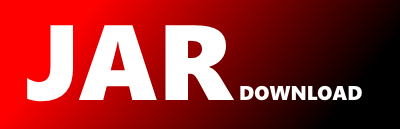
net.sf.cuf.model.PropertiesAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model;
import java.util.Properties;
/**
* PropertiesAdapter is a ValueModel that uses a Properties object
* as its backing store.
*/
public class PropertiesAdapter extends AbstractValueModel
© 2015 - 2025 Weber Informatics LLC | Privacy Policy