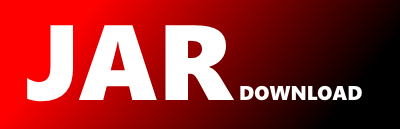
net.sf.cuf.state.ui.SwingDocumentState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.state.State;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import javax.swing.text.BadLocationException;
import javax.swing.text.Document;
import javax.swing.text.JTextComponent;
/**
* The SwingDocumentState class models a State with the Document of a
* Swing JTextField or JTextComponent as the state source. The value
* of the state is determined by comparing the Document's string with
* a user-defined string. If the string matches the document, the
* state is true.
* To avoid a "broadcast storm" of firing SwingDocumentState's, a
* SwingDocumentState can be switched to "silent", in that case it
* ignores all changes.
*/
public class SwingDocumentState extends AbstractSwingState implements State, DocumentListener
{
/** content we compare the model to, never null */
private String mContent;
/** the docment model we are watching, never null */
private Document mDocument;
/**
* Creates a new state, using the handed text field as its state source.
* If the content of the text field matches "" (= it is empty), then the
* state is true.
* @param pTextField text field we take the document from
*/
public SwingDocumentState(final JTextComponent pTextField)
{
this(pTextField, "");
}
/**
* Creates a new state, using the handed text field as its state source.
* If the content of the text field matches pContent, then the state is
* true
* @param pTextField text field we take the document from
* @param pContent string to compare to
*/
public SwingDocumentState(final JTextComponent pTextField, final String pContent)
{
super();
if (pTextField==null)
{
throw new IllegalArgumentException("textfield must not be null");
}
if (pContent==null)
{
throw new IllegalArgumentException("compare content must not be null");
}
mContent= pContent;
// initially set the state according to the document
mDocument= pTextField.getDocument();
mIsEnabled = getInternalState();
mName = pTextField.getName();
if (mName==null)
{
mName= "";
}
mDocument.addDocumentListener(this);
}
/**
* Sets the string we compare the document against.
* @param pContent string we compare our document to, must not be null
*/
public void setCompareContent(final String pContent)
{
if (pContent==null)
{
throw new IllegalArgumentException("compare content must not be null");
}
mContent= pContent;
checkStateChange();
}
/**
* Updates the compare content from the current value of the document.
*/
public void updateCompareFromDocument()
{
//noinspection EmptyCatchBlock
try
{
mContent= mDocument.getText( 0, mDocument.getLength());
}
catch (BadLocationException ignored) {}
checkStateChange();
}
/**
* Check the document state.
* @return true of our compare content matches the Document
*/
protected boolean getInternalState()
{
String documentContent= null;
//noinspection EmptyCatchBlock
try
{
documentContent= mDocument.getText(0, mDocument.getLength());
}
catch (BadLocationException ignored) {}
return mContent.equals(documentContent);
}
/**
* Set the source of the state as the reason for a change.
*/
protected void setReason()
{
mReason= mDocument;
}
/**
* Gives notification that there was an insert into the document. The
* range given by the DocumentEvent bounds the freshly inserted region.
*
* @param pEvent the document event
*/
public void insertUpdate(final DocumentEvent pEvent)
{
checkStateChange();
}
/**
* Gives notification that a portion of the document has been
* removed. The range is given in terms of what the view last
* saw (that is, before updating sticky positions).
*
* @param pEvent the document event
*/
public void removeUpdate(final DocumentEvent pEvent)
{
checkStateChange();
}
/**
* Gives notification that an attribute or set of attributes changed.
*
* @param pEvent the document event
*/
public void changedUpdate(final DocumentEvent pEvent)
{
checkStateChange();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy