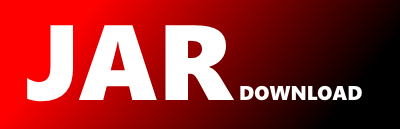
net.sf.cuf.fw.EDTExceptionUtil Maven / Gradle / Ivy
package net.sf.cuf.fw;
import javax.swing.SwingUtilities;
import java.io.FileOutputStream;
import java.io.PrintWriter;
import java.util.Date;
/**
* Small helper to display unhandled exceptions and abort the application.
* Since CUF 2.0 (which requires Java 7) we use the UncaughtExceptionHandler mechanism,
* before that we used the sun.awt.exception.handler hack. We ignore all exceptions not
* coming out of the EDT.
*
* To use, call {@link #install()}, (optionally) provide your application with {@link #setApp(Application)}
* and (optionally) configure your own error reporter with
* {@link #setFatalErrorReporter(FatalErrorReporter)}.
*/
@SuppressWarnings({"UseOfSystemOutOrSystemErr"})
public class EDTExceptionUtil implements Thread.UncaughtExceptionHandler
{
/** null or the back link to an application */
private static Application sApplication;
/**
* the implementation to use for error reporting, never null,
* initialized to DefaultFatalErrorReporter
*/
private static FatalErrorReporter sFatalErrorReporter = new DefaultFatalErrorReporter();
/** null or the version string of the application */
private static String sVersion;
/** null or the prefix we use for the dump file */
private static String sDumpPrefix;
/** path of our dump file **/
private static final String DUMP_FILE_PATH = System.getProperty("user.home") +
System.getProperty("file.separator");
/** suffix of our dump file */
private static final String DUMP_FILE_POSTFIX= "_dump.txt";
/**
* installs the exception handler by registering the uncaught exception handler.
*/
public static void install()
{
Thread.setDefaultUncaughtExceptionHandler(new EDTExceptionUtil());
}
/**
* Sets the fatal error reporter which is used to report an error.
* You may provide your own fatal error handler to replace the
* default behavior of the DefaultFatalErrorReporter.
* @param pFatalErrorReporter the reporter, must not be null
*/
public static void setFatalErrorReporter(final FatalErrorReporter pFatalErrorReporter)
{
if (pFatalErrorReporter==null)
{
throw new IllegalArgumentException("pFatalErrorReporter must not be null");
}
sFatalErrorReporter = pFatalErrorReporter;
}
/**
* Set back link to the application.
* If an error occurs, {@link net.sf.cuf.fw.Application#doStop()} will be called.
* @param pApp the application we are associated
*/
public static void setApp(final Application pApp)
{
sApplication = pApp;
}
/**
* Set application version string.
* @param pDumpPrefix for the dump file
* @param pVersion a version string for the application
*/
public static void setVersion(final String pDumpPrefix, final String pVersion)
{
sDumpPrefix= pDumpPrefix;
sVersion = pVersion;
}
/**
* The EDT exception handling expects a public empty constructor.
*/
public EDTExceptionUtil()
{
}
/** {@inheritDoc} */
@Override
public void uncaughtException(Thread t, Throwable pThrowable)
{
if(SwingUtilities.isEventDispatchThread())
{
handle(pThrowable);
}
}
/**
* This method gets called when an Exception is caught in the EDT.
*
* @param pThrowable caught Exception
*/
public void handle(final Throwable pThrowable)
{
sFatalErrorReporter.reportFatalError(pThrowable);
if (sApplication !=null)
{
sApplication.doStop();
}
System.err.println("could not stop application");
}
/**
* Interface to provide error reporting
*/
public interface FatalErrorReporter
{
/**
* report the fatal error to the user and write it to the log
* in an appropriate way.
* @param pThrowable the exception that is fatal
*/
void reportFatalError(Throwable pThrowable);
}
/**
* Default implementation of the error reporter,
* logs to System.err and tries to write the exception a file in the user directory.
*/
public static class DefaultFatalErrorReporter implements FatalErrorReporter
{
/**
* {@inheritDoc}
*/
public void reportFatalError(final Throwable pThrowable)
{
String message= "caught an unexpected exception on "+new Date()+" in the EventDispatchThread:";
System.err.println(message);
pThrowable.printStackTrace(System.err);
// try to save the information to a file
//noinspection EmptyCatchBlock
try
{
String dumpFileName;
if (sDumpPrefix!=null)
{
dumpFileName= DUMP_FILE_PATH+sDumpPrefix+DUMP_FILE_POSTFIX;
}
else
{
dumpFileName= DUMP_FILE_PATH+"unknown"+DUMP_FILE_POSTFIX;
}
FileOutputStream fout= new FileOutputStream(dumpFileName);
PrintWriter out = new PrintWriter(fout);
out.println(sVersion);
out.println(message);
pThrowable.printStackTrace(out);
out.close();
fout.close();
}
catch (Exception ignored)
{
}
}
}
}