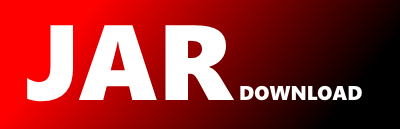
net.sf.cuf.state.AbstractStateAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state;
import java.lang.ref.WeakReference;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
/**
* This abstract implementation of StateAdapter handels all of the
* bookkeeping work and delegates the "real" work (adapting of a state
* change to a target object) to its subclasses.
*/
public abstract class AbstractStateAdapter implements StateAdapter, ChangeListener
{
/** our adaption targets, key= target object, value invert flag (TRUE or FALSE) */
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy