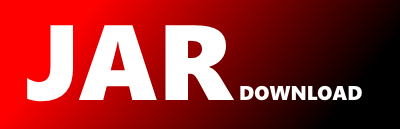
net.sf.cuf.model.ui.ButtonModelAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.ui;
import net.sf.cuf.model.ValueModel;
import javax.swing.ButtonModel;
import javax.swing.event.ChangeListener;
import javax.swing.event.ChangeEvent;
/**
* A ButtonModelAdapter connects the state of a ValueModel to the
* selection state of a ButtonModel. Whenever one of the model
* changes, the other is adjusted accordingly.
* A ButtonModelAdapter is itself not a ValueModel, but connects a
* ValueModel to a ButtonModel.
* The ValueModel should hold a Boolean, at least this adapter will
* write a Boolean to the ValueModel.
*/
public class ButtonModelAdapter implements ChangeListener
{
/** the value model we adapt to, never null */
private ValueModel> mValueModel;
/** the button model we adapt to, never null */
private ButtonModel mButtonModel;
/** last state of our button model */
private boolean mWasSelected;
/** marker if we are in stateChange */
private boolean mInStateChanged;
/** marker if we have inverted logic */
private boolean mInvert;
/**
* Creates a new adapter between a ValueModel and a ButtonModel .
* Whenever one of the models changes, the other is adjusted accordingly.
* The initial selection state is taken from the value model.
* @param pValueModel the value model
* @param pButtonModel the button model
*/
public ButtonModelAdapter(final ValueModel> pValueModel, final ButtonModel pButtonModel)
{
this(pValueModel, pButtonModel, false);
}
/**
* Creates a new adapter between a ValueModel and a ButtonModel .
* Whenever one of the models changes, the other is adjusted accordingly.
* The initial selection state is taken from the value model.
* @param pValueModel the value model
* @param pButtonModel the button model
* @param pInvert if true, we use inverted logic
*/
public ButtonModelAdapter(final ValueModel> pValueModel,
final ButtonModel pButtonModel,
final boolean pInvert)
{
if (pValueModel==null)
throw new IllegalArgumentException("value model must not be null");
if (pButtonModel==null)
throw new IllegalArgumentException("button model must not be null");
mValueModel = pValueModel;
mButtonModel= pButtonModel;
mInvert = pInvert;
mButtonModel.setSelected(isValueModelSelected());
mWasSelected = mButtonModel.isSelected();
mInStateChanged= false;
mButtonModel.addChangeListener(this);
mValueModel. addChangeListener(this);
}
/**
* Invoked when either the value model or the button model changed its state.
*
* @param pEvent a ChangeEvent object
*/
public void stateChanged(final ChangeEvent pEvent)
{
if (mInStateChanged)
{
return;
}
mInStateChanged= true;
try
{
Object source= pEvent.getSource();
//noinspection ObjectEquality
if (source == mValueModel)
{
// ValueModel callback
boolean isSelected= isValueModelSelected();
mButtonModel.setSelected(isSelected);
mWasSelected= isSelected;
}
else //noinspection ObjectEquality
if (source== mButtonModel)
{
// ButtonModel callback
boolean isSelected = isButtonModelSelected();
if (isSelected!=mWasSelected)
{
mValueModel.setValue(isSelected);
mWasSelected= isSelected;
}
}
else
{
// should never happen
throw new IllegalStateException("stateChanged with wrong source"+source);
}
}
finally
{
mInStateChanged= false;
}
}
/**
* Helper to extract the boolean from our ButtonModel.
* @return true if the ButtonModel is true and mInvert is false
*/
private boolean isButtonModelSelected()
{
boolean isSelected= mButtonModel.isSelected();
if (mInvert)
{
isSelected= !isSelected;
}
return isSelected;
}
/**
* Helper to extract the boolean from our ValueModel.
* @return true if the ValueModel contains "true" or Boolean.TRUE and mInvert is false
*/
private boolean isValueModelSelected()
{
boolean isSelected= mValueModel.booleanValue();
if (mInvert)
{
isSelected= !isSelected;
}
return isSelected;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy