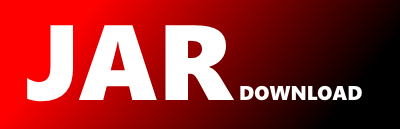
net.sf.buildbox.changes.bean.impl.UpgradeProcedureBeanImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of releasator Show documentation
Show all versions of releasator Show documentation
Commandline utility for creating reproducible releases. Minimal parametrization, isolated sandbox for releases. Currently built on top of maven-release-plugin.
The newest version!
/*
* XML Type: UpgradeProcedure
* Namespace: http://buildbox.sf.net/changes/2.0
* Java type: net.sf.buildbox.changes.bean.UpgradeProcedureBean
*
* Automatically generated - do not modify.
*/
package net.sf.buildbox.changes.bean.impl;
/**
* An XML UpgradeProcedure(@http://buildbox.sf.net/changes/2.0).
*
* This is a complex type.
*/
public class UpgradeProcedureBeanImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.sf.buildbox.changes.bean.UpgradeProcedureBean
{
private static final long serialVersionUID = 1L;
public UpgradeProcedureBeanImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName FROM$0 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "from");
private static final javax.xml.namespace.QName STEP$2 =
new javax.xml.namespace.QName("http://buildbox.sf.net/changes/2.0", "step");
/**
* Gets the "from" element
*/
public net.sf.buildbox.changes.bean.UpgradeProcedureBean.From getFrom()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.From target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From)get_store().find_element_user(FROM$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "from" element
*/
public void setFrom(net.sf.buildbox.changes.bean.UpgradeProcedureBean.From from)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.From target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From)get_store().find_element_user(FROM$0, 0);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From)get_store().add_element_user(FROM$0);
}
target.set(from);
}
}
/**
* Appends and returns a new empty "from" element
*/
public net.sf.buildbox.changes.bean.UpgradeProcedureBean.From addNewFrom()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.From target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From)get_store().add_element_user(FROM$0);
return target;
}
}
/**
* Gets array of all "step" elements
*/
public net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step[] getStepArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(STEP$2, targetList);
net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step[] result = new net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "step" element
*/
public net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step getStepArray(int i)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step)get_store().find_element_user(STEP$2, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "step" element
*/
public int sizeOfStepArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(STEP$2);
}
}
/**
* Sets array of all "step" element
*/
public void setStepArray(net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step[] stepArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(stepArray, STEP$2);
}
}
/**
* Sets ith "step" element
*/
public void setStepArray(int i, net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step step)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step)get_store().find_element_user(STEP$2, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(step);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "step" element
*/
public net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step insertNewStep(int i)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step)get_store().insert_element_user(STEP$2, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "step" element
*/
public net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step addNewStep()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step)get_store().add_element_user(STEP$2);
return target;
}
}
/**
* Removes the ith "step" element
*/
public void removeStep(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(STEP$2, i);
}
}
/**
* An XML from(@http://buildbox.sf.net/changes/2.0).
*
* This is a complex type.
*/
public static class FromImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.sf.buildbox.changes.bean.UpgradeProcedureBean.From
{
private static final long serialVersionUID = 1L;
public FromImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName GROUPID$0 =
new javax.xml.namespace.QName("", "groupId");
private static final javax.xml.namespace.QName ARTIFACTID$2 =
new javax.xml.namespace.QName("", "artifactId");
private static final javax.xml.namespace.QName VERSION$4 =
new javax.xml.namespace.QName("", "version");
/**
* Gets the "groupId" attribute
*/
public java.lang.String getGroupId()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(GROUPID$0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "groupId" attribute
*/
public net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.GroupId xgetGroupId()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.GroupId target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.GroupId)get_store().find_attribute_user(GROUPID$0);
return target;
}
}
/**
* Sets the "groupId" attribute
*/
public void setGroupId(java.lang.String groupId)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(GROUPID$0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(GROUPID$0);
}
target.setStringValue(groupId);
}
}
/**
* Sets (as xml) the "groupId" attribute
*/
public void xsetGroupId(net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.GroupId groupId)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.GroupId target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.GroupId)get_store().find_attribute_user(GROUPID$0);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.GroupId)get_store().add_attribute_user(GROUPID$0);
}
target.set(groupId);
}
}
/**
* Gets the "artifactId" attribute
*/
public java.lang.String getArtifactId()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ARTIFACTID$2);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "artifactId" attribute
*/
public net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.ArtifactId xgetArtifactId()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.ArtifactId target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.ArtifactId)get_store().find_attribute_user(ARTIFACTID$2);
return target;
}
}
/**
* Sets the "artifactId" attribute
*/
public void setArtifactId(java.lang.String artifactId)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ARTIFACTID$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(ARTIFACTID$2);
}
target.setStringValue(artifactId);
}
}
/**
* Sets (as xml) the "artifactId" attribute
*/
public void xsetArtifactId(net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.ArtifactId artifactId)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.ArtifactId target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.ArtifactId)get_store().find_attribute_user(ARTIFACTID$2);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.ArtifactId)get_store().add_attribute_user(ARTIFACTID$2);
}
target.set(artifactId);
}
}
/**
* Gets the "version" attribute
*/
public java.lang.String getVersion()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(VERSION$4);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "version" attribute
*/
public net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.Version xgetVersion()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.Version target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.Version)get_store().find_attribute_user(VERSION$4);
return target;
}
}
/**
* Sets the "version" attribute
*/
public void setVersion(java.lang.String version)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(VERSION$4);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(VERSION$4);
}
target.setStringValue(version);
}
}
/**
* Sets (as xml) the "version" attribute
*/
public void xsetVersion(net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.Version version)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.Version target = null;
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.Version)get_store().find_attribute_user(VERSION$4);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.Version)get_store().add_attribute_user(VERSION$4);
}
target.set(version);
}
}
/**
* An XML groupId(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.UpgradeProcedureBean$From$GroupId.
*/
public static class GroupIdImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.GroupId
{
private static final long serialVersionUID = 1L;
public GroupIdImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected GroupIdImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML artifactId(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.UpgradeProcedureBean$From$ArtifactId.
*/
public static class ArtifactIdImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.ArtifactId
{
private static final long serialVersionUID = 1L;
public ArtifactIdImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected ArtifactIdImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML version(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.UpgradeProcedureBean$From$Version.
*/
public static class VersionImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.UpgradeProcedureBean.From.Version
{
private static final long serialVersionUID = 1L;
public VersionImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected VersionImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
}
/**
* An XML step(@http://buildbox.sf.net/changes/2.0).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.UpgradeProcedureBean$Step.
*/
public static class StepImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.UpgradeProcedureBean.Step
{
private static final long serialVersionUID = 1L;
public StepImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, true);
}
protected StepImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
private static final javax.xml.namespace.QName TYPE$0 =
new javax.xml.namespace.QName("", "type");
/**
* Gets the "type" attribute
*/
public java.lang.String getType()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TYPE$0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "type" attribute
*/
public org.apache.xmlbeans.XmlString xgetType()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_attribute_user(TYPE$0);
return target;
}
}
/**
* True if has "type" attribute
*/
public boolean isSetType()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(TYPE$0) != null;
}
}
/**
* Sets the "type" attribute
*/
public void setType(java.lang.String type)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TYPE$0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(TYPE$0);
}
target.setStringValue(type);
}
}
/**
* Sets (as xml) the "type" attribute
*/
public void xsetType(org.apache.xmlbeans.XmlString type)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_attribute_user(TYPE$0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlString)get_store().add_attribute_user(TYPE$0);
}
target.set(type);
}
}
/**
* Unsets the "type" attribute
*/
public void unsetType()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(TYPE$0);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy