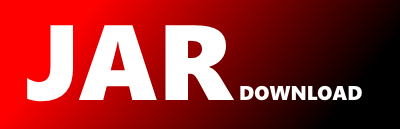
net.sf.cuf.state.ui.SwingTableModelFillState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.state.State;
import javax.swing.JTable;
import javax.swing.table.TableModel;
import javax.swing.event.TableModelListener;
import javax.swing.event.TableModelEvent;
/**
* The SwingTableModelFillState class models a State with the TableModel
* of a Swing JTable as the state source. The value of the state is
* determined by examining the TableModel's content.
* If the TableModel's content contains at least a provided number
* of rows (default: one element), the state is true.
*/
public class SwingTableModelFillState extends AbstractSwingState implements State, TableModelListener
{
/** the selection we monitor, never null */
private TableModel mTableModel;
/** the number of rows we take as fill threshold */
private int mThreshold;
/**
* Creates a new state, using the model of the handed table as its state source.
* If the model contains at least one row, then the state is true.
* @param pTable table we take the model from, must not be null
*/
public SwingTableModelFillState(final JTable pTable)
{
this(pTable, 1);
}
/**
* Creates a new state, using the model of the handed list as its state source.
* If the model contains at least pThreshold rows, then the state is true.
* @param pTable table we take the model from, must not be null
* @param pThreshold threshold of rows in the table
*/
public SwingTableModelFillState(final JTable pTable, final int pThreshold)
{
super();
if (pTable == null)
{
throw new IllegalArgumentException("JTable must not be null");
}
init(pTable.getModel(), pThreshold);
}
/**
* Creates a new state, using the handed model as its state source.
* If the model contains at least pThreshold rows, then the state is true.
* @param pThreshold threshold of rows in the table
* @param pModel the model we monitor, must not be null
*/
public SwingTableModelFillState(final TableModel pModel, final int pThreshold)
{
super();
init(pModel, pThreshold);
}
/**
* Common init stuff of the constructors.
* @param pModel the model we monitor, must not be null
* @param pThreshold threshold of rows in the table
*/
private void init(final TableModel pModel, final int pThreshold)
{
if (pModel == null)
{
throw new IllegalArgumentException("list model must not be null");
}
mTableModel= pModel;
mThreshold = pThreshold;
// initially set the state according to model content
mIsEnabled= getInternalState();
mTableModel.addTableModelListener(this);
}
/**
* Sets the threshold we compare again.
* @param pThreshold threshold of rows in the table
*/
public void setCompareContent(final int pThreshold)
{
mThreshold = pThreshold;
checkStateChange();
}
/**
* Callback method for our table model.
* @param pEvent not used
*/
public void tableChanged(final TableModelEvent pEvent)
{
checkStateChange();
}
/**
* Check if our table model contains at least our threshold number of rows.
* @return the state, true if the table model contains enough rows
*/
protected boolean getInternalState()
{
return (mTableModel.getRowCount() >= mThreshold);
}
/**
* Set the source of the state as the reason for a change.
*/
protected void setReason()
{
mReason = mTableModel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy