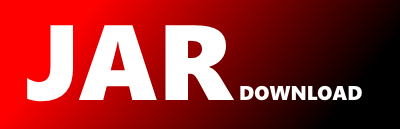
net.sf.cuf.model.ui.ComboBoxModelAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.ui;
import net.sf.cuf.model.ValueModel;
import javax.swing.ComboBoxModel;
import javax.swing.event.ChangeListener;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ListDataListener;
import javax.swing.event.ListDataEvent;
/**
* A ComboBoxModelAdapter connects a model of a JComboBox to a ValueModel
* holding an Integer with the index for the selected combobox item.
* The ValueModel holding the Integer is often the selectionHolder()
* of a SelectionInList ValueModel.
* Whenever either the selection of the combobox or
* the index of the ValueModel changes, the other model
* is adjusted accordingly.
* The "magic value" of -1 is used to signal no selection at all.
* A ComboBoxModelAdapter is not a ValueModel itself, but connects a
* ValueModel holding an integer to a ComboBoxModel.
*/
public class ComboBoxModelAdapter implements ListDataListener, ChangeListener
{
/** the value model we are watching, never null */
private ValueModel mSelectionHolder;
/** the combobox model, never null */
private ComboBoxModel mComboBoxModel;
/** marker if we are in an list data or state changed callback */
private boolean mInCallback;
/** no item is selected */
public static final Integer NO_SELECTION= -1;
/**
* Creates a new adapter between a ValueModel holding an Integer
* and a list of ButtonModels
* Whenever one of the models changes, the other is adjusted accordingly.
* The initial selected buttonmodel is taken from the value model.
*
* @param pSelectionHolder ValueModel holding an Integer
* @param pComboBoxModel a combobox model
* @throws IllegalArgumentException if pSelectionHolder or pComboBoxModel is null
*/
public ComboBoxModelAdapter(final ValueModel pSelectionHolder, final ComboBoxModel pComboBoxModel)
{
if (pSelectionHolder==null)
throw new IllegalArgumentException("selection holder must not be null");
if (pComboBoxModel==null)
throw new IllegalArgumentException("combobox model must not be null");
mSelectionHolder= pSelectionHolder;
mComboBoxModel = pComboBoxModel;
mInCallback = false;
mSelectionHolder.addChangeListener(this);
mComboBoxModel.addListDataListener(this);
adjustCombobox();
}
public void intervalAdded(final ListDataEvent pEvent)
{
handleComboboxModelChange();
}
public void intervalRemoved(final ListDataEvent pEvent)
{
handleComboboxModelChange();
}
public void contentsChanged(final ListDataEvent pEvent)
{
handleComboboxModelChange();
}
/**
* Common code of the combobox call back.
*/
private void handleComboboxModelChange()
{
if (mInCallback)
{
return;
}
Object selectedItem= mComboBoxModel.getSelectedItem();
Integer index = NO_SELECTION;
if (selectedItem!=null)
{
for (int i= 0, n= mComboBoxModel.getSize(); i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy