem(int i)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean target = null;
target = (net.sf.buildbox.changes.bean.ItemBean)get_store().insert_element_user(ITEM$0, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "item" element
*/
public net.sf.buildbox.changes.bean.ItemBean addNewItem()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean target = null;
target = (net.sf.buildbox.changes.bean.ItemBean)get_store().add_element_user(ITEM$0);
return target;
}
}
/**
* Removes the ith "item" element
*/
public void removeItem(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(ITEM$0, i);
}
}
}
/**
* An XML unreleased(@http://buildbox.sf.net/changes/2.0).
*
* This is a complex type.
*/
public static class UnreleasedImpl extends net.sf.buildbox.changes.bean.impl.VersionNotesBeanImpl implements net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Unreleased
{
private static final long serialVersionUID = 1L;
public UnreleasedImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
}
/**
* An XML release(@http://buildbox.sf.net/changes/2.0).
*
* This is a complex type.
*/
public static class ReleaseImpl extends net.sf.buildbox.changes.bean.impl.BuiltVersionNotesBeanImpl implements net.sf.buildbox.changes.bean.ChangesDocumentBean.Changes.Release
{
private static final long serialVersionUID = 1L;
public ReleaseImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy