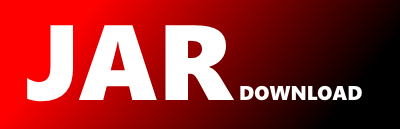
net.sf.cuf.model.LOVMapper Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import java.util.List;
/**
* A LOVMapper is a value model that maps between a display list-of-values (LOV)
* and their corresponding domain list-of-values.
* TODO: support also a single List where display and domain values are attributes.
*/
public class LOVMapper extends AbstractValueModel
© 2015 - 2025 Weber Informatics LLC | Privacy Policy