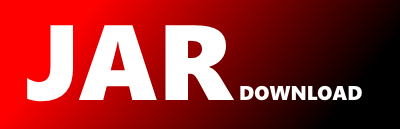
net.sf.buildbox.changes.bean.impl.VcsInfoBeanImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of releasator Show documentation
Show all versions of releasator Show documentation
Commandline utility for creating reproducible releases. Minimal parametrization, isolated sandbox for releases. Currently built on top of maven-release-plugin.
The newest version!
/*
* XML Type: VcsInfo
* Namespace: http://buildbox.sf.net/changes/2.0
* Java type: net.sf.buildbox.changes.bean.VcsInfoBean
*
* Automatically generated - do not modify.
*/
package net.sf.buildbox.changes.bean.impl;
/**
* An XML VcsInfo(@http://buildbox.sf.net/changes/2.0).
*
* This is a complex type.
*/
public class VcsInfoBeanImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.sf.buildbox.changes.bean.VcsInfoBean
{
private static final long serialVersionUID = 1L;
public VcsInfoBeanImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName ID$0 =
new javax.xml.namespace.QName("", "id");
private static final javax.xml.namespace.QName LOCATION$2 =
new javax.xml.namespace.QName("", "location");
private static final javax.xml.namespace.QName TAG$4 =
new javax.xml.namespace.QName("", "tag");
private static final javax.xml.namespace.QName TYPE$6 =
new javax.xml.namespace.QName("", "type");
private static final javax.xml.namespace.QName REVISION$8 =
new javax.xml.namespace.QName("", "revision");
/**
* Gets the "id" attribute
*/
public java.lang.String getId()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ID$0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "id" attribute
*/
public net.sf.buildbox.changes.bean.VcsInfoBean.Id xgetId()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Id target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Id)get_store().find_attribute_user(ID$0);
return target;
}
}
/**
* Sets the "id" attribute
*/
public void setId(java.lang.String id)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ID$0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(ID$0);
}
target.setStringValue(id);
}
}
/**
* Sets (as xml) the "id" attribute
*/
public void xsetId(net.sf.buildbox.changes.bean.VcsInfoBean.Id id)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Id target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Id)get_store().find_attribute_user(ID$0);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Id)get_store().add_attribute_user(ID$0);
}
target.set(id);
}
}
/**
* Gets the "location" attribute
*/
public java.lang.String getLocation()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(LOCATION$2);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "location" attribute
*/
public net.sf.buildbox.changes.bean.VcsInfoBean.Location xgetLocation()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Location target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Location)get_store().find_attribute_user(LOCATION$2);
return target;
}
}
/**
* Sets the "location" attribute
*/
public void setLocation(java.lang.String location)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(LOCATION$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(LOCATION$2);
}
target.setStringValue(location);
}
}
/**
* Sets (as xml) the "location" attribute
*/
public void xsetLocation(net.sf.buildbox.changes.bean.VcsInfoBean.Location location)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Location target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Location)get_store().find_attribute_user(LOCATION$2);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Location)get_store().add_attribute_user(LOCATION$2);
}
target.set(location);
}
}
/**
* Gets the "tag" attribute
*/
public java.lang.String getTag()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TAG$4);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "tag" attribute
*/
public net.sf.buildbox.changes.bean.VcsInfoBean.Tag xgetTag()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Tag target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Tag)get_store().find_attribute_user(TAG$4);
return target;
}
}
/**
* Sets the "tag" attribute
*/
public void setTag(java.lang.String tag)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TAG$4);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(TAG$4);
}
target.setStringValue(tag);
}
}
/**
* Sets (as xml) the "tag" attribute
*/
public void xsetTag(net.sf.buildbox.changes.bean.VcsInfoBean.Tag tag)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Tag target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Tag)get_store().find_attribute_user(TAG$4);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Tag)get_store().add_attribute_user(TAG$4);
}
target.set(tag);
}
}
/**
* Gets the "type" attribute
*/
public java.lang.String getType()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TYPE$6);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "type" attribute
*/
public net.sf.buildbox.changes.bean.VcsInfoBean.Type xgetType()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Type target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Type)get_store().find_attribute_user(TYPE$6);
return target;
}
}
/**
* True if has "type" attribute
*/
public boolean isSetType()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(TYPE$6) != null;
}
}
/**
* Sets the "type" attribute
*/
public void setType(java.lang.String type)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TYPE$6);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(TYPE$6);
}
target.setStringValue(type);
}
}
/**
* Sets (as xml) the "type" attribute
*/
public void xsetType(net.sf.buildbox.changes.bean.VcsInfoBean.Type type)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Type target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Type)get_store().find_attribute_user(TYPE$6);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Type)get_store().add_attribute_user(TYPE$6);
}
target.set(type);
}
}
/**
* Unsets the "type" attribute
*/
public void unsetType()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(TYPE$6);
}
}
/**
* Gets the "revision" attribute
*/
public java.lang.String getRevision()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REVISION$8);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "revision" attribute
*/
public net.sf.buildbox.changes.bean.VcsInfoBean.Revision xgetRevision()
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Revision target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Revision)get_store().find_attribute_user(REVISION$8);
return target;
}
}
/**
* True if has "revision" attribute
*/
public boolean isSetRevision()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(REVISION$8) != null;
}
}
/**
* Sets the "revision" attribute
*/
public void setRevision(java.lang.String revision)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REVISION$8);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(REVISION$8);
}
target.setStringValue(revision);
}
}
/**
* Sets (as xml) the "revision" attribute
*/
public void xsetRevision(net.sf.buildbox.changes.bean.VcsInfoBean.Revision revision)
{
synchronized (monitor())
{
check_orphaned();
net.sf.buildbox.changes.bean.VcsInfoBean.Revision target = null;
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Revision)get_store().find_attribute_user(REVISION$8);
if (target == null)
{
target = (net.sf.buildbox.changes.bean.VcsInfoBean.Revision)get_store().add_attribute_user(REVISION$8);
}
target.set(revision);
}
}
/**
* Unsets the "revision" attribute
*/
public void unsetRevision()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(REVISION$8);
}
}
/**
* An XML id(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.VcsInfoBean$Id.
*/
public static class IdImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.VcsInfoBean.Id
{
private static final long serialVersionUID = 1L;
public IdImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected IdImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML location(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.VcsInfoBean$Location.
*/
public static class LocationImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.VcsInfoBean.Location
{
private static final long serialVersionUID = 1L;
public LocationImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected LocationImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML tag(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.VcsInfoBean$Tag.
*/
public static class TagImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.VcsInfoBean.Tag
{
private static final long serialVersionUID = 1L;
public TagImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected TagImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML type(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.VcsInfoBean$Type.
*/
public static class TypeImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.VcsInfoBean.Type
{
private static final long serialVersionUID = 1L;
public TypeImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected TypeImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
/**
* An XML revision(@).
*
* This is an atomic type that is a restriction of net.sf.buildbox.changes.bean.VcsInfoBean$Revision.
*/
public static class RevisionImpl extends org.apache.xmlbeans.impl.values.JavaStringHolderEx implements net.sf.buildbox.changes.bean.VcsInfoBean.Revision
{
private static final long serialVersionUID = 1L;
public RevisionImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType, false);
}
protected RevisionImpl(org.apache.xmlbeans.SchemaType sType, boolean b)
{
super(sType, b);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy