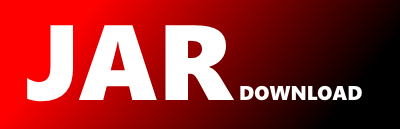
net.sf.cuf.state.ui.SwingBackgroundAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import java.awt.Color;
import java.util.HashMap;
import java.util.Map;
import javax.swing.JComponent;
import net.sf.cuf.state.AbstractStateAdapter;
import net.sf.cuf.state.State;
/**
* A SwingBackgroundAdapter adapts the "enabled" state to setting the
* original background color of a JComponent, the "disabled" state is mapped
* to setting the background Color supplied in the adapter's constructor
* or in the setDisabledBackground method.
*/
public class SwingBackgroundAdapter extends AbstractStateAdapter
{
/** the "disabled" background color, never null */
private Color mDisabledColor;
/** key= JComponent, value= original background Color, map is never null */
private Map mOriginalBackground;
/**
* Create a new adapter with a state and a default background Color.
* @param pState the state we adapt
*/
public SwingBackgroundAdapter(final State pState)
{
super(pState);
Color disabledColor= Color.PINK;
init(disabledColor);
}
/**
* Create a new adapter with no state associated.
* @param pDisabledColor the Color for the disabled state, must not be null
* @throws IllegalArgumentException if pDisabledColor is null
*/
public SwingBackgroundAdapter(final Color pDisabledColor)
{
super();
init(pDisabledColor);
}
/**
* Create a new adapter.
* @param pState the state we adapt
* @param pDisabledColor the Color for the disabled state, must not be null
* @throws IllegalArgumentException if pDisabledColor is null
*/
public SwingBackgroundAdapter(final State pState, final Color pDisabledColor)
{
super(pState);
init(pDisabledColor);
}
/**
* Small helper for the constructors.
* @param pDisabledColor the background Color for the disabled state, must not be null
*/
private void init(final Color pDisabledColor)
{
if (pDisabledColor==null)
{
throw new IllegalArgumentException("Color must not be null");
}
mOriginalBackground= new HashMap<>(1);
mDisabledColor= pDisabledColor;
}
/**
* Sets the disabled Color for the background, the new Color will be shown at the next
* state change.
* @param pDisabledColor the Color for the disabled state, must not be null
*/
public void setDisabledBackgound(final Color pDisabledColor)
{
if (pDisabledColor==null)
{
throw new IllegalArgumentException("Color must not be null");
}
mDisabledColor= pDisabledColor;
}
/**
* We store the original Color of the background of the component, and call processStateChange()
* afterwards.
* @param pTarget the target we should adjust
* @param pEnabled the state for the target
*/
protected void adjustInitialState(final Object pTarget, final boolean pEnabled)
{
if (pTarget instanceof JComponent)
{
JComponent component = (JComponent) pTarget;
mOriginalBackground.put(component, component.getBackground());
processStateChange(pTarget, pEnabled);
}
else
{
// you can add any objects but we won't handle them ;-)
throw new IllegalStateException("we cant handle that object:"+pTarget);
}
}
/**
* We map the pEnabled boolean to the setting/re-setting of a background Color
* on a JComponent.
* @param pTarget target object, must be a JComponent
* @param pEnabled true if the target object should get "enabled"
* @throws IllegalStateException if pTarget is not a JComponent
*/
protected void processStateChange(final Object pTarget, final boolean pEnabled)
{
if (pTarget instanceof JComponent)
{
JComponent component = (JComponent) pTarget;
if (pEnabled)
{
component.setBackground(mOriginalBackground.get(component));
}
else
{
component.setBackground(mDisabledColor);
}
}
else
{
// you can add any objects but we won't handle them ;-)
throw new IllegalStateException("we cant handle that object:"+pTarget);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy