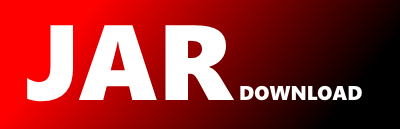
net.sf.cuf.model.ValueModel Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model;
import javax.swing.event.ChangeListener;
import java.util.List;
/**
* Java version of the Smalltalk/VisualWorks Value Model framework.
* See http://c2.com/ppr/vmodels.html for an introduction of the
* Smalltalk version.
* Because i was not sure what is the "right" listener registration
* method, i included both the smalltalk-like onChangeSend() as well
* as the Java-like addChangeListener() method.
* In any case, a change of the value of a ValueModel object will
* call the dependent method with a ChangeEvent as sole
* argument, the source of the event is always the ValueModel firing
* the event.
* @param the type we contain as our value
*/
public interface ValueModel
{
/*
* access stuff
*/
/**
* Get the current value, during a callback this is the new value.
* @return null or the value object
*/
T getValue();
/**
* Set a new value in this model. The method will fire a ChangeEvent
* to its listeners if the new value is different from the old value.
* If a ChangeEvent should always be sent, use #setValueForced.
* @param pValue the new value (null is o.k.)
* @throws UnsupportedOperationException if this ValueModel is not mutable
*/
void setValue(T pValue);
/**
* Needed since we generified everything, if we have no type given.
* @param pValue the value to be casted and set as new value
*/
void setObjectValue(Object pValue);
/**
* Set a new value in this model. The method will always fire a ChangeEvent
* to its listeners, even if the new value equals the old value.
* @param pValue the new value (null is o.k.)
* @throws UnsupportedOperationException if this ValueModel is not mutable
*/
void setValueForced(T pValue);
/**
* Set a new value, either forced or without force. The method might fire
* a ChangeEvent to its listeners.
* @param pValue the new value (null is o.k.)
* @param pIsSetForced true if a forced setValue should be done (see
* #setValueForced for details)
* @throws UnsupportedOperationException if this ValueModel is not mutable
*/
void setValue(T pValue, boolean pIsSetForced);
/**
* Returns true if setValueForced() was called, only useful during a callback.
* @return false if we are not inside a callback or a "normal" setValue() was issued
*/
boolean isSetForced();
/**
* Returns true if a setValue() is possible, and doesn't throw an
* UnsupportedOperationException,
* @return true if setValue() can be called
*/
boolean isEditable();
/*
* observer stuff
*/
/**
* Add an dependent listener to a ValueModel, "Smalltalk style".
* @param pDependent the object to call
* @param pMethodName the method name to call back
* @throws IllegalArgumentException if pDependent or pMethod is null,
* or no method with the signature
* "public void pMethodName(ChangeEvent e)"
* is found for pDependent.
*/
void onChangeSend(Object pDependent, String pMethodName);
/**
* Add an dependent listener to a ValueModel, "Java style".
* @param pDependent the object to call
* @throws IllegalArgumentException if pDependent is null
*/
void addChangeListener(ChangeListener pDependent);
/**
* Remove a dependent listener, "Smalltalk style".
* If pDependent is null or not known, nothing happens.
* @param pDependent object to de-register
*/
void retractInterestsFor(Object pDependent);
/**
* Remove a dependent listener, "Java style".
* If pDependent is null or not known, nothing happens.
* @param pDependent object to de-register
*/
void removeChangeListener(ChangeListener pDependent);
/**
* Cleanup all resources: disconnect from any input sources (like
* other ValueModel's ...), and remove all listeners.
* Any method besides isDisposed, dispose or getName will throw an
* IllegalStateException after the ValueModel was disposed.
*/
void dispose();
/**
* Check if the value model is disposed.
* @return true if the value model is disposed (= no longer valid)
*/
boolean isDisposed();
/**
* Return a immutable list of all direct dependents of the value model.
* @return an immutable List of all direct dependents
*/
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy