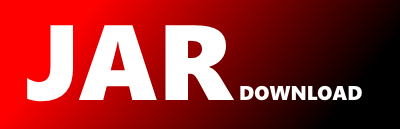
net.sf.cuf.state.ui.SwingListModelFillState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.state.State;
import javax.swing.JList;
import javax.swing.ListModel;
import javax.swing.event.ListDataEvent;
import javax.swing.event.ListDataListener;
/**
* The SwingListModelFillState class models a State with the ListModel
* of a Swing JList as the state source. The value of the state is
* determined by examining the ListModel's content.
* If the ListModel's content contains at least a provided number
* of elements (default: one element), the state is true.
*/
public class SwingListModelFillState extends AbstractSwingState implements State, ListDataListener
{
/** the selection we monitor, never null */
private ListModel mListModel;
/** the number of items we take as fill threshold */
private int mThreshold;
/**
* Creates a new state, using the model of the handed list as its state source.
* If the model contains at least one element, then the state is true.
* @param pList list we take the model from, must not be null
*/
public SwingListModelFillState(final JList pList)
{
this(pList, 1);
}
/**
* Creates a new state, using the model of the handed list as its state source.
* If the model contains at least pThreshold elements, then the state is true.
* @param pList list we take the model from, must not be null
* @param pThreshold threshold of items in the list
*/
public SwingListModelFillState(final JList pList, final int pThreshold)
{
super();
if (pList == null)
{
throw new IllegalArgumentException("JList must not be null");
}
init(pList.getModel(), pThreshold);
}
/**
* Creates a new state, using the handed model as its state source.
* If the model contains at least pThreshold elements, then the state is true.
* @param pThreshold threshold of items in the list
* @param pModel the model we monitor, must not be null
*/
public SwingListModelFillState(final ListModel pModel, final int pThreshold)
{
super();
init(pModel, pThreshold);
}
/**
* Common init stuff of the constructors.
* @param pModel the model we monitor, must not be null
* @param pThreshold threshold of items in the list
*/
private void init(final ListModel pModel, final int pThreshold)
{
if (pModel == null)
{
throw new IllegalArgumentException("list model must not be null");
}
mListModel= pModel;
mThreshold= pThreshold;
// initially set the state according to model content
mIsEnabled= getInternalState();
mListModel.addListDataListener(this);
}
/**
* Sets the threshold we compare again.
* @param pThreshold threshold of items in the list
*/
public void setCompareContent(final int pThreshold)
{
mThreshold = pThreshold;
checkStateChange();
}
/**
* Callback method for our list model.
* @param pEvent not used
*/
public void contentsChanged(final ListDataEvent pEvent)
{
checkStateChange();
}
/**
* Callback method for our list model.
* @param pEvent not used
*/
public void intervalAdded(final ListDataEvent pEvent)
{
checkStateChange();
}
/**
* Callback method for our list model.
* @param pEvent not used
*/
public void intervalRemoved(final ListDataEvent pEvent)
{
checkStateChange();
}
/**
* Check if our model contains at least our threshold number of items.
* @return the state, true if the model contains enough items
*/
protected boolean getInternalState()
{
return (mListModel.getSize() >= mThreshold);
}
/**
* Set the source of the state as the reason for a change.
*/
protected void setReason()
{
mReason = mListModel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy