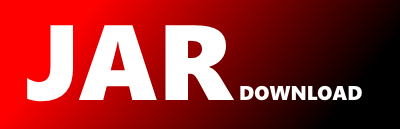
cz.vutbr.web.css.NodeData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jstyleparser Show documentation
Show all versions of jstyleparser Show documentation
jStyleParser is a CSS parser written in Java. It has its own application interface that is designed to allow an efficient CSS processing in Java and mapping the values to the Java data types. It parses CSS 2.1 style sheets into structures that can be efficiently assigned to DOM elements. It is intended be the primary CSS parser for the CSSBox library. While handling errors, it is user agent conforming according to the CSS specification.
The newest version!
package cz.vutbr.web.css;
import java.util.Collection;
import cz.vutbr.web.css.CSSProperty.ValueType;
/**
* Wrap of CSS properties defined for element. Enumeration values follows this
* syntax:
*
* -
* UPPERCASE terminal symbols, direct values present in stylesheet, such
* as background-color: transparent;
-
* lowercase non-terminal symbols, just information that concrete value
* is stored somewhere else
*
* @author kapy
* @author burgetr
*/
public interface NodeData {
/**
* Returns property of given name and supposed type. Inherited properties
* are included.
*
* @param
* Type of property returned. Let compiler infer returning type
* from left part of statement, otherwise return just CSSProperty
* @param name
* Name of property
* @return Value of property of type T or null
*/
public T getProperty(String name);
/**
* Returns property of given name and supposed type. Inherited properties
* can be avoided
*
* @param
* Type of property returned. Let compiler infer returning type
* from left part of statement, otherwise return just CSSProperty
* @param name
* Name of property
* @param includeInherited
* Whether to include inherited properties or not
* @return Value of property of type T or null
*/
public T getProperty(String name, boolean includeInherited);
/**
* Returns n-th property of given name and supposed type. Inherited properties
* are included. This is used for properties of the {@link ValueType#LIST} type
* which can have multiple values.
*
* @param
* Type of property returned. Let compiler infer returning type
* from left part of statement, otherwise return just CSSProperty
* @param name
* Name of property
* @param index
* Property value index
* @return Value of property of type T or null
*/
public T getProperty(String name, int index);
/**
* Returns n-th property of given name and supposed type. Inherited properties
* are included. This is used for properties of the {@link ValueType#LIST} type
* which can have multiple values.
*
* @param
* Type of property returned. Let compiler infer returning type
* from left part of statement, otherwise return just CSSProperty
* @param name
* Name of property
* @param index
* Property value index
* @param includeInherited
* Whether to include inherited properties or not
* @return Value of property of type T or null
*/
public T getProperty(String name, int index, boolean includeInherited);
/**
* Returns the property of the given name after applying the defaulting processes.
*
* @param name
* Property name
* @return The specified value of the property of the type T or {@code null} if the
* value is not available and no default value is applicable.
*/
public T getSpecifiedProperty(String name);
/**
* Returns the cascaded value of property of given name.
* Inherited values can be avoided.
*
* @param name
* Name of property
* @param includeInherited
* Whether to include inherited properties or not
* @return Value of property or null
*/
public Term> getValue(String name, boolean includeInherited);
/**
* Returns the cascaded value of property of given name and supposed type.
* Inherited values can be avoided.
*
* @param
* Type of value returned
* @param clazz
* Class of type
* @param name
* Name of property
* @return Value of property of type T or null
*/
public > T getValue(Class clazz, String name);
/**
* Returns the cascaded value of property of given name and supposed type.
* Inherited values can be avoided.
*
* @param
* Type of value returned
* @param clazz
* Class of type
* @param name
* Name of property
* @param includeInherited
* Whether to include inherited properties or not
* @return Value of property of type T or null
*/
public > T getValue(Class clazz, String name,
boolean includeInherited);
/**
* Returns the cascaded value of a list property of given name.
* Inherited values can be avoided.
*
* @param name
* Name of property
* @param index
* Property value index
* @param includeInherited
* Whether to include inherited properties or not
* @return Value of property or null
*/
public Term> getValue(String name, int index, boolean includeInherited);
/**
* Returns the cascaded value of a list property of given name and supposed type.
* Inherited values can be avoided.
*
* @param
* Type of value returned
* @param clazz
* Class of type
* @param name
* Name of property
* @param index
* Property value index
* @return Value of property of type T or null
*/
public > T getValue(Class clazz, String name, int index);
/**
* Returns the cascaded value of a list property of given name and supposed type.
* Inherited values can be avoided.
*
* @param
* Type of value returned
* @param clazz
* Class of type
* @param name
* Name of property
* @param index
* Property value index
* @param includeInherited
* Whether to include inherited properties or not
* @return Value of property of type T or null
*/
public > T getValue(Class clazz, String name, int index,
boolean includeInherited);
/**
* Returns the specified value of a property which corresponds to the
* cascaded value (obtained by {@link NodeData#getValue(String, boolean)}) with
* applying the defaulting processes.
* @param name the property name
* @return the property value or {@code null} when the property value is not defined
* and no default value is available.
*/
public Term> getSpecifiedValue(String name);
/**
* Returns the specified value of a property which corresponds to the
* cascaded value (obtained by {@link NodeData#getValue(String, boolean)}) with
* applying the defaulting processes.
*
* @param clazz the expected class of the result
* @param name the property name
* @return the property value or {@code null} when the property value is not defined
* and no default value is available.
*/
public > T getSpecifiedValue(Class clazz, String name);
/**
* Returns a string representation of the property value.
*
* @param name
* Property name
* @param includeInherited
* Whether to include inherited properties or not
* @return The string representation of the assigned property value
* or {@code null} when the property is not defined.
*/
public String getAsString(String name, boolean includeInherited);
/**
* For list properties, obtains the number of property values in the nested list.
*
* @param name property name
* @param includeInherited Whether to include inherited properties or not
* @return the number of property values defined in the list
*/
public int getListSize(String name, boolean includeInherited);
/**
* Accepts values from parent as its own. null
parent is
* allowed, than instance is returned unchanged.
*
* @param parent
* Source of inheritance
* @return Modified instance
* @throws ClassCastException When parent implementation class is not the same
*/
public NodeData inheritFrom(NodeData parent) throws ClassCastException;
/**
* Replaces all {@code inherit}, {@code initial} and {@code unset} CSS properties
* with the inherited values or default values of user agent.
*
* @return Modified property
*/
public NodeData concretize();
/**
* Adds data stored in declaration into current instance
*
* @param d
* Declaration to be added
* @return Modified instance
*
*/
public NodeData push(Declaration d);
/**
* Returns the names of all the that are available in the current node.
*
* @return the name of the properties.
*/
public Collection getPropertyNames();
/**
* Obtains the source declaration used for the given property. Inherited properties are included.
* @param name The property name.
* @return the source declaration
*/
public Declaration getSourceDeclaration(String name);
/**
* Obtains the source declaration used for the given property.
* @param name The property name.
* @param includeInherited whether to include the inherited properties.
* @return the source declaration
*/
public Declaration getSourceDeclaration(String name, boolean includeInherited);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy