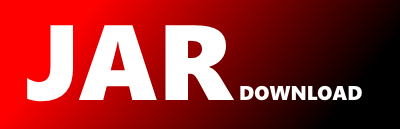
net.sf.cuf.state.StateExpression Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state;
/**
* The StateExpression interface allows to combine states to an
* arbitrary complex logical state expression.
* The expression is evaluated in the same order as it was build.
*/
public interface StateExpression extends State
{
/**
* Add a state to our current combined with a logical and expression.
* @param pState the state to add
* @throws IllegalArgumentException if pState is null
*/
void and(State pState);
/**
* Add the negation of a state to our current combined with a
* logical and expression.
* @param pState the state to add, the value of the state is inverted
* @throws IllegalArgumentException if pState is null
*/
void andNot(State pState);
/**
* Add a state to our current combined with a logical and expression.
* @param pState the state to add
* @param pInvert if true, invert the boolean state of pState
* @throws IllegalArgumentException if pState is null
*/
void and(State pState, boolean pInvert);
/**
* Add a state to our current combined with a logical or expression.
* @param pState the state to add
* @throws IllegalArgumentException if pState is null
*/
void or(State pState);
/**
* Add the negation of a state to our current combined with a
* logical or expression.
* @param pState the state to add, the value of the state is inverted
* @throws IllegalArgumentException if pState is null
*/
void orNot(State pState);
/**
* Add a state to our current combined with a logical or expression.
* @param pState the state to add
* @param pInvert if true, invert the boolean state of pState
* @throws IllegalArgumentException if pState is null
*/
void or(State pState, boolean pInvert);
/**
* Add a state to our current combined with a logical xor expression.
* @param pState the state to add
* @throws IllegalArgumentException if pState is null
*/
void xor(State pState);
/**
* Add the negation of a state to our current combined with a
* logical xor expression.
* @param pState the state to add, the value of the state is inverted
* @throws IllegalArgumentException if pState is null
*/
void xorNot(State pState);
/**
* Add a state to our current combined with a logical xor expression.
* @param pState the state to add
* @param pInvert if true, invert the boolean state of pState
* @throws IllegalArgumentException if pState is null
*/
void xor(State pState, boolean pInvert);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy