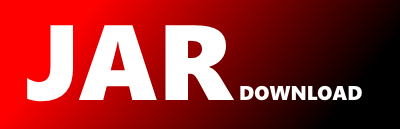
cz.vutbr.web.csskit.antlr4.CSSLexer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jstyleparser Show documentation
Show all versions of jstyleparser Show documentation
jStyleParser is a CSS parser written in Java. It has its own application interface that is designed to allow an efficient CSS processing in Java and mapping the values to the Java data types. It parses CSS 2.1 style sheets into structures that can be efficiently assigned to DOM elements. It is intended be the primary CSS parser for the CSSBox library. While handling errors, it is user agent conforming according to the CSS specification.
The newest version!
// Generated from cz/vutbr/web/csskit/antlr4/CSSLexer.g4 by ANTLR 4.13.2
package cz.vutbr.web.csskit.antlr4;
import org.antlr.v4.runtime.Lexer;
import org.antlr.v4.runtime.CharStream;
import org.antlr.v4.runtime.Token;
import org.antlr.v4.runtime.TokenStream;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.misc.*;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast", "CheckReturnValue", "this-escape"})
public class CSSLexer extends Lexer {
static { RuntimeMetaData.checkVersion("4.13.2", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
STYLESHEET=1, INLINESTYLE=2, ATBLOCK=3, CURLYBLOCK=4, PARENBLOCK=5, BRACKETBLOCK=6,
RULE=7, SELECTOR=8, ELEMENT=9, PSEUDOCLASS=10, PSEUDOELEM=11, ADJACENT=12,
PRECEDING=13, CHILD=14, DESCENDANT=15, ATTRIBUTE=16, SET=17, DECLARATION=18,
VALUE=19, MEDIA_QUERY=20, INVALID_STRING=21, INVALID_SELECTOR=22, INVALID_SELPART=23,
INVALID_DECLARATION=24, INVALID_STATEMENT=25, INVALID_ATSTATEMENT=26,
INVALID_IMPORT=27, INVALID_DIRECTIVE=28, IMPORTANT=29, IDENT=30, CHARSET=31,
IMPORT=32, KEYFRAMES=33, MEDIA=34, PAGE=35, MARGIN_AREA=36, VIEWPORT=37,
FONTFACE=38, ATKEYWORD=39, CLASSKEYWORD=40, STRING=41, UNCLOSED_STRING=42,
HASH=43, INDEX=44, NUMBER=45, PERCENTAGE=46, DIMENSION=47, URI=48, UNCLOSED_URI=49,
UNIRANGE=50, CDO=51, CDC=52, SEMICOLON=53, COLON=54, COMMA=55, QUESTION=56,
PERCENT=57, EQUALS=58, SLASH=59, GREATER=60, LESS=61, LCURLY=62, RCURLY=63,
APOS=64, QUOT=65, LPAREN=66, RPAREN=67, LBRACKET=68, RBRACKET=69, EXCLAMATION=70,
TILDE=71, MINUS=72, PLUS=73, ASTERISK=74, POUND=75, AMPERSAND=76, HAT=77,
S=78, COMMENT=79, SL_COMMENT=80, EXPRESSION=81, FUNCTION=82, INCLUDES=83,
DASHMATCH=84, STARTSWITH=85, ENDSWITH=86, CONTAINS=87, CTRL=88, INVALID_TOKEN=89,
STRING_MACR=90, UNCLOSED_STRING_MACR=91, STRING_CHAR=92;
public static String[] channelNames = {
"DEFAULT_TOKEN_CHANNEL", "HIDDEN"
};
public static String[] modeNames = {
"DEFAULT_MODE"
};
private static String[] makeRuleNames() {
return new String[] {
"IMPORTANT", "IDENT", "CHARSET", "IMPORT", "KEYFRAMES", "MEDIA", "PAGE",
"MARGIN_AREA", "VIEWPORT", "FONTFACE", "ATKEYWORD", "CLASSKEYWORD", "STRING",
"UNCLOSED_STRING", "HASH", "INDEX", "NUMBER", "PERCENTAGE", "DIMENSION",
"URI", "UNCLOSED_URI", "UNIRANGE", "CDO", "CDC", "SEMICOLON", "COLON",
"COMMA", "QUESTION", "PERCENT", "EQUALS", "SLASH", "GREATER", "LESS",
"LCURLY", "RCURLY", "APOS", "QUOT", "LPAREN", "RPAREN", "LBRACKET", "RBRACKET",
"EXCLAMATION", "TILDE", "MINUS", "PLUS", "ASTERISK", "POUND", "AMPERSAND",
"HAT", "S", "COMMENT", "SL_COMMENT", "EXPRESSION", "FUNCTION", "INCLUDES",
"DASHMATCH", "STARTSWITH", "ENDSWITH", "CONTAINS", "CTRL", "INVALID_TOKEN",
"IDENT_MACR", "NAME_MACR", "NAME_START", "NON_ASCII", "ESCAPE_CHAR",
"NAME_CHAR", "INTEGER_MACR", "NUMBER_MACR", "STRING_MACR", "UNCLOSED_STRING_MACR",
"STRING_CHAR", "URI_MACR", "URI_CHAR", "NL_CHAR", "W_MACR", "W_CHAR",
"CTRL_CHAR"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, "'important'", null, null, "'@import'",
"'@keyframes'", "'@media'", "'@page'", null, "'@viewport'", "'@font-face'",
null, null, null, null, null, null, null, null, null, null, null, null,
"''", "';'", "':'", "','", "'?'", "'%'", "'='", "'/'", "'>'",
"'<'", "'{'", "'}'", "'''", "'\"'", "'('", "')'", "'['", "']'", "'!'",
"'~'", "'-'", "'+'", "'*'", "'#'", "'&'", "'^'", null, null, null, "'expression('",
null, "'~='", "'|='", "'^='", "'$='", "'*='"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "STYLESHEET", "INLINESTYLE", "ATBLOCK", "CURLYBLOCK", "PARENBLOCK",
"BRACKETBLOCK", "RULE", "SELECTOR", "ELEMENT", "PSEUDOCLASS", "PSEUDOELEM",
"ADJACENT", "PRECEDING", "CHILD", "DESCENDANT", "ATTRIBUTE", "SET", "DECLARATION",
"VALUE", "MEDIA_QUERY", "INVALID_STRING", "INVALID_SELECTOR", "INVALID_SELPART",
"INVALID_DECLARATION", "INVALID_STATEMENT", "INVALID_ATSTATEMENT", "INVALID_IMPORT",
"INVALID_DIRECTIVE", "IMPORTANT", "IDENT", "CHARSET", "IMPORT", "KEYFRAMES",
"MEDIA", "PAGE", "MARGIN_AREA", "VIEWPORT", "FONTFACE", "ATKEYWORD",
"CLASSKEYWORD", "STRING", "UNCLOSED_STRING", "HASH", "INDEX", "NUMBER",
"PERCENTAGE", "DIMENSION", "URI", "UNCLOSED_URI", "UNIRANGE", "CDO",
"CDC", "SEMICOLON", "COLON", "COMMA", "QUESTION", "PERCENT", "EQUALS",
"SLASH", "GREATER", "LESS", "LCURLY", "RCURLY", "APOS", "QUOT", "LPAREN",
"RPAREN", "LBRACKET", "RBRACKET", "EXCLAMATION", "TILDE", "MINUS", "PLUS",
"ASTERISK", "POUND", "AMPERSAND", "HAT", "S", "COMMENT", "SL_COMMENT",
"EXPRESSION", "FUNCTION", "INCLUDES", "DASHMATCH", "STARTSWITH", "ENDSWITH",
"CONTAINS", "CTRL", "INVALID_TOKEN", "STRING_MACR", "UNCLOSED_STRING_MACR",
"STRING_CHAR"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
// CSSLexer.g4 members start
private org.slf4j.Logger log;
// number of already processed tokens (for checking the beginning of the style sheet)
protected int tokencnt = 0;
// 'charset changed' flag for preventing multiple @charset rules
protected boolean charsetChanged = false;
// current lexer state
protected cz.vutbr.web.csskit.antlr4.CSSLexerState ls;
// last UNCLOSED_* token
protected cz.vutbr.web.csskit.antlr4.CSSToken lastUnclosed;
/**
* token facctory for generating custom tokens (CSSToken)
*/
protected cz.vutbr.web.csskit.antlr4.CSSTokenFactory tf;
protected cz.vutbr.web.csskit.antlr4.CSSTokenRecovery tr;
/**
* This function must be called to initialize lexer's state.
* Because we can't change directly generated constructors.
*/
public void init() {
this.log = org.slf4j.LoggerFactory.getLogger(getClass());
this.ls = new cz.vutbr.web.csskit.antlr4.CSSLexerState();
//initialize CSSTokenFactory
this.tf = new cz.vutbr.web.csskit.antlr4.CSSTokenFactory(_tokenFactorySourcePair, this, ls, getClass());
//initialize CSSTokenRecovery
this.tr = new cz.vutbr.web.csskit.antlr4.CSSTokenRecovery(this, _input, ls, log);
}
@Override
public void reset() {
throw new UnsupportedOperationException();
}
/**
* Overrides inputStream to avoid setting input
* stream after construction
*/
@Override
public void setInputStream(IntStream input) {
throw new UnsupportedOperationException();
}
/**
* Overrides next token to match includes and to
* recover from EOF
*/
@Override
public Token nextToken(){
Token token = tr.nextToken();
//count non-empty tokens for eventual checking of the style sheet start
if (token.getType() == S) {
tokencnt++;
}
//save last UNCLOSED_URI for later checking the EOF
if (token.getType() == UNCLOSED_URI) {
lastUnclosed = (CSSToken) token;
lastUnclosed.setValid(false);
}
//in case of EOF, convert the unclosed token to closed one
else if (token.getType() == Token.EOF) {
if (lastUnclosed != null) {
lastUnclosed.setValid(true);
log.debug("Validating UNCLOSED_URI by EOF");
}
}
//reset unclosed uri
else if (!tr.isAtEof())
lastUnclosed = null;
// Skip first token after switching on another input.
if(((CommonToken)token).getStartIndex() < 0)
token = nextToken();
return token;
}
/**
* Adds contextual information about nesting into token.
*/
@Override
public Token emit() {
Token t = tf.make();
emit(t);
return t;
}
/**
* Does special token recovery for some cases
*/
@Override
public void recover(RecognitionException re) {
log.debug("recover" + re.toString());
if (!tr.recover())
super.recover(re);
}
// CSSLexer.g4 members end
public CSSLexer(CharStream input) {
super(input);
_interp = new LexerATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
@Override
public String getGrammarFileName() { return "CSSLexer.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public String[] getChannelNames() { return channelNames; }
@Override
public String[] getModeNames() { return modeNames; }
@Override
public ATN getATN() { return _ATN; }
@Override
public void action(RuleContext _localctx, int ruleIndex, int actionIndex) {
switch (ruleIndex) {
case 2:
CHARSET_action((RuleContext)_localctx, actionIndex);
break;
case 12:
STRING_action((RuleContext)_localctx, actionIndex);
break;
case 24:
SEMICOLON_action((RuleContext)_localctx, actionIndex);
break;
case 33:
LCURLY_action((RuleContext)_localctx, actionIndex);
break;
case 34:
RCURLY_action((RuleContext)_localctx, actionIndex);
break;
case 35:
APOS_action((RuleContext)_localctx, actionIndex);
break;
case 36:
QUOT_action((RuleContext)_localctx, actionIndex);
break;
case 37:
LPAREN_action((RuleContext)_localctx, actionIndex);
break;
case 38:
RPAREN_action((RuleContext)_localctx, actionIndex);
break;
case 39:
LBRACKET_action((RuleContext)_localctx, actionIndex);
break;
case 40:
RBRACKET_action((RuleContext)_localctx, actionIndex);
break;
case 53:
FUNCTION_action((RuleContext)_localctx, actionIndex);
break;
case 69:
STRING_MACR_action((RuleContext)_localctx, actionIndex);
break;
case 70:
UNCLOSED_STRING_MACR_action((RuleContext)_localctx, actionIndex);
break;
}
}
private void CHARSET_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 0:
tr.expecting(CHARSET);
break;
case 1:
// we have to trim manually
String enc = cz.vutbr.web.csskit.antlr4.CSSToken.extractCHARSET(getText());
if (tokencnt <= 1 && !charsetChanged) //we are at the beginning of the style sheet
{
try {
log.warn("Changing charset to {}", enc);
charsetChanged = true;
((cz.vutbr.web.csskit.antlr4.CSSInputStream) _input).setEncoding(enc);
}
catch(java.nio.charset.IllegalCharsetNameException icne) {
log.warn("Could not change to unsupported charset!", icne);
throw new RuntimeException(new cz.vutbr.web.css.CSSException("Unsupported charset: " + enc));
}
catch (java.io.IOException e) {
log.warn("Could not change to unsupported charset!", e);
}
}
else{
log.warn("Ignoring @charset rule not at the beginning of the style sheet");
}
tr.end();
break;
}
}
private void STRING_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 2:
tr.expecting(STRING);
break;
case 3:
tr.end();
break;
}
}
private void SEMICOLON_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 4:
ls.sqNest = 0;
break;
}
}
private void LCURLY_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 5:
ls.curlyNest++;
break;
}
}
private void RCURLY_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 6:
if(ls.curlyNest>0) ls.curlyNest--; ls.sqNest = 0;
break;
}
}
private void APOS_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 7:
ls.aposOpen=!ls.aposOpen;
break;
}
}
private void QUOT_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 8:
ls.quotOpen=!ls.quotOpen;
break;
}
}
private void LPAREN_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 9:
ls.parenNest++;
break;
}
}
private void RPAREN_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 10:
if(ls.parenNest>0) ls.parenNest--;
break;
}
}
private void LBRACKET_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 11:
ls.sqNest++;
break;
}
}
private void RBRACKET_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 12:
if(ls.sqNest>0) ls.sqNest--;
break;
}
}
private void FUNCTION_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 13:
ls.parenNest++;
break;
}
}
private void STRING_MACR_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 14:
ls.aposOpen=false;
break;
case 15:
ls.quotOpen=false;
break;
}
}
private void UNCLOSED_STRING_MACR_action(RuleContext _localctx, int actionIndex) {
switch (actionIndex) {
case 16:
ls.aposOpen=false;
break;
case 17:
ls.quotOpen=false;
break;
}
}
public static final String _serializedATN =
"\u0004\u0000\\\u0349\u0006\uffff\uffff\u0002\u0000\u0007\u0000\u0002\u0001"+
"\u0007\u0001\u0002\u0002\u0007\u0002\u0002\u0003\u0007\u0003\u0002\u0004"+
"\u0007\u0004\u0002\u0005\u0007\u0005\u0002\u0006\u0007\u0006\u0002\u0007"+
"\u0007\u0007\u0002\b\u0007\b\u0002\t\u0007\t\u0002\n\u0007\n\u0002\u000b"+
"\u0007\u000b\u0002\f\u0007\f\u0002\r\u0007\r\u0002\u000e\u0007\u000e\u0002"+
"\u000f\u0007\u000f\u0002\u0010\u0007\u0010\u0002\u0011\u0007\u0011\u0002"+
"\u0012\u0007\u0012\u0002\u0013\u0007\u0013\u0002\u0014\u0007\u0014\u0002"+
"\u0015\u0007\u0015\u0002\u0016\u0007\u0016\u0002\u0017\u0007\u0017\u0002"+
"\u0018\u0007\u0018\u0002\u0019\u0007\u0019\u0002\u001a\u0007\u001a\u0002"+
"\u001b\u0007\u001b\u0002\u001c\u0007\u001c\u0002\u001d\u0007\u001d\u0002"+
"\u001e\u0007\u001e\u0002\u001f\u0007\u001f\u0002 \u0007 \u0002!\u0007"+
"!\u0002\"\u0007\"\u0002#\u0007#\u0002$\u0007$\u0002%\u0007%\u0002&\u0007"+
"&\u0002\'\u0007\'\u0002(\u0007(\u0002)\u0007)\u0002*\u0007*\u0002+\u0007"+
"+\u0002,\u0007,\u0002-\u0007-\u0002.\u0007.\u0002/\u0007/\u00020\u0007"+
"0\u00021\u00071\u00022\u00072\u00023\u00073\u00024\u00074\u00025\u0007"+
"5\u00026\u00076\u00027\u00077\u00028\u00078\u00029\u00079\u0002:\u0007"+
":\u0002;\u0007;\u0002<\u0007<\u0002=\u0007=\u0002>\u0007>\u0002?\u0007"+
"?\u0002@\u0007@\u0002A\u0007A\u0002B\u0007B\u0002C\u0007C\u0002D\u0007"+
"D\u0002E\u0007E\u0002F\u0007F\u0002G\u0007G\u0002H\u0007H\u0002I\u0007"+
"I\u0002J\u0007J\u0002K\u0007K\u0002L\u0007L\u0002M\u0007M\u0001\u0000"+
"\u0001\u0000\u0001\u0000\u0001\u0000\u0001\u0000\u0001\u0000\u0001\u0000"+
"\u0001\u0000\u0001\u0000\u0001\u0000\u0001\u0001\u0001\u0001\u0001\u0002"+
"\u0001\u0002\u0001\u0002\u0001\u0002\u0001\u0002\u0001\u0002\u0001\u0002"+
"\u0001\u0002\u0001\u0002\u0001\u0002\u0001\u0002\u0005\u0002\u00b5\b\u0002"+
"\n\u0002\f\u0002\u00b8\t\u0002\u0001\u0002\u0003\u0002\u00bb\b\u0002\u0001"+
"\u0002\u0005\u0002\u00be\b\u0002\n\u0002\f\u0002\u00c1\t\u0002\u0001\u0002"+
"\u0001\u0002\u0001\u0002\u0001\u0003\u0001\u0003\u0001\u0003\u0001\u0003"+
"\u0001\u0003\u0001\u0003\u0001\u0003\u0001\u0003\u0001\u0004\u0001\u0004"+
"\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0004"+
"\u0001\u0004\u0001\u0004\u0001\u0004\u0001\u0005\u0001\u0005\u0001\u0005"+
"\u0001\u0005\u0001\u0005\u0001\u0005\u0001\u0005\u0001\u0006\u0001\u0006"+
"\u0001\u0006\u0001\u0006\u0001\u0006\u0001\u0006\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007"+
"\u0001\u0007\u0001\u0007\u0001\u0007\u0001\u0007\u0003\u0007\u01b8\b\u0007"+
"\u0001\b\u0001\b\u0001\b\u0001\b\u0001\b\u0001\b\u0001\b\u0001\b\u0001"+
"\b\u0001\b\u0001\t\u0001\t\u0001\t\u0001\t\u0001\t\u0001\t\u0001\t\u0001"+
"\t\u0001\t\u0001\t\u0001\t\u0001\n\u0001\n\u0003\n\u01d1\b\n\u0001\n\u0001"+
"\n\u0001\u000b\u0001\u000b\u0001\u000b\u0001\f\u0001\f\u0001\f\u0001\f"+
"\u0001\r\u0001\r\u0001\u000e\u0001\u000e\u0001\u000e\u0001\u000f\u0003"+
"\u000f\u01e2\b\u000f\u0001\u000f\u0001\u000f\u0005\u000f\u01e6\b\u000f"+
"\n\u000f\f\u000f\u01e9\t\u000f\u0001\u000f\u0001\u000f\u0003\u000f\u01ed"+
"\b\u000f\u0001\u000f\u0005\u000f\u01f0\b\u000f\n\u000f\f\u000f\u01f3\t"+
"\u000f\u0001\u000f\u0001\u000f\u0003\u000f\u01f7\b\u000f\u0001\u0010\u0001"+
"\u0010\u0001\u0011\u0001\u0011\u0001\u0011\u0001\u0012\u0001\u0012\u0001"+
"\u0012\u0001\u0013\u0001\u0013\u0001\u0013\u0001\u0013\u0001\u0013\u0001"+
"\u0013\u0001\u0013\u0001\u0013\u0003\u0013\u0209\b\u0013\u0001\u0013\u0001"+
"\u0013\u0001\u0013\u0001\u0014\u0001\u0014\u0001\u0014\u0001\u0014\u0001"+
"\u0014\u0001\u0014\u0001\u0014\u0001\u0014\u0001\u0014\u0003\u0014\u0217"+
"\b\u0014\u0001\u0014\u0001\u0014\u0001\u0015\u0001\u0015\u0001\u0015\u0001"+
"\u0015\u0001\u0015\u0001\u0015\u0001\u0015\u0001\u0015\u0001\u0015\u0003"+
"\u0015\u0224\b\u0015\u0001\u0015\u0001\u0015\u0001\u0015\u0001\u0015\u0001"+
"\u0015\u0001\u0015\u0001\u0015\u0003\u0015\u022d\b\u0015\u0003\u0015\u022f"+
"\b\u0015\u0001\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0001\u0016\u0001"+
"\u0017\u0001\u0017\u0001\u0017\u0001\u0017\u0001\u0018\u0001\u0018\u0001"+
"\u0018\u0001\u0019\u0001\u0019\u0001\u001a\u0001\u001a\u0001\u001b\u0001"+
"\u001b\u0001\u001c\u0001\u001c\u0001\u001d\u0001\u001d\u0001\u001e\u0001"+
"\u001e\u0001\u001f\u0001\u001f\u0001 \u0001 \u0001!\u0001!\u0001!\u0001"+
"\"\u0001\"\u0001\"\u0001#\u0001#\u0001#\u0001$\u0001$\u0001$\u0001%\u0001"+
"%\u0001%\u0001&\u0001&\u0001&\u0001\'\u0001\'\u0001\'\u0001(\u0001(\u0001"+
"(\u0001)\u0001)\u0001*\u0001*\u0001+\u0001+\u0001,\u0001,\u0001-\u0001"+
"-\u0001.\u0001.\u0001/\u0001/\u00010\u00010\u00011\u00041\u0276\b1\u000b"+
"1\f1\u0277\u00012\u00012\u00012\u00012\u00052\u027e\b2\n2\f2\u0281\t2"+
"\u00012\u00012\u00012\u00032\u0286\b2\u00012\u00012\u00013\u00013\u0001"+
"3\u00013\u00053\u028e\b3\n3\f3\u0291\t3\u00013\u00013\u00013\u00013\u0001"+
"4\u00014\u00014\u00014\u00014\u00014\u00014\u00014\u00014\u00014\u0001"+
"4\u00014\u00015\u00015\u00015\u00015\u00016\u00016\u00016\u00017\u0001"+
"7\u00017\u00018\u00018\u00018\u00019\u00019\u00019\u0001:\u0001:\u0001"+
":\u0001;\u0004;\u02b7\b;\u000b;\f;\u02b8\u0001<\u0001<\u0001=\u0001=\u0005"+
"=\u02bf\b=\n=\f=\u02c2\t=\u0001>\u0004>\u02c5\b>\u000b>\f>\u02c6\u0001"+
"?\u0001?\u0001?\u0003?\u02cc\b?\u0001@\u0001@\u0001A\u0001A\u0004A\u02d2"+
"\bA\u000bA\fA\u02d3\u0001A\u0003A\u02d7\bA\u0001A\u0003A\u02da\bA\u0001"+
"B\u0001B\u0001B\u0003B\u02df\bB\u0001C\u0004C\u02e2\bC\u000bC\fC\u02e3"+
"\u0001D\u0004D\u02e7\bD\u000bD\fD\u02e8\u0001D\u0005D\u02ec\bD\nD\fD\u02ef"+
"\tD\u0001D\u0001D\u0004D\u02f3\bD\u000bD\fD\u02f4\u0003D\u02f7\bD\u0001"+
"E\u0001E\u0001E\u0001E\u0001E\u0005E\u02fe\bE\nE\fE\u0301\tE\u0001E\u0001"+
"E\u0001E\u0001E\u0001E\u0001E\u0001E\u0005E\u030a\bE\nE\fE\u030d\tE\u0001"+
"E\u0001E\u0003E\u0311\bE\u0001F\u0001F\u0001F\u0001F\u0001F\u0005F\u0318"+
"\bF\nF\fF\u031b\tF\u0001F\u0001F\u0001F\u0001F\u0001F\u0005F\u0322\bF"+
"\nF\fF\u0325\tF\u0003F\u0327\bF\u0001G\u0001G\u0001G\u0001G\u0003G\u032d"+
"\bG\u0001H\u0005H\u0330\bH\nH\fH\u0333\tH\u0001I\u0001I\u0001I\u0003I"+
"\u0338\bI\u0001J\u0001J\u0001J\u0001J\u0003J\u033e\bJ\u0001K\u0005K\u0341"+
"\bK\nK\fK\u0344\tK\u0001L\u0001L\u0001M\u0001M\u0002\u027f\u028f\u0000"+
"N\u0001\u001d\u0003\u001e\u0005\u001f\u0007 \t!\u000b\"\r#\u000f$\u0011"+
"%\u0013&\u0015\'\u0017(\u0019)\u001b*\u001d+\u001f,!-#.%/\'0)1+2-3/41"+
"536577899;:=;?E?G@IAKBMCODQESFUGWHYI[J]K_LaMcNeOgPiQkRmSoTqUsVuW"+
"wXyY{\u0000}\u0000\u007f\u0000\u0081\u0000\u0083\u0000\u0085\u0000\u0087"+
"\u0000\u0089\u0000\u008bZ\u008d[\u008f\\\u0091\u0000\u0093\u0000\u0095"+
"\u0000\u0097\u0000\u0099\u0000\u009b\u0000\u0001\u0000\f\u0002\u0000N"+
"Nnn\u0004\u000009??AFaf\u0003\u000009AFaf\u0002\u0000\n\n\r\r\u0003\u0000"+
"AZ__az\u0002\u0000\u0080\u8000\ud7ff\u8000\ue000\u8000\ufffd\u0006\u0000"+
" /:@G`g~\u0080\u8000\ud7ff\u8000\ue000\u8000\ufffd\u0005\u0000--09AZ_"+
"_az\u0002\u0000 ()\u0004\u0000\t\t!!#&*~\u0002\u0000\t\r \u0002\u0000"+
"\u0000\b\u000e\u001f\u037d\u0000\u0001\u0001\u0000\u0000\u0000\u0000\u0003"+
"\u0001\u0000\u0000\u0000\u0000\u0005\u0001\u0000\u0000\u0000\u0000\u0007"+
"\u0001\u0000\u0000\u0000\u0000\t\u0001\u0000\u0000\u0000\u0000\u000b\u0001"+
"\u0000\u0000\u0000\u0000\r\u0001\u0000\u0000\u0000\u0000\u000f\u0001\u0000"+
"\u0000\u0000\u0000\u0011\u0001\u0000\u0000\u0000\u0000\u0013\u0001\u0000"+
"\u0000\u0000\u0000\u0015\u0001\u0000\u0000\u0000\u0000\u0017\u0001\u0000"+
"\u0000\u0000\u0000\u0019\u0001\u0000\u0000\u0000\u0000\u001b\u0001\u0000"+
"\u0000\u0000\u0000\u001d\u0001\u0000\u0000\u0000\u0000\u001f\u0001\u0000"+
"\u0000\u0000\u0000!\u0001\u0000\u0000\u0000\u0000#\u0001\u0000\u0000\u0000"+
"\u0000%\u0001\u0000\u0000\u0000\u0000\'\u0001\u0000\u0000\u0000\u0000"+
")\u0001\u0000\u0000\u0000\u0000+\u0001\u0000\u0000\u0000\u0000-\u0001"+
"\u0000\u0000\u0000\u0000/\u0001\u0000\u0000\u0000\u00001\u0001\u0000\u0000"+
"\u0000\u00003\u0001\u0000\u0000\u0000\u00005\u0001\u0000\u0000\u0000\u0000"+
"7\u0001\u0000\u0000\u0000\u00009\u0001\u0000\u0000\u0000\u0000;\u0001"+
"\u0000\u0000\u0000\u0000=\u0001\u0000\u0000\u0000\u0000?\u0001\u0000\u0000"+
"\u0000\u0000A\u0001\u0000\u0000\u0000\u0000C\u0001\u0000\u0000\u0000\u0000"+
"E\u0001\u0000\u0000\u0000\u0000G\u0001\u0000\u0000\u0000\u0000I\u0001"+
"\u0000\u0000\u0000\u0000K\u0001\u0000\u0000\u0000\u0000M\u0001\u0000\u0000"+
"\u0000\u0000O\u0001\u0000\u0000\u0000\u0000Q\u0001\u0000\u0000\u0000\u0000"+
"S\u0001\u0000\u0000\u0000\u0000U\u0001\u0000\u0000\u0000\u0000W\u0001"+
"\u0000\u0000\u0000\u0000Y\u0001\u0000\u0000\u0000\u0000[\u0001\u0000\u0000"+
"\u0000\u0000]\u0001\u0000\u0000\u0000\u0000_\u0001\u0000\u0000\u0000\u0000"+
"a\u0001\u0000\u0000\u0000\u0000c\u0001\u0000\u0000\u0000\u0000e\u0001"+
"\u0000\u0000\u0000\u0000g\u0001\u0000\u0000\u0000\u0000i\u0001\u0000\u0000"+
"\u0000\u0000k\u0001\u0000\u0000\u0000\u0000m\u0001\u0000\u0000\u0000\u0000"+
"o\u0001\u0000\u0000\u0000\u0000q\u0001\u0000\u0000\u0000\u0000s\u0001"+
"\u0000\u0000\u0000\u0000u\u0001\u0000\u0000\u0000\u0000w\u0001\u0000\u0000"+
"\u0000\u0000y\u0001\u0000\u0000\u0000\u0000\u008b\u0001\u0000\u0000\u0000"+
"\u0000\u008d\u0001\u0000\u0000\u0000\u0000\u008f\u0001\u0000\u0000\u0000"+
"\u0001\u009d\u0001\u0000\u0000\u0000\u0003\u00a7\u0001\u0000\u0000\u0000"+
"\u0005\u00a9\u0001\u0000\u0000\u0000\u0007\u00c5\u0001\u0000\u0000\u0000"+
"\t\u00cd\u0001\u0000\u0000\u0000\u000b\u00d8\u0001\u0000\u0000\u0000\r"+
"\u00df\u0001\u0000\u0000\u0000\u000f\u01b7\u0001\u0000\u0000\u0000\u0011"+
"\u01b9\u0001\u0000\u0000\u0000\u0013\u01c3\u0001\u0000\u0000\u0000\u0015"+
"\u01ce\u0001\u0000\u0000\u0000\u0017\u01d4\u0001\u0000\u0000\u0000\u0019"+
"\u01d7\u0001\u0000\u0000\u0000\u001b\u01db\u0001\u0000\u0000\u0000\u001d"+
"\u01dd\u0001\u0000\u0000\u0000\u001f\u01e1\u0001\u0000\u0000\u0000!\u01f8"+
"\u0001\u0000\u0000\u0000#\u01fa\u0001\u0000\u0000\u0000%\u01fd\u0001\u0000"+
"\u0000\u0000\'\u0200\u0001\u0000\u0000\u0000)\u020d\u0001\u0000\u0000"+
"\u0000+\u021a\u0001\u0000\u0000\u0000-\u0230\u0001\u0000\u0000\u0000/"+
"\u0235\u0001\u0000\u0000\u00001\u0239\u0001\u0000\u0000\u00003\u023c\u0001"+
"\u0000\u0000\u00005\u023e\u0001\u0000\u0000\u00007\u0240\u0001\u0000\u0000"+
"\u00009\u0242\u0001\u0000\u0000\u0000;\u0244\u0001\u0000\u0000\u0000="+
"\u0246\u0001\u0000\u0000\u0000?\u0248\u0001\u0000\u0000\u0000A\u024a\u0001"+
"\u0000\u0000\u0000C\u024c\u0001\u0000\u0000\u0000E\u024f\u0001\u0000\u0000"+
"\u0000G\u0252\u0001\u0000\u0000\u0000I\u0255\u0001\u0000\u0000\u0000K"+
"\u0258\u0001\u0000\u0000\u0000M\u025b\u0001\u0000\u0000\u0000O\u025e\u0001"+
"\u0000\u0000\u0000Q\u0261\u0001\u0000\u0000\u0000S\u0264\u0001\u0000\u0000"+
"\u0000U\u0266\u0001\u0000\u0000\u0000W\u0268\u0001\u0000\u0000\u0000Y"+
"\u026a\u0001\u0000\u0000\u0000[\u026c\u0001\u0000\u0000\u0000]\u026e\u0001"+
"\u0000\u0000\u0000_\u0270\u0001\u0000\u0000\u0000a\u0272\u0001\u0000\u0000"+
"\u0000c\u0275\u0001\u0000\u0000\u0000e\u0279\u0001\u0000\u0000\u0000g"+
"\u0289\u0001\u0000\u0000\u0000i\u0296\u0001\u0000\u0000\u0000k\u02a2\u0001"+
"\u0000\u0000\u0000m\u02a6\u0001\u0000\u0000\u0000o\u02a9\u0001\u0000\u0000"+
"\u0000q\u02ac\u0001\u0000\u0000\u0000s\u02af\u0001\u0000\u0000\u0000u"+
"\u02b2\u0001\u0000\u0000\u0000w\u02b6\u0001\u0000\u0000\u0000y\u02ba\u0001"+
"\u0000\u0000\u0000{\u02bc\u0001\u0000\u0000\u0000}\u02c4\u0001\u0000\u0000"+
"\u0000\u007f\u02cb\u0001\u0000\u0000\u0000\u0081\u02cd\u0001\u0000\u0000"+
"\u0000\u0083\u02cf\u0001\u0000\u0000\u0000\u0085\u02de\u0001\u0000\u0000"+
"\u0000\u0087\u02e1\u0001\u0000\u0000\u0000\u0089\u02f6\u0001\u0000\u0000"+
"\u0000\u008b\u0310\u0001\u0000\u0000\u0000\u008d\u0326\u0001\u0000\u0000"+
"\u0000\u008f\u032c\u0001\u0000\u0000\u0000\u0091\u0331\u0001\u0000\u0000"+
"\u0000\u0093\u0337\u0001\u0000\u0000\u0000\u0095\u033d\u0001\u0000\u0000"+
"\u0000\u0097\u0342\u0001\u0000\u0000\u0000\u0099\u0345\u0001\u0000\u0000"+
"\u0000\u009b\u0347\u0001\u0000\u0000\u0000\u009d\u009e\u0005i\u0000\u0000"+
"\u009e\u009f\u0005m\u0000\u0000\u009f\u00a0\u0005p\u0000\u0000\u00a0\u00a1"+
"\u0005o\u0000\u0000\u00a1\u00a2\u0005r\u0000\u0000\u00a2\u00a3\u0005t"+
"\u0000\u0000\u00a3\u00a4\u0005a\u0000\u0000\u00a4\u00a5\u0005n\u0000\u0000"+
"\u00a5\u00a6\u0005t\u0000\u0000\u00a6\u0002\u0001\u0000\u0000\u0000\u00a7"+
"\u00a8\u0003{=\u0000\u00a8\u0004\u0001\u0000\u0000\u0000\u00a9\u00aa\u0006"+
"\u0002\u0000\u0000\u00aa\u00ab\u0005@\u0000\u0000\u00ab\u00ac\u0005c\u0000"+
"\u0000\u00ac\u00ad\u0005h\u0000\u0000\u00ad\u00ae\u0005a\u0000\u0000\u00ae"+
"\u00af\u0005r\u0000\u0000\u00af\u00b0\u0005s\u0000\u0000\u00b0\u00b1\u0005"+
"e\u0000\u0000\u00b1\u00b2\u0005t\u0000\u0000\u00b2\u00b6\u0001\u0000\u0000"+
"\u0000\u00b3\u00b5\u0003c1\u0000\u00b4\u00b3\u0001\u0000\u0000\u0000\u00b5"+
"\u00b8\u0001\u0000\u0000\u0000\u00b6\u00b4\u0001\u0000\u0000\u0000\u00b6"+
"\u00b7\u0001\u0000\u0000\u0000\u00b7\u00ba\u0001\u0000\u0000\u0000\u00b8"+
"\u00b6\u0001\u0000\u0000\u0000\u00b9\u00bb\u0003\u008bE\u0000\u00ba\u00b9"+
"\u0001\u0000\u0000\u0000\u00ba\u00bb\u0001\u0000\u0000\u0000\u00bb\u00bf"+
"\u0001\u0000\u0000\u0000\u00bc\u00be\u0003c1\u0000\u00bd\u00bc\u0001\u0000"+
"\u0000\u0000\u00be\u00c1\u0001\u0000\u0000\u0000\u00bf\u00bd\u0001\u0000"+
"\u0000\u0000\u00bf\u00c0\u0001\u0000\u0000\u0000\u00c0\u00c2\u0001\u0000"+
"\u0000\u0000\u00c1\u00bf\u0001\u0000\u0000\u0000\u00c2\u00c3\u00031\u0018"+
"\u0000\u00c3\u00c4\u0006\u0002\u0001\u0000\u00c4\u0006\u0001\u0000\u0000"+
"\u0000\u00c5\u00c6\u0005@\u0000\u0000\u00c6\u00c7\u0005i\u0000\u0000\u00c7"+
"\u00c8\u0005m\u0000\u0000\u00c8\u00c9\u0005p\u0000\u0000\u00c9\u00ca\u0005"+
"o\u0000\u0000\u00ca\u00cb\u0005r\u0000\u0000\u00cb\u00cc\u0005t\u0000"+
"\u0000\u00cc\b\u0001\u0000\u0000\u0000\u00cd\u00ce\u0005@\u0000\u0000"+
"\u00ce\u00cf\u0005k\u0000\u0000\u00cf\u00d0\u0005e\u0000\u0000\u00d0\u00d1"+
"\u0005y\u0000\u0000\u00d1\u00d2\u0005f\u0000\u0000\u00d2\u00d3\u0005r"+
"\u0000\u0000\u00d3\u00d4\u0005a\u0000\u0000\u00d4\u00d5\u0005m\u0000\u0000"+
"\u00d5\u00d6\u0005e\u0000\u0000\u00d6\u00d7\u0005s\u0000\u0000\u00d7\n"+
"\u0001\u0000\u0000\u0000\u00d8\u00d9\u0005@\u0000\u0000\u00d9\u00da\u0005"+
"m\u0000\u0000\u00da\u00db\u0005e\u0000\u0000\u00db\u00dc\u0005d\u0000"+
"\u0000\u00dc\u00dd\u0005i\u0000\u0000\u00dd\u00de\u0005a\u0000\u0000\u00de"+
"\f\u0001\u0000\u0000\u0000\u00df\u00e0\u0005@\u0000\u0000\u00e0\u00e1"+
"\u0005p\u0000\u0000\u00e1\u00e2\u0005a\u0000\u0000\u00e2\u00e3\u0005g"+
"\u0000\u0000\u00e3\u00e4\u0005e\u0000\u0000\u00e4\u000e\u0001\u0000\u0000"+
"\u0000\u00e5\u00e6\u0005@\u0000\u0000\u00e6\u00e7\u0005t\u0000\u0000\u00e7"+
"\u00e8\u0005o\u0000\u0000\u00e8\u00e9\u0005p\u0000\u0000\u00e9\u00ea\u0005"+
"-\u0000\u0000\u00ea\u00eb\u0005l\u0000\u0000\u00eb\u00ec\u0005e\u0000"+
"\u0000\u00ec\u00ed\u0005f\u0000\u0000\u00ed\u00ee\u0005t\u0000\u0000\u00ee"+
"\u00ef\u0005-\u0000\u0000\u00ef\u00f0\u0005c\u0000\u0000\u00f0\u00f1\u0005"+
"o\u0000\u0000\u00f1\u00f2\u0005r\u0000\u0000\u00f2\u00f3\u0005n\u0000"+
"\u0000\u00f3\u00f4\u0005e\u0000\u0000\u00f4\u01b8\u0005r\u0000\u0000\u00f5"+
"\u00f6\u0005@\u0000\u0000\u00f6\u00f7\u0005t\u0000\u0000\u00f7\u00f8\u0005"+
"o\u0000\u0000\u00f8\u00f9\u0005p\u0000\u0000\u00f9\u00fa\u0005-\u0000"+
"\u0000\u00fa\u00fb\u0005l\u0000\u0000\u00fb\u00fc\u0005e\u0000\u0000\u00fc"+
"\u00fd\u0005f\u0000\u0000\u00fd\u01b8\u0005t\u0000\u0000\u00fe\u00ff\u0005"+
"@\u0000\u0000\u00ff\u0100\u0005t\u0000\u0000\u0100\u0101\u0005o\u0000"+
"\u0000\u0101\u0102\u0005p\u0000\u0000\u0102\u0103\u0005-\u0000\u0000\u0103"+
"\u0104\u0005c\u0000\u0000\u0104\u0105\u0005e\u0000\u0000\u0105\u0106\u0005"+
"n\u0000\u0000\u0106\u0107\u0005t\u0000\u0000\u0107\u0108\u0005e\u0000"+
"\u0000\u0108\u01b8\u0005r\u0000\u0000\u0109\u010a\u0005@\u0000\u0000\u010a"+
"\u010b\u0005t\u0000\u0000\u010b\u010c\u0005o\u0000\u0000\u010c\u010d\u0005"+
"p\u0000\u0000\u010d\u010e\u0005-\u0000\u0000\u010e\u010f\u0005r\u0000"+
"\u0000\u010f\u0110\u0005i\u0000\u0000\u0110\u0111\u0005g\u0000\u0000\u0111"+
"\u0112\u0005h\u0000\u0000\u0112\u01b8\u0005t\u0000\u0000\u0113\u0114\u0005"+
"@\u0000\u0000\u0114\u0115\u0005t\u0000\u0000\u0115\u0116\u0005o\u0000"+
"\u0000\u0116\u0117\u0005p\u0000\u0000\u0117\u0118\u0005-\u0000\u0000\u0118"+
"\u0119\u0005r\u0000\u0000\u0119\u011a\u0005i\u0000\u0000\u011a\u011b\u0005"+
"g\u0000\u0000\u011b\u011c\u0005h\u0000\u0000\u011c\u011d\u0005t\u0000"+
"\u0000\u011d\u011e\u0005-\u0000\u0000\u011e\u011f\u0005c\u0000\u0000\u011f"+
"\u0120\u0005o\u0000\u0000\u0120\u0121\u0005r\u0000\u0000\u0121\u0122\u0005"+
"n\u0000\u0000\u0122\u0123\u0005e\u0000\u0000\u0123\u01b8\u0005r\u0000"+
"\u0000\u0124\u0125\u0005@\u0000\u0000\u0125\u0126\u0005b\u0000\u0000\u0126"+
"\u0127\u0005o\u0000\u0000\u0127\u0128\u0005t\u0000\u0000\u0128\u0129\u0005"+
"t\u0000\u0000\u0129\u012a\u0005o\u0000\u0000\u012a\u012b\u0005m\u0000"+
"\u0000\u012b\u012c\u0005-\u0000\u0000\u012c\u012d\u0005l\u0000\u0000\u012d"+
"\u012e\u0005e\u0000\u0000\u012e\u012f\u0005f\u0000\u0000\u012f\u0130\u0005"+
"t\u0000\u0000\u0130\u0131\u0005-\u0000\u0000\u0131\u0132\u0005c\u0000"+
"\u0000\u0132\u0133\u0005o\u0000\u0000\u0133\u0134\u0005r\u0000\u0000\u0134"+
"\u0135\u0005n\u0000\u0000\u0135\u0136\u0005e\u0000\u0000\u0136\u01b8\u0005"+
"r\u0000\u0000\u0137\u0138\u0005@\u0000\u0000\u0138\u0139\u0005b\u0000"+
"\u0000\u0139\u013a\u0005o\u0000\u0000\u013a\u013b\u0005t\u0000\u0000\u013b"+
"\u013c\u0005t\u0000\u0000\u013c\u013d\u0005o\u0000\u0000\u013d\u013e\u0005"+
"m\u0000\u0000\u013e\u013f\u0005-\u0000\u0000\u013f\u0140\u0005l\u0000"+
"\u0000\u0140\u0141\u0005e\u0000\u0000\u0141\u0142\u0005f\u0000\u0000\u0142"+
"\u01b8\u0005t\u0000\u0000\u0143\u0144\u0005@\u0000\u0000\u0144\u0145\u0005"+
"b\u0000\u0000\u0145\u0146\u0005o\u0000\u0000\u0146\u0147\u0005t\u0000"+
"\u0000\u0147\u0148\u0005t\u0000\u0000\u0148\u0149\u0005o\u0000\u0000\u0149"+
"\u014a\u0005m\u0000\u0000\u014a\u014b\u0005-\u0000\u0000\u014b\u014c\u0005"+
"c\u0000\u0000\u014c\u014d\u0005e\u0000\u0000\u014d\u014e\u0005n\u0000"+
"\u0000\u014e\u014f\u0005t\u0000\u0000\u014f\u0150\u0005e\u0000\u0000\u0150"+
"\u01b8\u0005r\u0000\u0000\u0151\u0152\u0005@\u0000\u0000\u0152\u0153\u0005"+
"b\u0000\u0000\u0153\u0154\u0005o\u0000\u0000\u0154\u0155\u0005t\u0000"+
"\u0000\u0155\u0156\u0005t\u0000\u0000\u0156\u0157\u0005o\u0000\u0000\u0157"+
"\u0158\u0005m\u0000\u0000\u0158\u0159\u0005-\u0000\u0000\u0159\u015a\u0005"+
"r\u0000\u0000\u015a\u015b\u0005i\u0000\u0000\u015b\u015c\u0005g\u0000"+
"\u0000\u015c\u015d\u0005h\u0000\u0000\u015d\u01b8\u0005t\u0000\u0000\u015e"+
"\u015f\u0005@\u0000\u0000\u015f\u0160\u0005b\u0000\u0000\u0160\u0161\u0005"+
"o\u0000\u0000\u0161\u0162\u0005t\u0000\u0000\u0162\u0163\u0005t\u0000"+
"\u0000\u0163\u0164\u0005o\u0000\u0000\u0164\u0165\u0005m\u0000\u0000\u0165"+
"\u0166\u0005-\u0000\u0000\u0166\u0167\u0005r\u0000\u0000\u0167\u0168\u0005"+
"i\u0000\u0000\u0168\u0169\u0005g\u0000\u0000\u0169\u016a\u0005h\u0000"+
"\u0000\u016a\u016b\u0005t\u0000\u0000\u016b\u016c\u0005-\u0000\u0000\u016c"+
"\u016d\u0005c\u0000\u0000\u016d\u016e\u0005o\u0000\u0000\u016e\u016f\u0005"+
"r\u0000\u0000\u016f\u0170\u0005n\u0000\u0000\u0170\u0171\u0005e\u0000"+
"\u0000\u0171\u01b8\u0005r\u0000\u0000\u0172\u0173\u0005@\u0000\u0000\u0173"+
"\u0174\u0005l\u0000\u0000\u0174\u0175\u0005e\u0000\u0000\u0175\u0176\u0005"+
"f\u0000\u0000\u0176\u0177\u0005t\u0000\u0000\u0177\u0178\u0005-\u0000"+
"\u0000\u0178\u0179\u0005t\u0000\u0000\u0179\u017a\u0005o\u0000\u0000\u017a"+
"\u01b8\u0005p\u0000\u0000\u017b\u017c\u0005@\u0000\u0000\u017c\u017d\u0005"+
"l\u0000\u0000\u017d\u017e\u0005e\u0000\u0000\u017e\u017f\u0005f\u0000"+
"\u0000\u017f\u0180\u0005t\u0000\u0000\u0180\u0181\u0005-\u0000\u0000\u0181"+
"\u0182\u0005m\u0000\u0000\u0182\u0183\u0005i\u0000\u0000\u0183\u0184\u0005"+
"d\u0000\u0000\u0184\u0185\u0005d\u0000\u0000\u0185\u0186\u0005l\u0000"+
"\u0000\u0186\u01b8\u0005e\u0000\u0000\u0187\u0188\u0005@\u0000\u0000\u0188"+
"\u0189\u0005l\u0000\u0000\u0189\u018a\u0005e\u0000\u0000\u018a\u018b\u0005"+
"f\u0000\u0000\u018b\u018c\u0005t\u0000\u0000\u018c\u018d\u0005-\u0000"+
"\u0000\u018d\u018e\u0005b\u0000\u0000\u018e\u018f\u0005o\u0000\u0000\u018f"+
"\u0190\u0005t\u0000\u0000\u0190\u0191\u0005t\u0000\u0000\u0191\u0192\u0005"+
"o\u0000\u0000\u0192\u01b8\u0005m\u0000\u0000\u0193\u0194\u0005@\u0000"+
"\u0000\u0194\u0195\u0005r\u0000\u0000\u0195\u0196\u0005i\u0000\u0000\u0196"+
"\u0197\u0005g\u0000\u0000\u0197\u0198\u0005h\u0000\u0000\u0198\u0199\u0005"+
"t\u0000\u0000\u0199\u019a\u0005-\u0000\u0000\u019a\u019b\u0005t\u0000"+
"\u0000\u019b\u019c\u0005o\u0000\u0000\u019c\u01b8\u0005p\u0000\u0000\u019d"+
"\u019e\u0005@\u0000\u0000\u019e\u019f\u0005r\u0000\u0000\u019f\u01a0\u0005"+
"i\u0000\u0000\u01a0\u01a1\u0005g\u0000\u0000\u01a1\u01a2\u0005h\u0000"+
"\u0000\u01a2\u01a3\u0005t\u0000\u0000\u01a3\u01a4\u0005-\u0000\u0000\u01a4"+
"\u01a5\u0005m\u0000\u0000\u01a5\u01a6\u0005i\u0000\u0000\u01a6\u01a7\u0005"+
"d\u0000\u0000\u01a7\u01a8\u0005d\u0000\u0000\u01a8\u01a9\u0005l\u0000"+
"\u0000\u01a9\u01b8\u0005e\u0000\u0000\u01aa\u01ab\u0005@\u0000\u0000\u01ab"+
"\u01ac\u0005r\u0000\u0000\u01ac\u01ad\u0005i\u0000\u0000\u01ad\u01ae\u0005"+
"g\u0000\u0000\u01ae\u01af\u0005h\u0000\u0000\u01af\u01b0\u0005t\u0000"+
"\u0000\u01b0\u01b1\u0005-\u0000\u0000\u01b1\u01b2\u0005b\u0000\u0000\u01b2"+
"\u01b3\u0005o\u0000\u0000\u01b3\u01b4\u0005t\u0000\u0000\u01b4\u01b5\u0005"+
"t\u0000\u0000\u01b5\u01b6\u0005o\u0000\u0000\u01b6\u01b8\u0005m\u0000"+
"\u0000\u01b7\u00e5\u0001\u0000\u0000\u0000\u01b7\u00f5\u0001\u0000\u0000"+
"\u0000\u01b7\u00fe\u0001\u0000\u0000\u0000\u01b7\u0109\u0001\u0000\u0000"+
"\u0000\u01b7\u0113\u0001\u0000\u0000\u0000\u01b7\u0124\u0001\u0000\u0000"+
"\u0000\u01b7\u0137\u0001\u0000\u0000\u0000\u01b7\u0143\u0001\u0000\u0000"+
"\u0000\u01b7\u0151\u0001\u0000\u0000\u0000\u01b7\u015e\u0001\u0000\u0000"+
"\u0000\u01b7\u0172\u0001\u0000\u0000\u0000\u01b7\u017b\u0001\u0000\u0000"+
"\u0000\u01b7\u0187\u0001\u0000\u0000\u0000\u01b7\u0193\u0001\u0000\u0000"+
"\u0000\u01b7\u019d\u0001\u0000\u0000\u0000\u01b7\u01aa\u0001\u0000\u0000"+
"\u0000\u01b8\u0010\u0001\u0000\u0000\u0000\u01b9\u01ba\u0005@\u0000\u0000"+
"\u01ba\u01bb\u0005v\u0000\u0000\u01bb\u01bc\u0005i\u0000\u0000\u01bc\u01bd"+
"\u0005e\u0000\u0000\u01bd\u01be\u0005w\u0000\u0000\u01be\u01bf\u0005p"+
"\u0000\u0000\u01bf\u01c0\u0005o\u0000\u0000\u01c0\u01c1\u0005r\u0000\u0000"+
"\u01c1\u01c2\u0005t\u0000\u0000\u01c2\u0012\u0001\u0000\u0000\u0000\u01c3"+
"\u01c4\u0005@\u0000\u0000\u01c4\u01c5\u0005f\u0000\u0000\u01c5\u01c6\u0005"+
"o\u0000\u0000\u01c6\u01c7\u0005n\u0000\u0000\u01c7\u01c8\u0005t\u0000"+
"\u0000\u01c8\u01c9\u0005-\u0000\u0000\u01c9\u01ca\u0005f\u0000\u0000\u01ca"+
"\u01cb\u0005a\u0000\u0000\u01cb\u01cc\u0005c\u0000\u0000\u01cc\u01cd\u0005"+
"e\u0000\u0000\u01cd\u0014\u0001\u0000\u0000\u0000\u01ce\u01d0\u0005@\u0000"+
"\u0000\u01cf\u01d1\u0003W+\u0000\u01d0\u01cf\u0001\u0000\u0000\u0000\u01d0"+
"\u01d1\u0001\u0000\u0000\u0000\u01d1\u01d2\u0001\u0000\u0000\u0000\u01d2"+
"\u01d3\u0003{=\u0000\u01d3\u0016\u0001\u0000\u0000\u0000\u01d4\u01d5\u0005"+
".\u0000\u0000\u01d5\u01d6\u0003{=\u0000\u01d6\u0018\u0001\u0000\u0000"+
"\u0000\u01d7\u01d8\u0006\f\u0002\u0000\u01d8\u01d9\u0003\u008bE\u0000"+
"\u01d9\u01da\u0006\f\u0003\u0000\u01da\u001a\u0001\u0000\u0000\u0000\u01db"+
"\u01dc\u0003\u008dF\u0000\u01dc\u001c\u0001\u0000\u0000\u0000\u01dd\u01de"+
"\u0003].\u0000\u01de\u01df\u0003}>\u0000\u01df\u001e\u0001\u0000\u0000"+
"\u0000\u01e0\u01e2\u0003\u0087C\u0000\u01e1\u01e0\u0001\u0000\u0000\u0000"+
"\u01e1\u01e2\u0001\u0000\u0000\u0000\u01e2\u01e3\u0001\u0000\u0000\u0000"+
"\u01e3\u01f6\u0007\u0000\u0000\u0000\u01e4\u01e6\u0003c1\u0000\u01e5\u01e4"+
"\u0001\u0000\u0000\u0000\u01e6\u01e9\u0001\u0000\u0000\u0000\u01e7\u01e5"+
"\u0001\u0000\u0000\u0000\u01e7\u01e8\u0001\u0000\u0000\u0000\u01e8\u01ec"+
"\u0001\u0000\u0000\u0000\u01e9\u01e7\u0001\u0000\u0000\u0000\u01ea\u01ed"+
"\u0003Y,\u0000\u01eb\u01ed\u0003W+\u0000\u01ec\u01ea\u0001\u0000\u0000"+
"\u0000\u01ec\u01eb\u0001\u0000\u0000\u0000\u01ed\u01f1\u0001\u0000\u0000"+
"\u0000\u01ee\u01f0\u0003c1\u0000\u01ef\u01ee\u0001\u0000\u0000\u0000\u01f0"+
"\u01f3\u0001\u0000\u0000\u0000\u01f1\u01ef\u0001\u0000\u0000\u0000\u01f1"+
"\u01f2\u0001\u0000\u0000\u0000\u01f2\u01f4\u0001\u0000\u0000\u0000\u01f3"+
"\u01f1\u0001\u0000\u0000\u0000\u01f4\u01f5\u0003\u0087C\u0000\u01f5\u01f7"+
"\u0001\u0000\u0000\u0000\u01f6\u01e7\u0001\u0000\u0000\u0000\u01f6\u01f7"+
"\u0001\u0000\u0000\u0000\u01f7 \u0001\u0000\u0000\u0000\u01f8\u01f9\u0003"+
"\u0089D\u0000\u01f9\"\u0001\u0000\u0000\u0000\u01fa\u01fb\u0003\u0089"+
"D\u0000\u01fb\u01fc\u0005%\u0000\u0000\u01fc$\u0001\u0000\u0000\u0000"+
"\u01fd\u01fe\u0003\u0089D\u0000\u01fe\u01ff\u0003{=\u0000\u01ff&\u0001"+
"\u0000\u0000\u0000\u0200\u0201\u0005u\u0000\u0000\u0201\u0202\u0005r\u0000"+
"\u0000\u0202\u0203\u0005l\u0000\u0000\u0203\u0204\u0005(\u0000\u0000\u0204"+
"\u0205\u0001\u0000\u0000\u0000\u0205\u0208\u0003\u0097K\u0000\u0206\u0209"+
"\u0003\u008bE\u0000\u0207\u0209\u0003\u0091H\u0000\u0208\u0206\u0001\u0000"+
"\u0000\u0000\u0208\u0207\u0001\u0000\u0000\u0000\u0209\u020a\u0001\u0000"+
"\u0000\u0000\u020a\u020b\u0003\u0097K\u0000\u020b\u020c\u0005)\u0000\u0000"+
"\u020c(\u0001\u0000\u0000\u0000\u020d\u020e\u0005u\u0000\u0000\u020e\u020f"+
"\u0005r\u0000\u0000\u020f\u0210\u0005l\u0000\u0000\u0210\u0211\u0005("+
"\u0000\u0000\u0211\u0212\u0001\u0000\u0000\u0000\u0212\u0216\u0003\u0097"+
"K\u0000\u0213\u0217\u0003\u008bE\u0000\u0214\u0217\u0003\u008dF\u0000"+
"\u0215\u0217\u0003\u0091H\u0000\u0216\u0213\u0001\u0000\u0000\u0000\u0216"+
"\u0214\u0001\u0000\u0000\u0000\u0216\u0215\u0001\u0000\u0000\u0000\u0217"+
"\u0218\u0001\u0000\u0000\u0000\u0218\u0219\u0003\u0097K\u0000\u0219*\u0001"+
"\u0000\u0000\u0000\u021a\u021b\u0005U\u0000\u0000\u021b\u021c\u0005+\u0000"+
"\u0000\u021c\u021d\u0001\u0000\u0000\u0000\u021d\u021e\u0007\u0001\u0000"+
"\u0000\u021e\u021f\u0007\u0001\u0000\u0000\u021f\u0220\u0007\u0001\u0000"+
"\u0000\u0220\u0223\u0007\u0001\u0000\u0000\u0221\u0222\u0007\u0001\u0000"+
"\u0000\u0222\u0224\u0007\u0001\u0000\u0000\u0223\u0221\u0001\u0000\u0000"+
"\u0000\u0223\u0224\u0001\u0000\u0000\u0000\u0224\u022e\u0001\u0000\u0000"+
"\u0000\u0225\u0226\u0005-\u0000\u0000\u0226\u0227\u0007\u0002\u0000\u0000"+
"\u0227\u0228\u0007\u0002\u0000\u0000\u0228\u0229\u0007\u0002\u0000\u0000"+
"\u0229\u022c\u0007\u0002\u0000\u0000\u022a\u022b\u0007\u0002\u0000\u0000"+
"\u022b\u022d\u0007\u0002\u0000\u0000\u022c\u022a\u0001\u0000\u0000\u0000"+
"\u022c\u022d\u0001\u0000\u0000\u0000\u022d\u022f\u0001\u0000\u0000\u0000"+
"\u022e\u0225\u0001\u0000\u0000\u0000\u022e\u022f\u0001\u0000\u0000\u0000"+
"\u022f,\u0001\u0000\u0000\u0000\u0230\u0231\u0005<\u0000\u0000\u0231\u0232"+
"\u0005!\u0000\u0000\u0232\u0233\u0005-\u0000\u0000\u0233\u0234\u0005-"+
"\u0000\u0000\u0234.\u0001\u0000\u0000\u0000\u0235\u0236\u0005-\u0000\u0000"+
"\u0236\u0237\u0005-\u0000\u0000\u0237\u0238\u0005>\u0000\u0000\u02380"+
"\u0001\u0000\u0000\u0000\u0239\u023a\u0005;\u0000\u0000\u023a\u023b\u0006"+
"\u0018\u0004\u0000\u023b2\u0001\u0000\u0000\u0000\u023c\u023d\u0005:\u0000"+
"\u0000\u023d4\u0001\u0000\u0000\u0000\u023e\u023f\u0005,\u0000\u0000\u023f"+
"6\u0001\u0000\u0000\u0000\u0240\u0241\u0005?\u0000\u0000\u02418\u0001"+
"\u0000\u0000\u0000\u0242\u0243\u0005%\u0000\u0000\u0243:\u0001\u0000\u0000"+
"\u0000\u0244\u0245\u0005=\u0000\u0000\u0245<\u0001\u0000\u0000\u0000\u0246"+
"\u0247\u0005/\u0000\u0000\u0247>\u0001\u0000\u0000\u0000\u0248\u0249\u0005"+
">\u0000\u0000\u0249@\u0001\u0000\u0000\u0000\u024a\u024b\u0005<\u0000"+
"\u0000\u024bB\u0001\u0000\u0000\u0000\u024c\u024d\u0005{\u0000\u0000\u024d"+
"\u024e\u0006!\u0005\u0000\u024eD\u0001\u0000\u0000\u0000\u024f\u0250\u0005"+
"}\u0000\u0000\u0250\u0251\u0006\"\u0006\u0000\u0251F\u0001\u0000\u0000"+
"\u0000\u0252\u0253\u0005\'\u0000\u0000\u0253\u0254\u0006#\u0007\u0000"+
"\u0254H\u0001\u0000\u0000\u0000\u0255\u0256\u0005\"\u0000\u0000\u0256"+
"\u0257\u0006$\b\u0000\u0257J\u0001\u0000\u0000\u0000\u0258\u0259\u0005"+
"(\u0000\u0000\u0259\u025a\u0006%\t\u0000\u025aL\u0001\u0000\u0000\u0000"+
"\u025b\u025c\u0005)\u0000\u0000\u025c\u025d\u0006&\n\u0000\u025dN\u0001"+
"\u0000\u0000\u0000\u025e\u025f\u0005[\u0000\u0000\u025f\u0260\u0006\'"+
"\u000b\u0000\u0260P\u0001\u0000\u0000\u0000\u0261\u0262\u0005]\u0000\u0000"+
"\u0262\u0263\u0006(\f\u0000\u0263R\u0001\u0000\u0000\u0000\u0264\u0265"+
"\u0005!\u0000\u0000\u0265T\u0001\u0000\u0000\u0000\u0266\u0267\u0005~"+
"\u0000\u0000\u0267V\u0001\u0000\u0000\u0000\u0268\u0269\u0005-\u0000\u0000"+
"\u0269X\u0001\u0000\u0000\u0000\u026a\u026b\u0005+\u0000\u0000\u026bZ"+
"\u0001\u0000\u0000\u0000\u026c\u026d\u0005*\u0000\u0000\u026d\\\u0001"+
"\u0000\u0000\u0000\u026e\u026f\u0005#\u0000\u0000\u026f^\u0001\u0000\u0000"+
"\u0000\u0270\u0271\u0005&\u0000\u0000\u0271`\u0001\u0000\u0000\u0000\u0272"+
"\u0273\u0005^\u0000\u0000\u0273b\u0001\u0000\u0000\u0000\u0274\u0276\u0003"+
"\u0099L\u0000\u0275\u0274\u0001\u0000\u0000\u0000\u0276\u0277\u0001\u0000"+
"\u0000\u0000\u0277\u0275\u0001\u0000\u0000\u0000\u0277\u0278\u0001\u0000"+
"\u0000\u0000\u0278d\u0001\u0000\u0000\u0000\u0279\u027a\u0005/\u0000\u0000"+
"\u027a\u027b\u0005*\u0000\u0000\u027b\u027f\u0001\u0000\u0000\u0000\u027c"+
"\u027e\t\u0000\u0000\u0000\u027d\u027c\u0001\u0000\u0000\u0000\u027e\u0281"+
"\u0001\u0000\u0000\u0000\u027f\u0280\u0001\u0000\u0000\u0000\u027f\u027d"+
"\u0001\u0000\u0000\u0000\u0280\u0285\u0001\u0000\u0000\u0000\u0281\u027f"+
"\u0001\u0000\u0000\u0000\u0282\u0283\u0005*\u0000\u0000\u0283\u0286\u0005"+
"/\u0000\u0000\u0284\u0286\u0005\u0000\u0000\u0001\u0285\u0282\u0001\u0000"+
"\u0000\u0000\u0285\u0284\u0001\u0000\u0000\u0000\u0286\u0287\u0001\u0000"+
"\u0000\u0000\u0287\u0288\u00062\r\u0000\u0288f\u0001\u0000\u0000\u0000"+
"\u0289\u028a\u0005/\u0000\u0000\u028a\u028b\u0005/\u0000\u0000\u028b\u028f"+
"\u0001\u0000\u0000\u0000\u028c\u028e\t\u0000\u0000\u0000\u028d\u028c\u0001"+
"\u0000\u0000\u0000\u028e\u0291\u0001\u0000\u0000\u0000\u028f\u0290\u0001"+
"\u0000\u0000\u0000\u028f\u028d\u0001\u0000\u0000\u0000\u0290\u0292\u0001"+
"\u0000\u0000\u0000\u0291\u028f\u0001\u0000\u0000\u0000\u0292\u0293\u0007"+
"\u0003\u0000\u0000\u0293\u0294\u0001\u0000\u0000\u0000\u0294\u0295\u0006"+
"3\r\u0000\u0295h\u0001\u0000\u0000\u0000\u0296\u0297\u0005e\u0000\u0000"+
"\u0297\u0298\u0005x\u0000\u0000\u0298\u0299\u0005p\u0000\u0000\u0299\u029a"+
"\u0005r\u0000\u0000\u029a\u029b\u0005e\u0000\u0000\u029b\u029c\u0005s"+
"\u0000\u0000\u029c\u029d\u0005s\u0000\u0000\u029d\u029e\u0005i\u0000\u0000"+
"\u029e\u029f\u0005o\u0000\u0000\u029f\u02a0\u0005n\u0000\u0000\u02a0\u02a1"+
"\u0005(\u0000\u0000\u02a1j\u0001\u0000\u0000\u0000\u02a2\u02a3\u0003{"+
"=\u0000\u02a3\u02a4\u0005(\u0000\u0000\u02a4\u02a5\u00065\u000e\u0000"+
"\u02a5l\u0001\u0000\u0000\u0000\u02a6\u02a7\u0005~\u0000\u0000\u02a7\u02a8"+
"\u0005=\u0000\u0000\u02a8n\u0001\u0000\u0000\u0000\u02a9\u02aa\u0005|"+
"\u0000\u0000\u02aa\u02ab\u0005=\u0000\u0000\u02abp\u0001\u0000\u0000\u0000"+
"\u02ac\u02ad\u0005^\u0000\u0000\u02ad\u02ae\u0005=\u0000\u0000\u02aer"+
"\u0001\u0000\u0000\u0000\u02af\u02b0\u0005$\u0000\u0000\u02b0\u02b1\u0005"+
"=\u0000\u0000\u02b1t\u0001\u0000\u0000\u0000\u02b2\u02b3\u0005*\u0000"+
"\u0000\u02b3\u02b4\u0005=\u0000\u0000\u02b4v\u0001\u0000\u0000\u0000\u02b5"+
"\u02b7\u0003\u009bM\u0000\u02b6\u02b5\u0001\u0000\u0000\u0000\u02b7\u02b8"+
"\u0001\u0000\u0000\u0000\u02b8\u02b6\u0001\u0000\u0000\u0000\u02b8\u02b9"+
"\u0001\u0000\u0000\u0000\u02b9x\u0001\u0000\u0000\u0000\u02ba\u02bb\t"+
"\u0000\u0000\u0000\u02bbz\u0001\u0000\u0000\u0000\u02bc\u02c0\u0003\u007f"+
"?\u0000\u02bd\u02bf\u0003\u0085B\u0000\u02be\u02bd\u0001\u0000\u0000\u0000"+
"\u02bf\u02c2\u0001\u0000\u0000\u0000\u02c0\u02be\u0001\u0000\u0000\u0000"+
"\u02c0\u02c1\u0001\u0000\u0000\u0000\u02c1|\u0001\u0000\u0000\u0000\u02c2"+
"\u02c0\u0001\u0000\u0000\u0000\u02c3\u02c5\u0003\u0085B\u0000\u02c4\u02c3"+
"\u0001\u0000\u0000\u0000\u02c5\u02c6\u0001\u0000\u0000\u0000\u02c6\u02c4"+
"\u0001\u0000\u0000\u0000\u02c6\u02c7\u0001\u0000\u0000\u0000\u02c7~\u0001"+
"\u0000\u0000\u0000\u02c8\u02cc\u0007\u0004\u0000\u0000\u02c9\u02cc\u0003"+
"\u0081@\u0000\u02ca\u02cc\u0003\u0083A\u0000\u02cb\u02c8\u0001\u0000\u0000"+
"\u0000\u02cb\u02c9\u0001\u0000\u0000\u0000\u02cb\u02ca\u0001\u0000\u0000"+
"\u0000\u02cc\u0080\u0001\u0000\u0000\u0000\u02cd\u02ce\u0007\u0005\u0000"+
"\u0000\u02ce\u0082\u0001\u0000\u0000\u0000\u02cf\u02d9\u0005\\\u0000\u0000"+
"\u02d0\u02d2\u0007\u0002\u0000\u0000\u02d1\u02d0\u0001\u0000\u0000\u0000"+
"\u02d2\u02d3\u0001\u0000\u0000\u0000\u02d3\u02d1\u0001\u0000\u0000\u0000"+
"\u02d3\u02d4\u0001\u0000\u0000\u0000\u02d4\u02d6\u0001\u0000\u0000\u0000"+
"\u02d5\u02d7\u0003\u0099L\u0000\u02d6\u02d5\u0001\u0000\u0000\u0000\u02d6"+
"\u02d7\u0001\u0000\u0000\u0000\u02d7\u02da\u0001\u0000\u0000\u0000\u02d8"+
"\u02da\u0007\u0006\u0000\u0000\u02d9\u02d1\u0001\u0000\u0000\u0000\u02d9"+
"\u02d8\u0001\u0000\u0000\u0000\u02da\u0084\u0001\u0000\u0000\u0000\u02db"+
"\u02df\u0007\u0007\u0000\u0000\u02dc\u02df\u0003\u0081@\u0000\u02dd\u02df"+
"\u0003\u0083A\u0000\u02de\u02db\u0001\u0000\u0000\u0000\u02de\u02dc\u0001"+
"\u0000\u0000\u0000\u02de\u02dd\u0001\u0000\u0000\u0000\u02df\u0086\u0001"+
"\u0000\u0000\u0000\u02e0\u02e2\u000209\u0000\u02e1\u02e0\u0001\u0000\u0000"+
"\u0000\u02e2\u02e3\u0001\u0000\u0000\u0000\u02e3\u02e1\u0001\u0000\u0000"+
"\u0000\u02e3\u02e4\u0001\u0000\u0000\u0000\u02e4\u0088\u0001\u0000\u0000"+
"\u0000\u02e5\u02e7\u000209\u0000\u02e6\u02e5\u0001\u0000\u0000\u0000\u02e7"+
"\u02e8\u0001\u0000\u0000\u0000\u02e8\u02e6\u0001\u0000\u0000\u0000\u02e8"+
"\u02e9\u0001\u0000\u0000\u0000\u02e9\u02f7\u0001\u0000\u0000\u0000\u02ea"+
"\u02ec\u000209\u0000\u02eb\u02ea\u0001\u0000\u0000\u0000\u02ec\u02ef\u0001"+
"\u0000\u0000\u0000\u02ed\u02eb\u0001\u0000\u0000\u0000\u02ed\u02ee\u0001"+
"\u0000\u0000\u0000\u02ee\u02f0\u0001\u0000\u0000\u0000\u02ef\u02ed\u0001"+
"\u0000\u0000\u0000\u02f0\u02f2\u0005.\u0000\u0000\u02f1\u02f3\u000209"+
"\u0000\u02f2\u02f1\u0001\u0000\u0000\u0000\u02f3\u02f4\u0001\u0000\u0000"+
"\u0000\u02f4\u02f2\u0001\u0000\u0000\u0000\u02f4\u02f5\u0001\u0000\u0000"+
"\u0000\u02f5\u02f7\u0001\u0000\u0000\u0000\u02f6\u02e6\u0001\u0000\u0000"+
"\u0000\u02f6\u02ed\u0001\u0000\u0000\u0000\u02f7\u008a\u0001\u0000\u0000"+
"\u0000\u02f8\u02ff\u0003I$\u0000\u02f9\u02fe\u0003\u008fG\u0000\u02fa"+
"\u02fb\u0003G#\u0000\u02fb\u02fc\u0006E\u000f\u0000\u02fc\u02fe\u0001"+
"\u0000\u0000\u0000\u02fd\u02f9\u0001\u0000\u0000\u0000\u02fd\u02fa\u0001"+
"\u0000\u0000\u0000\u02fe\u0301\u0001\u0000\u0000\u0000\u02ff\u02fd\u0001"+
"\u0000\u0000\u0000\u02ff\u0300\u0001\u0000\u0000\u0000\u0300\u0302\u0001"+
"\u0000\u0000\u0000\u0301\u02ff\u0001\u0000\u0000\u0000\u0302\u0303\u0003"+
"I$\u0000\u0303\u0311\u0001\u0000\u0000\u0000\u0304\u030b\u0003G#\u0000"+
"\u0305\u030a\u0003\u008fG\u0000\u0306\u0307\u0003I$\u0000\u0307\u0308"+
"\u0006E\u0010\u0000\u0308\u030a\u0001\u0000\u0000\u0000\u0309\u0305\u0001"+
"\u0000\u0000\u0000\u0309\u0306\u0001\u0000\u0000\u0000\u030a\u030d\u0001"+
"\u0000\u0000\u0000\u030b\u0309\u0001\u0000\u0000\u0000\u030b\u030c\u0001"+
"\u0000\u0000\u0000\u030c\u030e\u0001\u0000\u0000\u0000\u030d\u030b\u0001"+
"\u0000\u0000\u0000\u030e\u030f\u0003G#\u0000\u030f\u0311\u0001\u0000\u0000"+
"\u0000\u0310\u02f8\u0001\u0000\u0000\u0000\u0310\u0304\u0001\u0000\u0000"+
"\u0000\u0311\u008c\u0001\u0000\u0000\u0000\u0312\u0319\u0003I$\u0000\u0313"+
"\u0318\u0003\u008fG\u0000\u0314\u0315\u0003G#\u0000\u0315\u0316\u0006"+
"F\u0011\u0000\u0316\u0318\u0001\u0000\u0000\u0000\u0317\u0313\u0001\u0000"+
"\u0000\u0000\u0317\u0314\u0001\u0000\u0000\u0000\u0318\u031b\u0001\u0000"+
"\u0000\u0000\u0319\u0317\u0001\u0000\u0000\u0000\u0319\u031a\u0001\u0000"+
"\u0000\u0000\u031a\u0327\u0001\u0000\u0000\u0000\u031b\u0319\u0001\u0000"+
"\u0000\u0000\u031c\u0323\u0003G#\u0000\u031d\u0322\u0003\u008fG\u0000"+
"\u031e\u031f\u0003I$\u0000\u031f\u0320\u0006F\u0012\u0000\u0320\u0322"+
"\u0001\u0000\u0000\u0000\u0321\u031d\u0001\u0000\u0000\u0000\u0321\u031e"+
"\u0001\u0000\u0000\u0000\u0322\u0325\u0001\u0000\u0000\u0000\u0323\u0321"+
"\u0001\u0000\u0000\u0000\u0323\u0324\u0001\u0000\u0000\u0000\u0324\u0327"+
"\u0001\u0000\u0000\u0000\u0325\u0323\u0001\u0000\u0000\u0000\u0326\u0312"+
"\u0001\u0000\u0000\u0000\u0326\u031c\u0001\u0000\u0000\u0000\u0327\u008e"+
"\u0001\u0000\u0000\u0000\u0328\u032d\u0003\u0093I\u0000\u0329\u032d\u0007"+
"\b\u0000\u0000\u032a\u032b\u0005\\\u0000\u0000\u032b\u032d\u0003\u0095"+
"J\u0000\u032c\u0328\u0001\u0000\u0000\u0000\u032c\u0329\u0001\u0000\u0000"+
"\u0000\u032c\u032a\u0001\u0000\u0000\u0000\u032d\u0090\u0001\u0000\u0000"+
"\u0000\u032e\u0330\u0003\u0093I\u0000\u032f\u032e\u0001\u0000\u0000\u0000"+
"\u0330\u0333\u0001\u0000\u0000\u0000\u0331\u032f\u0001\u0000\u0000\u0000"+
"\u0331\u0332\u0001\u0000\u0000\u0000\u0332\u0092\u0001\u0000\u0000\u0000"+
"\u0333\u0331\u0001\u0000\u0000\u0000\u0334\u0338\u0007\t\u0000\u0000\u0335"+
"\u0338\u0003\u0081@\u0000\u0336\u0338\u0003\u0083A\u0000\u0337\u0334\u0001"+
"\u0000\u0000\u0000\u0337\u0335\u0001\u0000\u0000\u0000\u0337\u0336\u0001"+
"\u0000\u0000\u0000\u0338\u0094\u0001\u0000\u0000\u0000\u0339\u033e\u0005"+
"\n\u0000\u0000\u033a\u033b\u0005\r\u0000\u0000\u033b\u033e\u0005\n\u0000"+
"\u0000\u033c\u033e\u0002\f\r\u0000\u033d\u0339\u0001\u0000\u0000\u0000"+
"\u033d\u033a\u0001\u0000\u0000\u0000\u033d\u033c\u0001\u0000\u0000\u0000"+
"\u033e\u0096\u0001\u0000\u0000\u0000\u033f\u0341\u0003\u0099L\u0000\u0340"+
"\u033f\u0001\u0000\u0000\u0000\u0341\u0344\u0001\u0000\u0000\u0000\u0342"+
"\u0340\u0001\u0000\u0000\u0000\u0342\u0343\u0001\u0000\u0000\u0000\u0343"+
"\u0098\u0001\u0000\u0000\u0000\u0344\u0342\u0001\u0000\u0000\u0000\u0345"+
"\u0346\u0007\n\u0000\u0000\u0346\u009a\u0001\u0000\u0000\u0000\u0347\u0348"+
"\u0007\u000b\u0000\u0000\u0348\u009c\u0001\u0000\u0000\u00000\u0000\u00b6"+
"\u00ba\u00bf\u01b7\u01d0\u01e1\u01e7\u01ec\u01f1\u01f6\u0208\u0216\u0223"+
"\u022c\u022e\u0277\u027f\u0285\u028f\u02b8\u02c0\u02c6\u02cb\u02d3\u02d6"+
"\u02d9\u02de\u02e3\u02e8\u02ed\u02f4\u02f6\u02fd\u02ff\u0309\u030b\u0310"+
"\u0317\u0319\u0321\u0323\u0326\u032c\u0331\u0337\u033d\u0342\u0013\u0001"+
"\u0002\u0000\u0001\u0002\u0001\u0001\f\u0002\u0001\f\u0003\u0001\u0018"+
"\u0004\u0001!\u0005\u0001\"\u0006\u0001#\u0007\u0001$\b\u0001%\t\u0001"+
"&\n\u0001\'\u000b\u0001(\f\u0000\u0001\u0000\u00015\r\u0001E\u000e\u0001"+
"E\u000f\u0001F\u0010\u0001F\u0011";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy