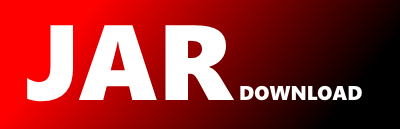
net.sf.cuf.model.converter.FormatConverter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.converter;
import net.sf.cuf.model.ValueModel;
import java.text.Format;
import java.text.ParseException;
import java.text.ParsePosition;
/**
* A FormatConverter uses the java.text.Format framework for doing
* the type conversion. In convertSubjectToOwnValue() the Format.format()
* method is used, in convertOwnToSubjectValue() the Format.parseObject()
* method is used.
* @param the type of the own value
* @param the type of the subject value
*/
public class FormatConverter extends AbstractTypeConverter implements TypeConverter
{
/** the format we use to convert our subjects value from/to */
private Format mFormat;
/** marker if we invert the parsing/formatting logic */
private boolean mInvert;
/**
* Create a new converter with the handed subject.
* @param pSubject the ValueModel holding the original value, must not be null
* @param pFormat the format we should use for formatting/parsing
* @param pInvert if this is true, we invert the conversion
* @throws IllegalArgumentException if pSubject or pFormat is null
*/
public FormatConverter(final ValueModel pSubject, final Format pFormat, final boolean pInvert)
{
super(pSubject, false);
if (pFormat==null)
{
throw new IllegalArgumentException("format must not be null");
}
mFormat= pFormat;
mInvert= pInvert;
}
/**
* Create a new converter with the handed subject.
* @param pSubject the ValueModel holding the original value, must not be null
* @param pFormat the format we should use for formatting/parsing
* @throws IllegalArgumentException if pSubject or pFormat is null
*/
public FormatConverter(final ValueModel pSubject, final Format pFormat)
{
this(pSubject, pFormat, false);
}
/**
* Converts from the type that the subject value model provides
* to the type this value model offers
* @param pSubjectValue value in the subject's type
* @return value in this value model's type
*/
public OwnT convertSubjectToOwnValue(final SubjectT pSubjectValue) throws ConversionException
{
if (mInvert)
return (OwnT)parse(pSubjectValue);
else
return (OwnT)format(pSubjectValue);
}
/**
* Format the handed object.
* @param pObjectToFormat the object to format
* @return the formatted object
* @throws ConversionException on conversation problems
*/
private Object format(final Object pObjectToFormat) throws ConversionException
{
if (pObjectToFormat == null)
{
return null;
}
else
{
try
{
return mFormat.format(pObjectToFormat);
}
catch (IllegalArgumentException e)
{
throw new ConversionException("could not parse with format", e);
}
}
}
/**
* Converts from the type that this value model offers to the type
* of the subject value model.
* @param pOwnValue value in this value model's type
* @return value in the subject's type
*/
public SubjectT convertOwnToSubjectValue(final OwnT pOwnValue) throws ConversionException
{
if (mInvert)
return (SubjectT)format(pOwnValue);
else
return (SubjectT)parse(pOwnValue);
}
/**
* Parse the handed object.
* @param pObjectToParse the object to parse
* @return the parsed object
* @throws ConversionException on conversation problems
*/
private Object parse(final Object pObjectToParse) throws ConversionException
{
String ownValue = "";
if (pObjectToParse != null)
{
ownValue= pObjectToParse.toString();
}
Object subjectValue;
ParsePosition pos = new ParsePosition(0);
subjectValue= mFormat.parseObject(ownValue, pos);
if (pos.getIndex()!=ownValue.length())
{
//noinspection ThrowableInstanceNeverThrown
throw new ConversionException(
"could not parse with format",
new ParseException("Format.parseObject(String) failed",
pos.getErrorIndex()).fillInStackTrace());
}
return subjectValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy