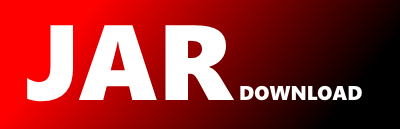
net.sf.cuf.state.ui.SwingEnabledAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.state.AbstractStateAdapter;
import net.sf.cuf.state.State;
import javax.swing.Action;
import javax.swing.JComponent;
import java.awt.Component;
import java.awt.Container;
/**
* A SwingEnabledAdapter maps the enabling/disabling of the monitored state
* to the enabling/disabling of a Component or Action.
* It also provides deep enable/disable capability and setting the JComponent (if it
* is one) to opaque or not.
*/
@SuppressWarnings("unused")
public class SwingEnabledAdapter extends AbstractStateAdapter
{
/** marker if we should enable/disable recursively */
private boolean mDeepEnable;
/** marker if we should also adjust the opaque property */
private boolean mHandleOpaque;
/**
* Create a new adapter with no state associated.
*/
public SwingEnabledAdapter()
{
this(null, false);
}
/**
* Create a new adapter.
* @param pState the state we adapt
*/
public SwingEnabledAdapter(final State pState)
{
this(pState, false);
}
/**
* Create a new adapter.
* @param pState the state we adapt
* @param pDeepEnable true if we should enable/disable recursively
*/
public SwingEnabledAdapter(final State pState, final boolean pDeepEnable)
{
this(pState, pDeepEnable, false);
}
/**
* Create a new adapter.
* @param pState the state we adapt
* @param pDeepEnable true if we should enable/disable recursively
* @param pHandleOpaque true if we should also seth the opaque property
*/
public SwingEnabledAdapter(final State pState,
final boolean pDeepEnable,
final boolean pHandleOpaque)
{
super(pState);
mDeepEnable= pDeepEnable;
mHandleOpaque= pHandleOpaque;
}
/**
* Sets if we should enable/disable recursively.
* @param pDeepEnable true if we should enable/disable recursively
*/
public void setDeepEnable(final boolean pDeepEnable)
{
boolean changed= (mDeepEnable!=pDeepEnable);
mDeepEnable = pDeepEnable;
if (changed)
{
stateChanged(null);
}
}
/**
* Returns if we enable/disable recursively.
* @return true if we enable/disable recursively
*/
public boolean isDeepEnable()
{
return mDeepEnable;
}
/**
* We don't need special treatment for the initial step, and
* just call processStateChange().
* @param pTarget the target we should adjust
* @param pEnabled the state for the target
*/
protected void adjustInitialState(final Object pTarget, final boolean pEnabled)
{
processStateChange(pTarget, pEnabled);
}
/**
* We map the pEnabled boolean to the enabled/not enabled behaviour of a
* AbstractButton or a Action.
* @param pTarget target object, either a Component or a Action
* @param pEnabled true if the target object should get "enabled"
* @throws IllegalStateException if pTarget is not a Component or Action
*/
protected void processStateChange(final Object pTarget, final boolean pEnabled)
{
if (pTarget instanceof Component)
{
Component component = (Component) pTarget;
setEnabled(component, pEnabled);
}
else if (pTarget instanceof Action)
{
Action action= (Action) pTarget;
action.setEnabled(pEnabled);
}
else
{
// you can add any objects but we won't handle them ;-)
throw new IllegalStateException("we cant handle that object:"+pTarget);
}
if (mHandleOpaque && pTarget instanceof JComponent)
{
((JComponent)pTarget).setOpaque(pEnabled);
}
}
/**
* Small helper to (recursively) enable/disable a component.
* @param pComponent the component
* @param pEnabled true if we should enable the component
*/
private void setEnabled(final Component pComponent, final boolean pEnabled)
{
if (mDeepEnable && (pComponent instanceof Container))
{
Component[] children= ((Container)pComponent).getComponents();
for (final Component child : children)
{
setEnabled(child, pEnabled);
}
}
pComponent.setEnabled(pEnabled);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy