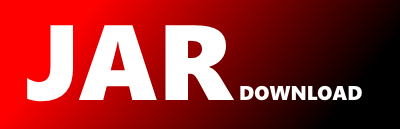
net.sf.cuf.model.state.ValueState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.state;
import net.sf.cuf.state.SimpleState;
import net.sf.cuf.state.MutableState;
import net.sf.cuf.model.ValueModel;
import javax.swing.event.ChangeListener;
import javax.swing.event.ChangeEvent;
/**
* A ValueState object wraps a ValueModel as a MutableState.
* The true/false state of a MutableState is mapped to setting the value
* of the ValueModel to Boolean.TRUE/Boolean.FALSE.
* The isInitialized()/reset() semantics are mapped to setting
* the value of the ValueModel to null.
*/
public class ValueState extends SimpleState implements MutableState, ChangeListener
{
/** string we consider as true */
private static final String TRUE = Boolean.TRUE.toString();
/** the ValueModel we wrap, never null */
private ValueModel> mModel;
/**
* If the property is null (default), then a value of null in the value model means
* that the state will be not {@link #isInitialized()}.
* If the property is {@link Boolean#TRUE}, then a value of null
* in the value model means that the state is enabled.
* If the property is {@link Boolean#FALSE}, then a value of null
* in the value model means that the state is disabled.
*/
private Boolean mMeaningOfNull;
/**
* We wrap a ValueModel as a MutableState object.
* @param pModel model we wrap, must not be null
* @throws IllegalArgumentException if pModel is null
*/
public ValueState(final ValueModel> pModel)
{
if (pModel==null)
throw new IllegalStateException("model must not be null");
mModel= pModel;
propagateModelToState();
mModel.addChangeListener(this);
}
/**
* Sets the state, the wrapped ValueModel will be set to Boolean.TRUE or Boolean.FALSE.
* @param pEnabled true if the state gets enabled
*/
public void setEnabled(final boolean pEnabled)
{
mInSetEnabled= true;
try
{
mModel.setValue(pEnabled);
super.setEnabled(pEnabled);
}
finally
{
mInSetEnabled= false;
}
}
/**
* Sets the state with a reason object. The reason object
* may be null, and is returned to a getChangeReason() call
* during the callback.
* @param pEnabled true if the state gets enabled
* @param pReason null or reason for the change
*/
public void setEnabled(final boolean pEnabled, final Object pReason)
{
mModel.setValue(pEnabled);
super.setEnabled(pEnabled, pReason);
}
/**
* Resets a MutableState to not initilized. Calling this
* method will not trigger a notification of the
* (State) listeners. The value of the ValueModel will
* be set to null.
*/
public void reset()
{
mModel.setValue(null);
super.reset();
}
/**
* configures the behaviour of the state when the
* value model has the value null.
* If the parameter is null (default), then a value of null in the value model means
* that the state will be not {@link #isInitialized()}.
* If the parameter is {@link Boolean#TRUE}, then a value of null
* in the value model means that the state is enabled.
* If the parameter is {@link Boolean#FALSE}, then a value of null
* in the value model means that the state is disabled.
*
* @param pMeaningOfNull the meaning of null
*/
public void setMeaningOfNull(final Boolean pMeaningOfNull)
{
mMeaningOfNull = pMeaningOfNull;
propagateModelToState();
}
/**
* @return the meaning of null
* @see #setMeaningOfNull(Boolean)
*/
public Boolean getMeaningOfNull()
{
return mMeaningOfNull;
}
/**
* Invoked when the target of the listener has changed its state.
*
* @param pEvent a ChangeEvent object
*/
public void stateChanged(final ChangeEvent pEvent)
{
if (inSetEnabled())
{
return;
}
propagateModelToState();
}
/**
* Small helper that propagates the value of the ValueModel to our state.
*/
private void propagateModelToState()
{
if (mModel.getValue()==null)
{
if (mMeaningOfNull==null)
{
super.reset();
}
else
{
super.setEnabled(mMeaningOfNull, mModel);
}
}
else
{
super.setEnabled(getModelAsBoolean(mModel), mModel);
}
}
/**
* Small helper to get the boolean value of the value of a ValueModel.
* @param pModel the model we examine, must not be null
* @return true if pModel is Boolean.TRUE or "true", false otherwise
*/
private boolean getModelAsBoolean(final ValueModel> pModel)
{
Object value= pModel.getValue();
if (value==null)
{
return false;
}
if (value instanceof Boolean)
{
return (Boolean) value;
}
return value.toString().equals(TRUE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy