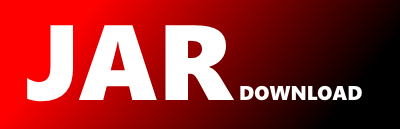
net.sf.buildbox.changes.bean.impl.ItemBeanImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of releasator Show documentation
Show all versions of releasator Show documentation
Commandline utility for creating reproducible releases. Minimal parametrization, isolated sandbox for releases. Currently built on top of maven-release-plugin.
The newest version!
/*
* XML Type: Item
* Namespace: http://buildbox.sf.net/changes/2.0
* Java type: net.sf.buildbox.changes.bean.ItemBean
*
* Automatically generated - do not modify.
*/
package net.sf.buildbox.changes.bean.impl;
/**
* An XML Item(@http://buildbox.sf.net/changes/2.0).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.ItemBean.
*/
public class ItemBeanImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.ItemBean
{
private static final long serialVersionUID = 1L;
public ItemBeanImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, true);
}
protected ItemBeanImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
private static final javax.xml.namespace.QName ACTION$0 =
new javax.xml.namespace.QName("", "action");
private static final javax.xml.namespace.QName ISSUE$2 =
new javax.xml.namespace.QName("", "issue");
private static final javax.xml.namespace.QName COMPONENT$4 =
new javax.xml.namespace.QName("", "component");
private static final javax.xml.namespace.QName TIMESTAMP$6 =
new javax.xml.namespace.QName("", "timestamp");
private static final javax.xml.namespace.QName REPORTER$8 =
new javax.xml.namespace.QName("", "reporter");
private static final javax.xml.namespace.QName RESOLVER$10 =
new javax.xml.namespace.QName("", "resolver");
private static final javax.xml.namespace.QName PRIORITY$12 =
new javax.xml.namespace.QName("", "priority");
/**
* Gets the "action" attribute
*/
public net.sf.buildbox.changes.bean.ItemBean.Action.Enum getAction()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ACTION$0);
if (target == null)
{
return null;
}
return (net.sf.buildbox.changes.bean.ItemBean.Action.Enum)target.getEnumValue();
}
}
/**
* Gets (as xml) the "action" attribute
*/
public net.sf.buildbox.changes.bean.ItemBean.Action xgetAction()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Action target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Action)get_store().find_attribute_user(ACTION$0);
return target;
}
}
/**
* True if has "action" attribute
*/
public boolean isSetAction()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(ACTION$0) != null;
}
}
/**
* Sets the "action" attribute
*/
public void setAction(net.sf.buildbox.changes.bean.ItemBean.Action.Enum action)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ACTION$0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(ACTION$0);
}
target.setEnumValue(action);
}
}
/**
* Sets (as xml) the "action" attribute
*/
public void xsetAction(net.sf.buildbox.changes.bean.ItemBean.Action action)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Action target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Action)get_store().find_attribute_user(ACTION$0);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ItemBean.Action)get_store().add_attribute_user(ACTION$0);
}
target.set(action);
}
}
/**
* Unsets the "action" attribute
*/
public void unsetAction()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(ACTION$0);
}
}
/**
* Gets the "issue" attribute
*/
public java.lang.String getIssue()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ISSUE$2);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "issue" attribute
*/
public net.sf.buildbox.changes.bean.ItemBean.Issue xgetIssue()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Issue target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Issue)get_store().find_attribute_user(ISSUE$2);
return target;
}
}
/**
* True if has "issue" attribute
*/
public boolean isSetIssue()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(ISSUE$2) != null;
}
}
/**
* Sets the "issue" attribute
*/
public void setIssue(java.lang.String issue)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ISSUE$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(ISSUE$2);
}
target.setStringValue(issue);
}
}
/**
* Sets (as xml) the "issue" attribute
*/
public void xsetIssue(net.sf.buildbox.changes.bean.ItemBean.Issue issue)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Issue target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Issue)get_store().find_attribute_user(ISSUE$2);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ItemBean.Issue)get_store().add_attribute_user(ISSUE$2);
}
target.set(issue);
}
}
/**
* Unsets the "issue" attribute
*/
public void unsetIssue()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(ISSUE$2);
}
}
/**
* Gets the "component" attribute
*/
public java.lang.String getComponent()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(COMPONENT$4);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "component" attribute
*/
public net.sf.buildbox.changes.bean.ItemBean.Component xgetComponent()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Component target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Component)get_store().find_attribute_user(COMPONENT$4);
return target;
}
}
/**
* True if has "component" attribute
*/
public boolean isSetComponent()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(COMPONENT$4) != null;
}
}
/**
* Sets the "component" attribute
*/
public void setComponent(java.lang.String component)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(COMPONENT$4);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(COMPONENT$4);
}
target.setStringValue(component);
}
}
/**
* Sets (as xml) the "component" attribute
*/
public void xsetComponent(net.sf.buildbox.changes.bean.ItemBean.Component component)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Component target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Component)get_store().find_attribute_user(COMPONENT$4);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ItemBean.Component)get_store().add_attribute_user(COMPONENT$4);
}
target.set(component);
}
}
/**
* Unsets the "component" attribute
*/
public void unsetComponent()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(COMPONENT$4);
}
}
/**
* Gets the "timestamp" attribute
*/
public java.util.Calendar getTimestamp()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TIMESTAMP$6);
if (target == null)
{
return null;
}
return target.getCalendarValue();
}
}
/**
* Gets (as xml) the "timestamp" attribute
*/
public org.apache.xmlbeans.XmlDateTime xgetTimestamp()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlDateTime target = null;
target = (org.apache.xmlbeans.XmlDateTime)get_store().find_attribute_user(TIMESTAMP$6);
return target;
}
}
/**
* True if has "timestamp" attribute
*/
public boolean isSetTimestamp()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(TIMESTAMP$6) != null;
}
}
/**
* Sets the "timestamp" attribute
*/
public void setTimestamp(java.util.Calendar timestamp)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TIMESTAMP$6);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(TIMESTAMP$6);
}
target.setCalendarValue(timestamp);
}
}
/**
* Sets (as xml) the "timestamp" attribute
*/
public void xsetTimestamp(org.apache.xmlbeans.XmlDateTime timestamp)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlDateTime target = null;
target = (org.apache.xmlbeans.XmlDateTime)get_store().find_attribute_user(TIMESTAMP$6);
if (target == null)
{
target = (org.apache.xmlbeans.XmlDateTime)get_store().add_attribute_user(TIMESTAMP$6);
}
target.set(timestamp);
}
}
/**
* Unsets the "timestamp" attribute
*/
public void unsetTimestamp()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(TIMESTAMP$6);
}
}
/**
* Gets the "reporter" attribute
*/
public java.lang.String getReporter()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REPORTER$8);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "reporter" attribute
*/
public net.sf.buildbox.changes.bean.ItemBean.Reporter xgetReporter()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Reporter target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Reporter)get_store().find_attribute_user(REPORTER$8);
return target;
}
}
/**
* True if has "reporter" attribute
*/
public boolean isSetReporter()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(REPORTER$8) != null;
}
}
/**
* Sets the "reporter" attribute
*/
public void setReporter(java.lang.String reporter)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REPORTER$8);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(REPORTER$8);
}
target.setStringValue(reporter);
}
}
/**
* Sets (as xml) the "reporter" attribute
*/
public void xsetReporter(net.sf.buildbox.changes.bean.ItemBean.Reporter reporter)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Reporter target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Reporter)get_store().find_attribute_user(REPORTER$8);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ItemBean.Reporter)get_store().add_attribute_user(REPORTER$8);
}
target.set(reporter);
}
}
/**
* Unsets the "reporter" attribute
*/
public void unsetReporter()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(REPORTER$8);
}
}
/**
* Gets the "resolver" attribute
*/
public java.lang.String getResolver()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(RESOLVER$10);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "resolver" attribute
*/
public net.sf.buildbox.changes.bean.ItemBean.Resolver xgetResolver()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Resolver target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Resolver)get_store().find_attribute_user(RESOLVER$10);
return target;
}
}
/**
* True if has "resolver" attribute
*/
public boolean isSetResolver()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(RESOLVER$10) != null;
}
}
/**
* Sets the "resolver" attribute
*/
public void setResolver(java.lang.String resolver)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(RESOLVER$10);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(RESOLVER$10);
}
target.setStringValue(resolver);
}
}
/**
* Sets (as xml) the "resolver" attribute
*/
public void xsetResolver(net.sf.buildbox.changes.bean.ItemBean.Resolver resolver)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Resolver target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Resolver)get_store().find_attribute_user(RESOLVER$10);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ItemBean.Resolver)get_store().add_attribute_user(RESOLVER$10);
}
target.set(resolver);
}
}
/**
* Unsets the "resolver" attribute
*/
public void unsetResolver()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(RESOLVER$10);
}
}
/**
* Gets the "priority" attribute
*/
public net.sf.buildbox.changes.bean.ItemBean.Priority.Enum getPriority()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PRIORITY$12);
if (target == null)
{
return null;
}
return (net.sf.buildbox.changes.bean.ItemBean.Priority.Enum)target.getEnumValue();
}
}
/**
* Gets (as xml) the "priority" attribute
*/
public net.sf.buildbox.changes.bean.ItemBean.Priority xgetPriority()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Priority target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Priority)get_store().find_attribute_user(PRIORITY$12);
return target;
}
}
/**
* True if has "priority" attribute
*/
public boolean isSetPriority()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(PRIORITY$12) != null;
}
}
/**
* Sets the "priority" attribute
*/
public void setPriority(net.sf.buildbox.changes.bean.ItemBean.Priority.Enum priority)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PRIORITY$12);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(PRIORITY$12);
}
target.setEnumValue(priority);
}
}
/**
* Sets (as xml) the "priority" attribute
*/
public void xsetPriority(net.sf.buildbox.changes.bean.ItemBean.Priority priority)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.ItemBean.Priority target = null;
target = (net.sf.buildbox.changes.bean.ItemBean.Priority)get_store().find_attribute_user(PRIORITY$12);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.ItemBean.Priority)get_store().add_attribute_user(PRIORITY$12);
}
target.set(priority);
}
}
/**
* Unsets the "priority" attribute
*/
public void unsetPriority()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(PRIORITY$12);
}
}
/**
* An XML action(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.ItemBean$Action.
*/
public static class ActionImpl extends org.apache.xmlbeans.impl.values.JavaStringEnumerationHolderEx implements net.sf.buildbox.changes.bean.ItemBean.Action
{
private static final long serialVersionUID = 1L;
public ActionImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected ActionImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML issue(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.ItemBean$Issue.
*/
public static class IssueImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.ItemBean.Issue
{
private static final long serialVersionUID = 1L;
public IssueImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected IssueImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML component(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.ItemBean$Component.
*/
public static class ComponentImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.ItemBean.Component
{
private static final long serialVersionUID = 1L;
public ComponentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected ComponentImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML reporter(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.ItemBean$Reporter.
*/
public static class ReporterImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.ItemBean.Reporter
{
private static final long serialVersionUID = 1L;
public ReporterImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected ReporterImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML resolver(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.ItemBean$Resolver.
*/
public static class ResolverImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.ItemBean.Resolver
{
private static final long serialVersionUID = 1L;
public ResolverImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected ResolverImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML priority(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.ItemBean$Priority.
*/
public static class PriorityImpl extends org.apache.xmlbeans.impl.values.JavaStringEnumerationHolderEx implements net.sf.buildbox.changes.bean.ItemBean.Priority
{
private static final long serialVersionUID = 1L;
public PriorityImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected PriorityImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy