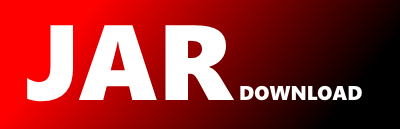
net.sf.cuf.fw2.GlassPane Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.fw2;
import java.awt.event.FocusEvent;
import java.awt.event.FocusListener;
import java.awt.event.KeyEvent;
import java.awt.event.MouseAdapter;
import javax.swing.InputVerifier;
import javax.swing.JComponent;
import javax.swing.SwingUtilities;
/**
* Small helper class for the block/unblock stuff.
*/
public class GlassPane extends JComponent
{
private static final long serialVersionUID = 1966978502308804795L;
/**
* Create a new pane that eats up all interaction.
*/
public GlassPane()
{
// handle all mouse events by ignoring them
addMouseListener(new MouseAdapter() { });
// disable tab handling
setNextFocusableComponent(this);
// Install a focus listener that refocuses the the glass pane
// if the focus should go to another component of the same window
// while the glass pane is visible.
addFocusListener( new FocusListener() {
public void focusGained(FocusEvent e)
{
// do nothing
}
public void focusLost(FocusEvent e)
{
if (isVisible())
{
if (e.getOppositeComponent()!=null)
{
if (SwingUtilities.getRoot(GlassPane.this) ==
SwingUtilities.getRoot(e.getOppositeComponent()))
{
requestFocus();
}
}
}
}
});
// And set up an input verifier to deny any focus changes
// done through the JComponent.requestFocus().
// Having an InputVerifier return false when the component is visible
// denies the focus change.
setInputVerifier( new InputVerifier()
{
public boolean verify(JComponent input)
{
return !isVisible();
}
public boolean shouldYieldFocus(JComponent input)
{
return !isVisible();
}
});
}
/**
* Consume all key events.
*/
protected void processKeyEvent(KeyEvent e)
{
e.consume();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy