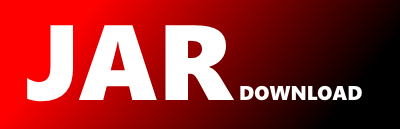
net.sf.cuf.state.ui.SwingJTableEnabledAdapter Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.state.AbstractStateAdapter;
import net.sf.cuf.state.State;
import javax.swing.JTable;
import javax.swing.UIManager;
/**
* A SwingJTableEnabledAdapter maps the enabeling/disabeling of the
* monitored state to the enabeling/disabeling of a JTable.
* Because the disabling of a JTable only disables the interaction,
* we use additonal code to signal the user that the table is
* disabled.
*/
public class SwingJTableEnabledAdapter extends AbstractStateAdapter
{
/**
* Create a new adapter with no state associated.
*/
public SwingJTableEnabledAdapter()
{
super();
}
/**
* Create a new adapter.
* @param pState the state we adapt
*/
public SwingJTableEnabledAdapter(final State pState)
{
super(pState);
}
/**
* We don't need special treatment for the inital step, and
* just call processStateChange().
* @param pTarget the target we should adjust
* @param pEnabled the state for the target
*/
protected void adjustInitialState(final Object pTarget, final boolean pEnabled)
{
processStateChange(pTarget, pEnabled);
}
/**
* We map the pEnabled boolean to the enabled/not enabled behaviour of a
* JTable by disabling the table, settting the foreground color to the
* disabled color of a combobox and setting the selection fore/background
* color to the disabled for/background color of a combobox.
* @param pTarget target object, either a JComponent or a Action
* @param pEnabled true if the target object should get "enabled"
* @throws IllegalStateException if pTarget is not a JComponent or Action
*/
protected void processStateChange(final Object pTarget, final boolean pEnabled)
{
if (pTarget instanceof JTable)
{
JTable table= (JTable) pTarget;
// "standard" enablement
table.setEnabled(pEnabled);
if (pEnabled)
{
// enable
table.setSelectionForeground(UIManager.getColor("Table.selectionForeground"));
table.setSelectionBackground(UIManager.getColor("Table.selectionBackground"));
table.setForeground (UIManager.getColor("Table.foreground"));
table.setBackground (UIManager.getColor("Table.background"));
}
else
{
// disable
table.setSelectionForeground(UIManager.getColor("ComboBox.disabledForeground"));
table.setSelectionBackground(UIManager.getColor("ComboBox.disabledBackground"));
table.setForeground (UIManager.getColor("ComboBox.disabledForeground"));
table.setBackground (UIManager.getColor("ComboBox.disabledBackground"));
}
}
else
{
// you can add any objects but we won't handle them ;-)
throw new IllegalStateException("we cant handle that object:"+pTarget);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy