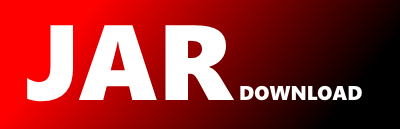
net.sf.cuf.model.converter.RegExpConverter Maven / Gradle / Ivy
package net.sf.cuf.model.converter;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import net.sf.cuf.model.ValueModel;
/**
* A RegExpConverter uses a regular expression for a string-to-string conversion.
* The main use of a RegExpConverter is obviously not to convert something,
* but to provide a convenient way of validating input.
*
* Note that by default the RegExpConverter blocks values that don't match its
* pattern and doesn't pass them through (both directions).
*/
public class RegExpConverter extends AbstractTypeConverter
implements TypeConverter
{
/** the pattern we use to convert our subjects value from/to */
private Pattern mPattern;
/** should we block values when the tests fail? */
private boolean mBlockValue;
/**
* Create a new converter with the handed subject.
* @param pSubject the ValueModel holding the original value, must not be null
* @param pRegExp the regular expression we should use for formatting/parsing
* @throws IllegalArgumentException if pSubject or pRegExp is null or pRegExp is not valid
*/
public RegExpConverter(final ValueModel pSubject, final String pRegExp)
{
this(pSubject,pRegExp,true);
}
/**
* Create a new converter with the handed subject.
* @param pSubject the ValueModel holding the original value, must not be null
* @param pRegExp the regular expression we should use for formatting/parsing
* @param pBlockValue if true, values are blocked if they don't match the regexp,
* if false, they are propagated
* @throws IllegalArgumentException if pSubject or pRegExp is null or pRegExp is not valid
*/
public RegExpConverter(final ValueModel pSubject, final String pRegExp, final boolean pBlockValue)
{
super(pSubject, false);
if (pRegExp==null)
{
throw new IllegalArgumentException("regExp must not be null");
}
mPattern= Pattern.compile(pRegExp);
mBlockValue = pBlockValue;
}
/**
* @return true if we should block values when the tests fail
*/
public boolean getBlockValue()
{
return mBlockValue;
}
/**
* sets the block behavious
* @param pBlockValue if true, values are blocked if they don't match the regexp,
* if false, they are propagated
*/
public void setBlockValue(final boolean pBlockValue)
{
mBlockValue = pBlockValue;
}
/**
* Converts from the type that the subject value model provides
* to the type this value model offers
* @param pSubjectValue value in the subject's type
* @return value in this value model's type
*/
public String convertSubjectToOwnValue(final String pSubjectValue) throws ConversionException
{
if (pSubjectValue == null)
{
return null;
}
Matcher matcher= mPattern.matcher(pSubjectValue);
if (matcher.matches())
{
return pSubjectValue;
}
else
{
if (mBlockValue)
{
throw new ConversionException("subject value does not match pattern", null);
}
else
{
throw new ConversionException("subject value does not match pattern", null, pSubjectValue);
}
}
}
/**
* Converts from the type that this value model offers to the type
* of the subject value model.
* @param pOwnValue value in this value model's type
* @return value in the subject's type
*/
public String convertOwnToSubjectValue(final String pOwnValue) throws ConversionException
{
if (pOwnValue == null)
{
return null;
}
Matcher matcher= mPattern.matcher(pOwnValue);
if (!matcher.matches())
{
if (mBlockValue)
{
throw new ConversionException("string "+pOwnValue+
" doesn't match pattern "+mPattern.pattern(),
null);
}
else
{
throw new ConversionException("string "+pOwnValue+
" doesn't match pattern "+mPattern.pattern(),
null, pOwnValue);
}
}
return pOwnValue;
}
}