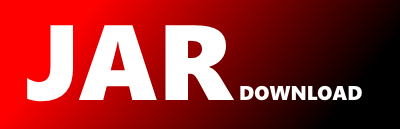
net.sf.cuf.model.converter.DoubleStringConverter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.converter;
import java.text.NumberFormat;
import java.text.ParseException;
import net.sf.cuf.model.ValueModel;
/**
* A DoubleStringConverter is taking a Double as it's subject value and converting
* it to an String as its own value.
*/
public class DoubleStringConverter extends AbstractNumberStringConverter
{
/** the (optional) formatter/parser to be used, may be null */
private NumberFormat mFormat;
/**
* Create a new Double-to-String converter where null is a valid value.
* @param pSubject the ValueModel holding the original value
*/
public DoubleStringConverter(final ValueModel pSubject)
{
this(pSubject, null, true);
}
/**
* Create a new Double-to-String converter.
* @param pSubject the ValueModel holding the original value
* @param pNullIsValid marker if we should use null as an
* alternative value if the conversion fails
*/
public DoubleStringConverter(final ValueModel pSubject, final boolean pNullIsValid)
{
this(pSubject, null, pNullIsValid);
}
/**
* Create a new Double-to-String converter where null is a valid value.
* @param pSubject the ValueModel holding the original value
* @param pFormat the number formatter, may be null
*/
public DoubleStringConverter(final ValueModel pSubject, final NumberFormat pFormat)
{
this(pSubject, pFormat, true);
}
/**
* Create a new Double-to-String converter.
* @param pSubject the ValueModel holding the original value
* @param pFormat the number formatter, may be null
* @param pNullIsValid marker if we should use null as an
* alternative value if the conversion fails
*/
public DoubleStringConverter(final ValueModel pSubject, final NumberFormat pFormat, final boolean pNullIsValid)
{
super(pSubject, pNullIsValid);
mFormat= pFormat;
}
/**
* {@inheritDoc}
*/
protected String convertToString(final Number pSubjectValue)
{
if (mFormat != null)
{
return mFormat.format(pSubjectValue.doubleValue());
}
else
{
//noinspection ObjectToString
return pSubjectValue.toString();
}
}
/**
* {@inheritDoc}
*/
protected Double convertToNumber(final String pOwnValue) throws NumberFormatException
{
if (mFormat != null)
{
try
{
return mFormat.parse(pOwnValue).doubleValue();
}
catch (ParseException e)
{
NumberFormatException ex = new NumberFormatException( "Could not parse string: "+e);
ex.initCause( e);
throw ex;
}
}
else
{
return new Double(pOwnValue);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy