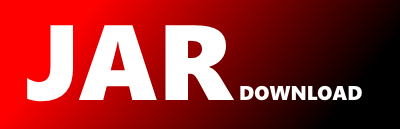
net.sf.cuf.state.ui.SwingEnabledState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.state.State;
import javax.swing.Action;
import javax.swing.JComponent;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
/**
* The SwingSelectedState class models a State with a Swing JComponent or
* Action as the state source.
*/
public class SwingEnabledState extends AbstractSwingState implements State, PropertyChangeListener
{
// null or the JComponent we use as enable/disable source
private JComponent mComponent;
// null or the Action we use as enable/disable source
private Action mAction;
// for the property change stuff
private static final String ENABLED_NAME = "enabled";
/**
* Creates a new initialized state with the Swing JComponent as its
* state backing store.
* @param pComponent the state source for this state
* @throws IllegalArgumentException if pComponent is null
*/
public SwingEnabledState(final JComponent pComponent)
{
super();
if (pComponent==null)
{
throw new IllegalArgumentException("component must not be null");
}
mAction = null;
mComponent= pComponent;
mName = mComponent.getName();
if (mName==null)
mName= "";
// initially set the state according to the document
mIsEnabled= getInternalState();
mComponent.addPropertyChangeListener(ENABLED_NAME, this);
}
/**
* Creates a new initialized state with the Swing Action as its
* state backing store.
* @param pAction the state source for this state
* @throws IllegalArgumentException if pAction is null
*/
public SwingEnabledState(final Action pAction)
{
super();
if (pAction==null)
{
throw new IllegalArgumentException("component must not be null");
}
mAction = pAction;
mComponent= null;
mName = "";
mAction.addPropertyChangeListener(this);
}
/**
* This method gets called when a bound property is changed.
* @param pEvent A PropertyChangeEvent object describing the event source
* and the property that has changed.
*/
public void propertyChange(final PropertyChangeEvent pEvent)
{
if (ENABLED_NAME.equals(pEvent.getPropertyName()))
{
checkStateChange();
}
}
/**
* Set the source of the state as the reason for a change.
*/
protected void setReason()
{
if (mComponent != null)
{
mReason= mComponent;
}
else
{
mReason= mAction;
}
}
/**
* Returns the enabled state of our component/action.
* @return true if our component or action is enabled
*/
protected boolean getInternalState()
{
if (mComponent != null)
{
return mComponent.isEnabled();
}
else
{
return mAction.isEnabled();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy