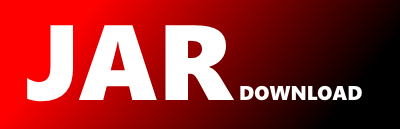
net.sf.cuf.state.ui.AbstractSwingState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.state.State;
import net.sf.cuf.state.AbstractState;
/**
* AbstractSwingState has the common boiler plate code for the various
* States in this package.
* To avoid a "broadcast storm" of firing AbstractSwingState's, a
* AbstractSwingState can be switched to "silent", in that case it
* ignores all changes.
*/
public abstract class AbstractSwingState extends AbstractState implements State
{
/** flag if we ignore all changes */
protected boolean mSilent;
/**
* The constructor initializes some member variables.
*/
public AbstractSwingState()
{
super();
mSilent = false;
mIsInitialized= true;
}
/**
* Checks the silent mode, returns true if we ignore a changing document.
* @return true if we are in silent mode
*/
public boolean isSilent()
{
return mSilent;
}
/**
* Switches silent mode on or off. Switching silent mode off may trigger
* an update if our current state is different from our document state.
* @param pSilent true if we should ignore changes
*/
public void setSilent(final boolean pSilent)
{
mSilent = pSilent;
if (!mSilent)
{
checkStateChange();
}
}
/**
* Check if our monitored state changed, if it did fireStateChanged().
*/
protected void checkStateChange()
{
if (mSilent)
{
return;
}
boolean selectionState= getInternalState();
if (mIsEnabled != selectionState)
{
mIsEnabled= selectionState;
setReason();
try
{
fireStateChanged();
}
finally
{
mReason= null;
}
}
}
/**
* Template method for the Strategy pattern: a derived class should
* return the state of its state abstraction.
* @return the current state
*/
protected abstract boolean getInternalState();
/**
* Template method for the Strategy pattern: a derived class should set
* mReason in this method.
*/
protected abstract void setReason();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy