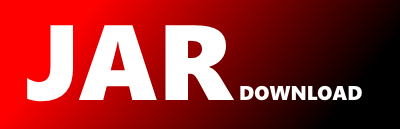
net.sf.cuf.model.state.RegExpState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.state;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import net.sf.cuf.model.ValueModel;
import net.sf.cuf.state.AbstractState;
/**
* The RegExpState is a state that is enabled if value model has a string value
* that matches the given regular expression. If the value model has a string
* value that does not match the regular expression or a value of different
* type (not a CharSequence), this state is disabled.
*/
public class RegExpState extends AbstractState implements ChangeListener
{
/**
* the value model we are responsible for, never null
*/
private ValueModel> mTrigger;
/**
* the compiled pattern for checking the value
*/
private Pattern mPattern;
/**
* Creates a new RegExpState for the given value model and
* the given regular expression pattern
* @param pTrigger the trigger value model whose value is observed and checked, must not be null
* @param pRegExpr the regular expression pattern, must not be null
*/
public RegExpState(final ValueModel> pTrigger, final String pRegExpr)
{
if (pTrigger==null)
{
throw new IllegalArgumentException( "pTrigger must not be null");
}
if (pRegExpr==null)
{
throw new IllegalArgumentException( "pRegExpr must not be null");
}
mTrigger = pTrigger;
mPattern = Pattern.compile( pRegExpr);
mIsInitialized = true;
mTrigger.addChangeListener( this);
checkTriggerValue();
}
/**
* called when our trigger changes
* {@inheritDoc}
*/
public void stateChanged(final ChangeEvent pE)
{
checkTriggerValue();
}
/**
* checks the value of our trigger and computes this state value
*/
private void checkTriggerValue()
{
boolean newIsEnabled = false;
Object value = mTrigger.getValue();
if (value instanceof CharSequence)
{
Matcher matcher= mPattern.matcher((CharSequence)value);
newIsEnabled = matcher.matches();
}
if (newIsEnabled!=mIsEnabled)
{
mIsEnabled = newIsEnabled;
fireStateChanged();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy