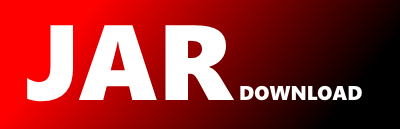
net.sf.cuf.model.ui.SpinnerModelAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.model.ui;
import net.sf.cuf.model.ValueModel;
import javax.swing.event.ChangeListener;
import javax.swing.event.ChangeEvent;
import javax.swing.SpinnerModel;
/**
* A SpinnerModelAdapter connects the value of a ValueModel to the
* value of a SpinnerModel. Whenever one of the model's changes,
* the other is adjusted accordingly.
* A SpinnerModelAdapter is itself not a ValueModel, but connects a
* ValueModel to a SpinnerModel.
*/
public class SpinnerModelAdapter implements ChangeListener
{
// the vallue model we adapt to, never null
private ValueModel mValueModel;
// the button model we adapt to, never null
private SpinnerModel mSpinnerModel;
// marker if we are in stateChange
private boolean mInStateChanged;
/**
* Creates a new adapter between a ValueModel and a SpinnerModel.
* Whenever one of the models changes, the other is adjusted accordingly.
* The initial selection state is taken from the value model.
* @param pValueModel the value model
* @param pSpinnerModel the spinner model
*/
public SpinnerModelAdapter(final ValueModel pValueModel, final SpinnerModel pSpinnerModel)
{
if (pValueModel==null)
throw new IllegalArgumentException("value model must not be null");
if (pSpinnerModel==null)
throw new IllegalArgumentException("spinner model must not be null");
mValueModel = pValueModel;
mSpinnerModel= pSpinnerModel;
mInStateChanged= false;
mSpinnerModel.addChangeListener(this);
mValueModel. addChangeListener(this);
}
/**
* Invoked when either the value model or the spinner model changed its state.
* @param pEvent a ChangeEvent object
*/
public void stateChanged(final ChangeEvent pEvent)
{
if (mInStateChanged)
{
return;
}
mInStateChanged= true;
try
{
Object source= pEvent.getSource();
if (source == mValueModel)
{
// ValueModel callback, update the SpinnerModel
mSpinnerModel.setValue(mValueModel.getValue());
}
else if (source== mSpinnerModel)
{
// SpinnerModel callback, update the ValueModel
mValueModel.setValue(mSpinnerModel.getValue());
}
else
{
// should never happen
throw new IllegalStateException("stateChanged with wrong source"+source);
}
}
finally
{
mInStateChanged= false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy