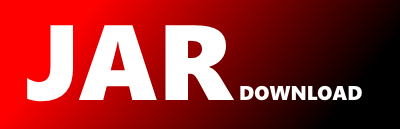
net.sf.cuf.debug.StateInfo Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.debug;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.lang.reflect.Field;
import java.util.List;
import org.w3c.dom.Node;
import net.sf.cuf.state.SimpleStateExpression;
import net.sf.cuf.state.State;
import net.sf.cuf.state.StateExpression;
public class StateInfo
{
private String mId;
private Node mStateNode;
private State mState;
public StateInfo(final String pId, final State pState)
{
this(pId, null, pState);
}
public StateInfo(final String pId, final Node pStateNode, final State pState)
{
mId = pId;
mStateNode = pStateNode;
mState = pState;
}
public String getId()
{
return mId;
}
public Node getStateNode()
{
return mStateNode;
}
public State getState()
{
return mState;
}
public String getDetails()
{
StringWriter sw = new StringWriter();
PrintWriter pw = new PrintWriter(sw);
getDetails(pw, "", mState);
pw.close();
return sw.toString();
}
private void getDetails(final PrintWriter pOut, final String pIndent, final State pState)
{
if (pState instanceof StateExpression)
{
pOut.println(pIndent + "Expression " + pState.getName() + ": " + getIniEnString(pState));
if (pState instanceof SimpleStateExpression)
{
SimpleStateExpression expression = (SimpleStateExpression) pState;
List states = (List) getPrivateValue(expression, "mStates");
List inverts = (List) getPrivateValue(expression, "mInverts");
List operations = (List) getPrivateValue(expression, "mOperations");
for (int i = 0; i < states.size(); i++)
{
pOut.println(pIndent + " " + operations.get(i) + (Boolean.TRUE.equals(inverts.get(i)) ? " not" : ""));
getDetails(pOut, pIndent + " ", (State) states.get(i));
}
}
}
else
{
pOut.println(pIndent + "State " + pState.getName() + ": " + getIniEnString(pState));
}
}
private static Object getPrivateValue(final Object pObj, final String pAttr)
{
try
{
Field field = pObj.getClass().getDeclaredField(pAttr);
field.setAccessible(true);
Object result = field.get(pObj);
field.setAccessible(false);
return result;
}
catch (Exception e)
{
e.printStackTrace();
return null;
}
}
private static String getIniEnString(final State pState)
{
return (pState.isInitialized() ? "initialized" : "not initialized") + (pState.isEnabled() ? " enabled" : " disabled");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy