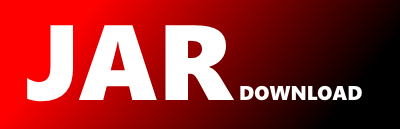
net.sf.cuf.state.ui.SwingGlassPaneAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.fw2.GlassPane;
import net.sf.cuf.state.AbstractStateAdapter;
import net.sf.cuf.state.State;
import javax.swing.RootPaneContainer;
import java.awt.Cursor;
import java.awt.Window;
import java.awt.Component;
/**
* The SwingGlassPaneAdapter maps the enabled/disabled of the observed state
* to the blocking/unblocking of a window using a glass pane.
* This works with targets that are both RootPaneContainer and Window,
* e.g. JFrame and JDialog.
*/
public class SwingGlassPaneAdapter extends AbstractStateAdapter
{
/** null or the last focus owner of the window before this glass pane was switched on. */
private Component mLastFocusOwner;
/** the cursor used in the window before this glass pane was switched on. */
private Cursor mLastCursor;
/**
* Create a new adapter with no state associated.
*/
public SwingGlassPaneAdapter()
{
super();
}
/**
* Create a new adapter.
* @param pState the state we adapt
*/
public SwingGlassPaneAdapter(final State pState)
{
super(pState);
}
/**
* Sets up the glass pane for the window.
* @param pTarget target object, never null
* @param pEnabled true if the target object should get "enabled"
*/
protected void adjustInitialState(final Object pTarget, final boolean pEnabled )
{
// set up the glass pane (we need a target that is a JRootPane and a Window)
if ((pTarget instanceof RootPaneContainer) && (pTarget instanceof Window))
{
RootPaneContainer target= (RootPaneContainer)pTarget;
if (!(target.getGlassPane() instanceof GlassPane))
{
target.setGlassPane(new GlassPane());
}
}
else
{
// you can add any objects but we won't handle them ;-)
throw new IllegalStateException("we cant handle that object:"+pTarget);
}
// now consider the state the normal way
processStateChange(pTarget, pEnabled);
}
/**
* Enables or disables the glasspane depending on the state.
* @param pTarget target object, never null
* @param pEnabled true if the target object should get "enabled"
*/
protected void processStateChange(final Object pTarget, final boolean pEnabled )
{
GlassPane glassPane= (GlassPane)((RootPaneContainer)pTarget).getGlassPane();
Window target = (Window)pTarget;
if (pEnabled && !glassPane.isVisible())
{
mLastFocusOwner= target.getFocusOwner();
mLastCursor = target.getCursor();
target.setCursor(Cursor.getPredefinedCursor(Cursor.WAIT_CURSOR));
glassPane.setVisible(true);
glassPane.requestFocus();
}
else if (!pEnabled && glassPane.isVisible())
{
if (mLastCursor!=null)
{
target.setCursor(mLastCursor);
}
glassPane.setVisible(false);
if (mLastFocusOwner != null)
{
mLastFocusOwner.requestFocus();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy