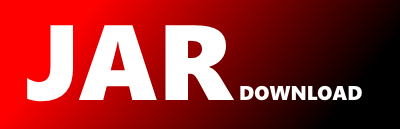
net.sf.cuf.debug.StateChecker Maven / Gradle / Ivy
package net.sf.cuf.debug;
import java.awt.BorderLayout;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSplitPane;
import javax.swing.JTable;
import javax.swing.JTextArea;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.EntityResolver;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import net.sf.cuf.model.DelegateAccess;
import net.sf.cuf.model.MixedAccessAdapter;
import net.sf.cuf.model.SelectionInList;
import net.sf.cuf.model.ui.ListTableMapper;
import net.sf.cuf.state.State;
import net.sf.cuf.ui.builder.SwingXMLBuilder;
/**
* Still in development!
*
* To use: e.g. call {@link #setupFromBuilder(String, SwingXMLBuilder)}
*/
public class StateChecker
{
private StateChecker()
{
}
public static void setupFromBuilder( final String pTitle, final SwingXMLBuilder pBuilder)
{
List stateInfos = getStateInfos( pBuilder);
buildDisplay( pTitle, pBuilder, stateInfos);
}
private static void buildDisplay(final String pFilename, final SwingXMLBuilder pBuilder, final List pStateInfos)
{
final JFrame frame = new JFrame("States for "+pFilename);
JPanel contentPane = new JPanel( new BorderLayout());
frame.setContentPane( contentPane );
JTable stateTable = new JTable();
final SelectionInList stateSIL = new SelectionInList( pStateInfos);
List mapping = new ArrayList();
AttrAdapter stateAA = new AttrAdapter( "state");
mapping.add( new ListTableMapper.Mapping( "ID", String.class, new AttrAdapter("id")));
mapping.add( new ListTableMapper.Mapping( "class", String.class, new DoubleDelegateAccess( stateAA, new ClassNameAdapter())));
mapping.add( new ListTableMapper.Mapping( "initialized", Boolean.class, new DoubleDelegateAccess( stateAA, new AttrAdapter("initialized","is","set"))));
mapping.add( new ListTableMapper.Mapping( "enabled", Boolean.class, new DoubleDelegateAccess( stateAA, new AttrAdapter("enabled","is","set"))));
new ListTableMapper( stateTable, stateSIL, mapping, false);
final ChangeListener updateListener = pE -> {
stateSIL.signalExternalUpdate();
stateSIL.selectionHolder().setValueForced( stateSIL.selectionHolder().getValue());
};
for (final Object pStateInfo : pStateInfos)
{
StateInfo stateInfo = (StateInfo) pStateInfo;
stateInfo.getState().addChangeListener(updateListener);
}
final JTextArea detailsTextArea = new JTextArea(30,100);
contentPane.add( new JSplitPane( JSplitPane.VERTICAL_SPLIT, new JScrollPane( stateTable), new JScrollPane( detailsTextArea)));
stateSIL.selectionHolder().addChangeListener(pE -> {
if (stateSIL.selectionHolder().intValue()>=0)
{
detailsTextArea.setText( getDetailsFor( (StateInfo) pStateInfos.get( stateSIL.selectionHolder().intValue())));
detailsTextArea.setCaretPosition( 0);
}
else
{
detailsTextArea.setText("");
}
});
frame.addWindowListener( new WindowAdapter(){
public void windowClosing(final WindowEvent pE)
{
for (final Object pStateInfo : pStateInfos)
{
StateInfo stateInfo = (StateInfo) pStateInfo;
stateInfo.getState().removeChangeListener(updateListener);
}
frame.setVisible(false);
frame.dispose();
}
});
frame.pack();
frame.setVisible(true);
}
protected static String getDetailsFor( final StateInfo pStateInfo)
{
return pStateInfo.getDetails();
}
private static List getStateInfos(final Document pDocument, final SwingXMLBuilder pBuilder)
{
List stateInfos = new ArrayList();
Node stateHandling = pDocument.getElementsByTagName( "statehandling").item(0);
NodeList childNodes = stateHandling.getChildNodes();
for (int i = 0; i < childNodes.getLength(); i++)
{
Node stateNode = childNodes.item(i);
if (stateNode.getAttributes()!=null)
{
Node idAttr = stateNode.getAttributes().getNamedItem("id");
if (idAttr!=null)
{
String id = idAttr.getNodeValue();
Object nonVisual = pBuilder.getNonVisualObject( id);
if (nonVisual instanceof State)
{
State state = (State) nonVisual;
stateInfos.add( new StateInfo( id, stateNode, state));
}
}
}
}
return stateInfos;
}
private static List getStateInfos(final SwingXMLBuilder pBuilder)
{
List stateInfos = new ArrayList();
Map nonvisualMap = pBuilder.getNameToNonVisual();
for (final Object o : nonvisualMap.keySet())
{
String key = (String) o;
Object nonVisual = nonvisualMap.get(key);
if (nonVisual instanceof State)
{
State state = (State) nonVisual;
stateInfos.add(new StateInfo(key, state));
}
}
return stateInfos;
}
private static Document scanXml(final String pFilename) throws SAXException, IOException, ParserConfigurationException
{
InputStream in = StateChecker.class.getResourceAsStream( pFilename);
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
db.setEntityResolver( new EntityHelper());
Document document = db.parse( in);
in.close();
return document;
}
/**
* helper class to resolve our dtd via a local file
*/
private static class EntityHelper implements EntityResolver
{
/**
* callback method from the XML parser to resolve public/system ID's
* @param pPublicId not used
* @param pSystemId checked against our SYSTEM_ID member
* @return null or a InputSource for pSystemId
*/
public InputSource resolveEntity (final String pPublicId, final String pSystemId)
{
if ("http://www.sdm.com/dtd/xml2swing-1.6.dtd".equals(pSystemId))
{
return loadDtd("xml2swing-1.6.dtd");
}
// if not one of our special SYSTEMID's, use the default behaviour
return null;
}
/**
* Small helper to load a file
* @param pFileName the dtd file name
* @return null or the InputSource for the file
*/
private InputSource loadDtd(final String pFileName)
{
InputSource back = null;
ClassLoader loader= Thread.currentThread().getContextClassLoader();
if (loader!=null)
{
InputStream dtd= loader.getResourceAsStream(pFileName);
if (dtd!=null)
{
back= new InputSource(dtd);
}
}
return back;
}
}
private static class AttrAdapter extends MixedAccessAdapter implements DelegateAccess
{
public AttrAdapter(final Class pSourceClass, final String pAspectName, final String pGetterPrefix, final String pSetterPrefix)
{
super(pSourceClass, pAspectName, pGetterPrefix, pSetterPrefix);
}
public AttrAdapter(final Class pSourceClass, final String pAspectName)
{
super(pSourceClass, pAspectName);
}
public AttrAdapter(final String pAspectName, final String pGetterPrefix, final String pSetterPrefix)
{
super(pAspectName, pGetterPrefix, pSetterPrefix);
}
public AttrAdapter(final String pAspectName)
{
super(pAspectName);
}
}
}