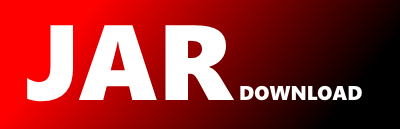
net.sf.cuf.state.SimpleState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state;
/**
* This class implements a MutableState and holds the necessary
* state information inside the SimpleState object.
*/
public class SimpleState extends AbstractState implements MutableState
{
/** Marker if we are in setEnabled(). */
protected boolean mInSetEnabled;
/**
* The default constructor creates an uninitialized state,
* which is not enabled. The state gets initialized as soon
* as setEnabled() is called.
*/
public SimpleState()
{
mInSetEnabled= false;
resetIntern();
}
/**
* Creates an initialized state.
* @param pIsEnabled true if our state should be enabled
*/
public SimpleState(final boolean pIsEnabled)
{
this();
setEnabled(pIsEnabled);
}
/**
* Sets the state.
* @param pEnabled true if the state gets enabled
*/
public void setEnabled(final boolean pEnabled)
{
setEnabled(pEnabled, null);
}
/**
* Sets the state with a reason object. The reason object
* may be null, and is returned to a getChangeReason() call
* during the callback.
* Only if the state change our listeners are notified.
* @param pEnabled true if the state gets enabled
* @param pReason null or reason for the change
*/
public void setEnabled(final boolean pEnabled, final Object pReason)
{
// check if there is work to be done
boolean changed= (!mIsInitialized) || (mIsEnabled!=pEnabled);
if (changed)
{
mIsInitialized= true;
mIsEnabled = pEnabled;
mReason = pReason;
mInSetEnabled = true;
try
{
fireStateChanged();
}
finally
{
mReason = null;
mInSetEnabled= false;
}
}
}
/**
* Marker method that indicates if the object is just firing a state change
* due to a call of setEnabled().
* This is useful for objects that are listeners to the State
* and are not interested in state changes triggered by themselfs
* calling setEnabled().
* @return true if we during a state change due to setEnabled(), false otherwise.
*/
protected boolean inSetEnabled()
{
return mInSetEnabled;
}
/**
* Resets a MutableState to not initilized. Calling this
* method will not trigger a notification of the
* listeners.
*/
public void reset()
{
resetIntern();
}
/**
* small helper that does the "real" reset, also called from
* the constructur.
*/
private void resetIntern()
{
mIsInitialized= false;
mIsEnabled = false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy