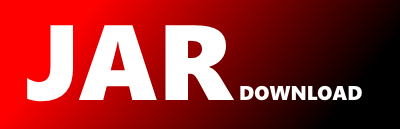
net.sf.cuf.model.converter.BigDecimalStringConverter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.converter;
import java.math.BigDecimal;
import java.text.NumberFormat;
import net.sf.cuf.model.ValueModel;
/**
* A BigDecimalStringConverter is taking a BigDecimal as it's subject value and converting
* it to an String as its own value.
*/
public class BigDecimalStringConverter extends AbstractTypeConverter
implements TypeConverter
{
/** marker if we should consider NULL as a valid value */
private boolean mNullIsValid;
/** the (optional) formatter/parser to be used, may be null */
private NumberFormat mFormat;
/** the BigDecimal subsitute, may be null */
private BigDecimal mNullSubstitute;
/**
* Create a new BigDecimal-to-String converter where null is a valid value.
* @param pSubject the ValueModel holding the original value
*/
public BigDecimalStringConverter(final ValueModel pSubject)
{
this(pSubject, null, true);
}
/**
* Create a new BigDecimal-to-String converter.
* @param pSubject the ValueModel holding the original value
* @param pNullIsValid marker if we should use null as an
* alternative value if the conversion fails
*/
public BigDecimalStringConverter(final ValueModel pSubject, final boolean pNullIsValid)
{
this(pSubject, null, pNullIsValid);
}
/**
* Create a new BigDecimal-to-String converter where null is a valid value.
* @param pSubject the ValueModel holding the original value
* @param pFormat the number formatter, may be null
*/
public BigDecimalStringConverter(final ValueModel pSubject, final NumberFormat pFormat)
{
this(pSubject, pFormat, true);
}
/**
* Create a new BigDecimal-to-String converter.
* @param pSubject the ValueModel holding the original value
* @param pFormat the number formatter, may be null
* @param pNullIsValid marker if we should use null as an
* alternative value if the conversion fails
*/
public BigDecimalStringConverter(final ValueModel pSubject, final NumberFormat pFormat, final boolean pNullIsValid)
{
super(pSubject);
mNullIsValid= pNullIsValid;
mFormat = pFormat;
}
/**
* Method to set the BigDecimal null subsitute.
* @param pNullSubstitute null or any BigDecimal
* @throws IllegalStateException if null is not valid
*/
public void setNullSubstitute(final BigDecimal pNullSubstitute)
{
if (!mNullIsValid)
{
throw new IllegalStateException("null substitute "+pNullSubstitute+" set but null is not valid at all");
}
mNullSubstitute= pNullSubstitute;
}
/**
* Converts from a BigDecimal to a String.
* @param pSubjectValue value in the subject's type (BigDecimal)
* @return value in this value model's type (String)
*/
public String convertSubjectToOwnValue(final BigDecimal pSubjectValue) throws ConversionException
{
String ownValue;
if (pSubjectValue==null)
{
if (mNullIsValid)
throw new ConversionException("BigDecimal subject is null", null, null);
else
throw new ConversionException("BigDecimal subject is null and !mNullIsValid", null);
}
else
{
if (mFormat != null)
{
ownValue= mFormat.format(pSubjectValue.doubleValue());
}
else
{
ownValue= pSubjectValue.toString();
}
}
return ownValue;
}
/**
* Converts from a String to an BigDecimal.
* @param pOwnValue value in this value model's type (String)
* @return value in the subject's type (BigDecimal)
* @throws ConversionException if we detected an error during the conversion
*/
public BigDecimal convertOwnToSubjectValue(final String pOwnValue) throws ConversionException
{
BigDecimal subjectValue;
try
{
if (mFormat != null)
{
subjectValue = (BigDecimal)mFormat.parse(pOwnValue);
}
else
{
subjectValue= new BigDecimal(pOwnValue);
}
}
catch (Exception e)
{
if (mNullIsValid)
throw new ConversionException(e.getMessage(), e, mNullSubstitute);
else
throw new ConversionException(e.getMessage(), e);
}
return subjectValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy