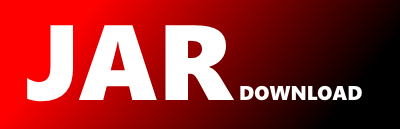
net.sf.cuf.model.converter.AbstractNumberStringConverter Maven / Gradle / Ivy
package net.sf.cuf.model.converter;
import net.sf.cuf.model.ValueModel;
/**
* A base class for converters that take a Number as it's subject value and converting
* it to an String as its own value.
*
* Derived classes must only implement {@link #convertToNumber(String)}
* and my override {@link #convertToString(Number)} if the default behaviour
* is not desired.
* @param the number type we support as our subjects type
*/
public abstract class AbstractNumberStringConverter extends AbstractTypeConverter
implements TypeConverter
{
/** marker if we should consider NULL as a valid value */
private boolean mNullIsValid;
/** the NULL subsitute, may be null */
private Number mNullSubstitute;
/**
* Create a new Number-to-String converter where null is a valid value.
* @param pSubject the ValueModel holding the original value
*/
public AbstractNumberStringConverter(final ValueModel pSubject)
{
this(pSubject, true);
}
/**
* Create a new Number-to-String converter.
* @param pSubject the ValueModel holding the original value
* @param pNullIsValid true if we should use null as an
* alternative value if the conversion fails
*/
public AbstractNumberStringConverter(final ValueModel pSubject, final boolean pNullIsValid)
{
super(pSubject);
mNullIsValid = pNullIsValid;
mNullSubstitute= null;
}
/**
* Sets the flag if we should use null as an alternative value if the conversion fails
* @param pNullIsValid true if we should use null as an
* alternative value if the conversion fails
*/
public void setNullIsValid(final boolean pNullIsValid)
{
mNullIsValid = pNullIsValid;
}
/**
* @return true if we should use null as an
* alternative value if the conversion fails
*/
public boolean getNullIsValid()
{
return mNullIsValid;
}
/**
* Method to set the Integer null substitute.
* @param pNullSubstitute null or any integer
* @throws IllegalStateException if null is not valid
*/
public void setNullSubstitute(final Number pNullSubstitute)
{
if (!mNullIsValid)
{
//noinspection ObjectToString
throw new IllegalStateException("null substitute "+pNullSubstitute+" set but null is not valid at all");
}
mNullSubstitute= pNullSubstitute;
}
/**
* Converts from a Number to a String.
* @param pSubjectValue value in the subject's type (Number)
* @return value in this value model's type (String)
*/
public String convertSubjectToOwnValue(final T pSubjectValue) throws ConversionException
{
String ownValue;
if (pSubjectValue==null)
{
if (mNullIsValid)
throw new ConversionException("Number subject is null", null, null);
else
throw new ConversionException("Number subject is null and !mNullIsValid", null);
}
else
{
ownValue= convertToString(pSubjectValue);
}
return ownValue;
}
/**
* Converts from a Number to the String by calling toString.
* Override to provide different behaviour.
*
* @param pSubjectValue the value in the source type (Number), never null
* @return value in this value model's type (String)
*/
protected String convertToString(final Number pSubjectValue)
{
//noinspection ObjectToString
return pSubjectValue.toString();
}
/**
* Converts from a String to an Number.
* @param pOwnValue value in this value model's type (String)
* @return value in the subject's type (at least a Number)
*/
public T convertOwnToSubjectValue(final String pOwnValue) throws ConversionException
{
T subjectValue;
try
{
subjectValue = convertToNumber(pOwnValue);
}
catch (Exception e)
{
if (mNullIsValid)
throw new ConversionException(e.getMessage(), e, mNullSubstitute);
else
throw new ConversionException(e.getMessage(), e);
}
return subjectValue;
}
/**
* convert the own string value to the number.
* Implement this method to create your number instance,
* e.g. like
*
* return new Integer( ownValue);
*
.
* If the conversion is not possible, you can throw a {@link NumberFormatException}
* which will be converted into a {@link ConversionException} with the correct
* substitute values.
* @param pOwnValue the value as a string
* @return the parsed number in its desired class
* @throws NumberFormatException if the string value is not parsable into a number
*/
protected abstract T convertToNumber(String pOwnValue) throws NumberFormatException;
}