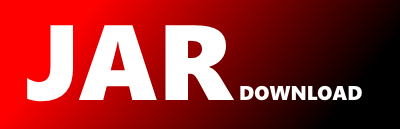
net.sf.cuf.state.ui.SwingEditableAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.state.AbstractStateAdapter;
import net.sf.cuf.state.State;
import javax.swing.JTextArea;
import javax.swing.UIManager;
import javax.swing.text.JTextComponent;
import java.awt.Color;
/**
* A SwingEditableAdapter maps the enabeling/disabeling of the monitored state
* to the editable/nonEditable of a JTextComponent.
* There is a special treatment for JTextArea: the background is set to
* the same backgroundcolor as a JTextField.
* FIXME: SwingEditableAdapter currently doesn't handle a Look&Feel change.
*/
public class SwingEditableAdapter extends AbstractStateAdapter
{
// shared background color of all handled widgets in the "true" case.
private Color mNonEditableFieldBackground;
// shared background color of all handled widgets in the "false" case.
private Color mEditableFieldBackground;
/**
* Creates a new adapter, that monitors no state and has an
* empty string as its name.
*/
public SwingEditableAdapter()
{
super();
initColors();
}
/**
* Creates a new adapter, that monitors the handed state and has an
* empty string as its name.
* @param pState the state we adopt to
* @throws IllegalArgumentException if pState is null
*/
public SwingEditableAdapter(final State pState)
{
super(pState);
initColors();
}
/**
* Init our enabled/disabled background colors.
*/
private void initColors()
{
mEditableFieldBackground = UIManager.getColor("TextField.background");
mNonEditableFieldBackground= UIManager.getColor("TextField.inactiveBackground");
}
/**
* We don't need special treatment for the inital step, and
* just call processStateChange().
* @param pTarget target object, never null
* @param pEnabled true if the target object should get "enabled"
*/
protected void adjustInitialState(final Object pTarget, final boolean pEnabled)
{
processStateChange(pTarget, pEnabled);
}
/**
* We map the pEnabled boolean to the editable/not editable behaviour
* of a JTextComponent.
* @param pTarget target object, never null
* @param pEnabled true if the target object should get "enabled"
*/
protected void processStateChange(final Object pTarget, final boolean pEnabled)
{
if (pTarget instanceof JTextComponent)
{
JTextComponent component = (JTextComponent) pTarget;
component.setEditable(pEnabled);
if (pTarget instanceof JTextArea)
{
JTextArea textArea= (JTextArea) pTarget;
if (pEnabled)
{
textArea.setBackground(mEditableFieldBackground);
}
else
{
textArea.setBackground(mNonEditableFieldBackground);
}
}
}
else
{
// you can add any objects but we won't handle them ;-)
throw new IllegalStateException("we cant handle that object:" + pTarget);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy