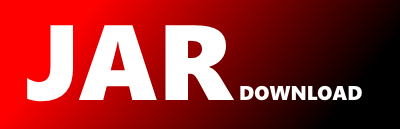
net.sf.cuf.model.ui.RadioButtonAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.ui;
import net.sf.cuf.model.ValueModel;
import javax.swing.ButtonModel;
import javax.swing.event.ChangeListener;
import javax.swing.event.ChangeEvent;
import java.util.List;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
/**
* A RadioButtonAdapter connects a number of radio button
* models to a ValueModel holding an Integer with the index
* for the radio button list.
* The ValueModel holding the Integer is often the selectionHolder()
* of a SelectionInList ValueModel.
* Whenever either the selection of the radio buttons or
* the index of the ValueModel changes, the other model
* is adjusted accordingly.
* A RadioButtonAdapter is not a ValueModel itself, but connects a
* ValueModel holding an integer to a list of ButtonModels.
*/
public class RadioButtonAdapter implements ActionListener, ChangeListener
{
/** the value model we are watching, never null */
private ValueModel mSelectionHolder;
/** the list of ButtonModels, never null */
private List mButtonModels;
/** marker if we are in an action performed or state changed callback */
private boolean mInCallback;
/**
* Creates a new adapter between a ValueModel holding an Integer
* and a list of ButtonModels
* Whenever one of the models changes, the other is adjusted accordingly.
* The initial selected buttonmodel is taken from the value model.
*
* @param pSelectionHolder ValueModel holding an Integer
* @param pButtonModels a list of ButtonModel objects
* @throws IllegalArgumentException if pSelectionHolder or pButtonModels is null
*/
public RadioButtonAdapter(final ValueModel pSelectionHolder, final List pButtonModels)
{
if (pSelectionHolder==null)
throw new IllegalArgumentException("selection holder must not be null");
if (pButtonModels==null)
throw new IllegalArgumentException("button model list must not be null");
mSelectionHolder= pSelectionHolder;
mButtonModels = pButtonModels;
mInCallback = false;
for (ButtonModel buttonModel : mButtonModels)
{
buttonModel.addActionListener(this);
}
mSelectionHolder.addChangeListener(this);
adjustRadiobuttons();
}
/**
* Invoked when an action occurs.
* @param pEvent a ActionEvent object
*/
public void actionPerformed(final ActionEvent pEvent)
{
if (mInCallback)
{
return;
}
ButtonModel model= (ButtonModel)pEvent.getSource();
Integer index= mButtonModels.indexOf(model);
try
{
mInCallback= true;
mSelectionHolder.setValue(index);
}
finally
{
mInCallback= false;
}
}
/**
* Invoked when the target of the listener has changed its state.
* @param pEvent a ChangeEvent object
*/
public void stateChanged(final ChangeEvent pEvent)
{
if (mInCallback)
{
return;
}
adjustRadiobuttons();
}
/**
* Helper to adjust the radio buttons to the index.
*/
private void adjustRadiobuttons()
{
Integer index= (Integer)mSelectionHolder.getValue();
try
{
mInCallback= true;
// null is the magic marker for "nothing selected"
if (index==null)
{
for (ButtonModel model : mButtonModels)
{
model.setSelected(false);
}
}
else
{
ButtonModel model= mButtonModels.get(index);
model.setSelected(true);
}
}
finally
{
mInCallback= false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy