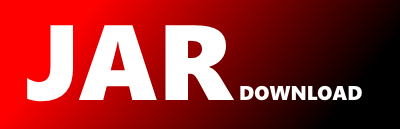
net.sf.cuf.model.ui.LOVCellEditor Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.ui;
import javax.swing.DefaultCellEditor;
import javax.swing.JComboBox;
import javax.swing.DefaultComboBoxModel;
import javax.swing.JTable;
import javax.swing.table.TableCellRenderer;
import java.util.List;
import java.util.EventObject;
import java.util.ArrayList;
import java.awt.event.MouseEvent;
import java.awt.event.ActionEvent;
import java.awt.Component;
/**
* This cell editor supports list-of-values mapping for a
* JComboBox, and is usefull if the domain object differs
* from the displayed item in the combobox.
* The class implements the TableCellRenderer interface so
* it can be also used for rendering.
* TODO: use isSelected and hasFocus for better rendering.
*/
public class LOVCellEditor extends DefaultCellEditor implements TableCellRenderer
{
/** our key list, never null */
private List> mKeys;
/** the combobox for our renderer personality */
private JComboBox mRenderer;
/**
* Constructs a LOVCellEditor
object that uses a
* combo box for display purpose and maps between the two lists.
* @param pKeys a list of keys, must not be null
* @param pDisplayedItems a list of displayed items, must not be null
*/
public LOVCellEditor(final List> pKeys, final List> pDisplayedItems)
{
super(new JComboBox());
// some error checking
if (pKeys==null) throw new IllegalArgumentException("Keys must not be null");
if (pDisplayedItems==null) throw new IllegalArgumentException("DisplayedItems must not be null");
if (pKeys.size()!=pDisplayedItems.size())
{
throw new IllegalArgumentException("size of Keys and DisplayedItems must match");
}
final JComboBox comboBox = (JComboBox) editorComponent;
// undo the DefaultCellEditor constructor delegate binding
comboBox.removeActionListener(delegate);
delegate= null;
// fill the combo boxes and remember our keys
comboBox.setModel(new DefaultComboBoxModel(pDisplayedItems.toArray()));
mRenderer= new JComboBox(pDisplayedItems.toArray());
mKeys= new ArrayList(pKeys);
// create a new delegate
delegate = new EditorDelegate()
{
public void setValue(final Object pValue)
{
int index= mKeys.indexOf(pValue);
comboBox.setSelectedIndex(index);
}
public Object getCellEditorValue()
{
int index= comboBox.getSelectedIndex();
if (index==-1)
return null;
else
return mKeys.get(index);
}
public boolean shouldSelectCell(final EventObject pEvent)
{
if (pEvent instanceof MouseEvent)
{
MouseEvent e = (MouseEvent) pEvent;
return e.getID() != MouseEvent.MOUSE_DRAGGED;
}
return true;
}
public boolean stopCellEditing()
{
if (comboBox.isEditable())
{
// Commit edited value.
comboBox.actionPerformed(new ActionEvent(LOVCellEditor.this, 0, ""));
}
return super.stopCellEditing();
}
};
// re-bind the combobox with the new delegate
comboBox.addActionListener(delegate);
}
public Component getTableCellRendererComponent(final JTable pTable, final Object pValue, final boolean pIsSelected, final boolean pHasFocus, final int pRow, final int pColumn)
{
int index= mKeys.indexOf(pValue);
mRenderer.setSelectedIndex(index);
return mRenderer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy