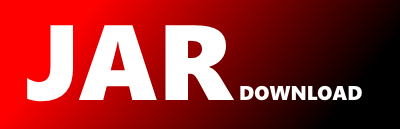
net.sf.cuf.model.MapAccessAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model;
import java.util.Map;
import java.util.StringTokenizer;
/**
* This adapter maps aspect names to a Map get/put operation.
* The disadvantege to a MethodAccessAdapter is that isEditable always
* returns true and most checks can't be done during the construction,
* so the MapAccessAdapter is less fail fast than the MethodAccessAdapter.
*/
public class MapAccessAdapter implements AspectAccessAdapter
{
// the keys for the map, never null
private String[] mKeys;
/**
* Creates a new adapter for a Map-based source.
* @param pAspectName the aspect name, may be separated by AspectAdapter.SEPARATOR
*/
public MapAccessAdapter(final String pAspectName)
{
// setup mKeys from pAspectName
StringTokenizer st= new StringTokenizer(pAspectName, AspectAdapter.SEPARATOR);
mKeys= new String[st.countTokens()];
for (int i= 0; i< mKeys.length; i++ )
{
mKeys[i]= st.nextToken();
}
}
/**
* Returns always true.
* @return true
*/
public boolean isEditable()
{
return true;
}
/**
* Extract the value from the source.
* @param pSource the source, may be null
* @return null or the value
*/
public Object getValue(final Object pSource)
{
if (pSource==null)
{
return null;
}
Object aspect= pSource;
for (final String mKey : mKeys)
{
Map source = (Map) aspect;
aspect = source.get(mKey);
}
return aspect;
}
/**
* Sets a value to the source.
* @param pSource the source, never null
* @param pValue the new value, may be null
*/
public void setValue(final Object pSource, final Object pValue)
{
Object aspect= pSource;
Map source= (Map)aspect;
for (final String mKey : mKeys)
{
source = (Map) aspect;
aspect = source.get(mKey);
}
source.put(mKeys[mKeys.length-1], pValue);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy