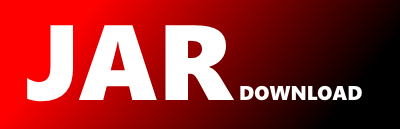
cz.vutbr.web.domassign.Traversal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jstyleparser Show documentation
Show all versions of jstyleparser Show documentation
jStyleParser is a CSS parser written in Java. It has its own application interface that is designed to allow an efficient CSS processing in Java and mapping the values to the Java data types. It parses CSS 2.1 style sheets into structures that can be efficiently assigned to DOM elements. It is intended be the primary CSS parser for the CSSBox library. While handling errors, it is user agent conforming according to the CSS specification.
The newest version!
package cz.vutbr.web.domassign;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.w3c.dom.traversal.DocumentTraversal;
import org.w3c.dom.traversal.TreeWalker;
/**
* This class implements traversal of DOM tree with simplified Visitor
* pattern.
*
* @author kapy
*
*/
public abstract class Traversal
{
protected Object source;
protected TreeWalker walker;
public Traversal(TreeWalker walker, Object source) {
this.source = source;
this.walker = walker;
}
public Traversal(Document doc, Object source, int whatToShow) {
if (doc instanceof DocumentTraversal) {
DocumentTraversal dt = (DocumentTraversal) doc;
this.walker = dt.createTreeWalker(doc.getDocumentElement(), whatToShow, null, false);
} else {
this.walker = new GenericTreeWalker(doc.getDocumentElement(), whatToShow);
}
this.source = source;
}
public void listTraversal(T result) {
// tree traversal as nodes are found inside
Node current, checkpoint = null;
current = walker.nextNode();
while (current != null) {
// this method can change position in walker
checkpoint = walker.getCurrentNode();
processNode(result, current, source);
walker.setCurrentNode(checkpoint);
current = walker.nextNode();
}
}
public void levelTraversal(T result) {
// this method can change position in walker
final Node checkpoint = walker.getCurrentNode();
processNode(result, checkpoint, source);
walker.setCurrentNode(checkpoint);
// traverse children:
for (Node n = walker.firstChild(); n != null; n = walker.nextSibling()) {
levelTraversal(result);
}
// return position to the current (level up):
walker.setCurrentNode(checkpoint);
}
protected abstract void processNode(T result, Node current, Object source);
public Traversal reset(TreeWalker walker, Object source) {
this.walker = walker;
this.source = source;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy