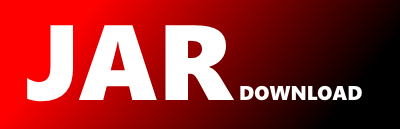
net.sf.cuf.state.ui.SwingTreeModelFillState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import net.sf.cuf.state.State;
import javax.swing.JTree;
import javax.swing.event.TreeModelEvent;
import javax.swing.event.TreeModelListener;
import javax.swing.tree.TreeModel;
/**
* The SwingTreeModelFillState class models a State with the TreeModel of a
* Jtree as the state source. The value of the state is determined by
* examining the TreeModels's content.
* If the TreeModel's content contains at least a provided number
* of elements (default: one element), the state is true.
*/
public class SwingTreeModelFillState extends AbstractSwingState implements State, TreeModelListener
{
/** the selection we monitor, never null */
private TreeModel mTreeModel;
/** indicates whether the root-node is counted as content or not */
private boolean mCountRootAsContent;
/** the number of items we take as fill threshold */
private int mThreshold;
/**
* Creates a new state, using the model of the handed tree as its state source.
* If the model contains at least one element (not counting the root element
* as element), then the state is true.
* @param pTree tree we take the model from, must not be null
*/
public SwingTreeModelFillState(final JTree pTree)
{
this(pTree, false);
}
/**
* Creates a new state, using the model of the handed tree as its state source.
* If the model contains at least one element, then the state is true.
* @param pTree tree we take the model from, must not be null
* @param pCountRootAsContent indicates whether the root-node is counted as
* content or not
*/
public SwingTreeModelFillState(final JTree pTree, final boolean pCountRootAsContent)
{
super();
if (pTree== null)
{
throw new IllegalArgumentException("JTree must not be null");
}
init(pTree.getModel(), pCountRootAsContent, 1);
}
/**
* Creates a new state, using the handed model as its state source.
* If the model contains any data, then the
* state is true.
* @param pModel the model we monitor, must not be null
* @param pCountRootAsContent indicates whether the root-node is counted as
* content or not
* @param pThreshold threshold of items in the tree
*/
public SwingTreeModelFillState(final TreeModel pModel, final boolean pCountRootAsContent, final int pThreshold)
{
super();
init(pModel, pCountRootAsContent, pThreshold);
}
/**
* Common init stuff of the constructors.
* @param pModel the model we monitor, must not be null
* @param pCountRootAsContent indicates whether the root-node is counted as
* content or not
* @param pThreshold threshold of items in the tree
*/
private void init(final TreeModel pModel, final boolean pCountRootAsContent, final int pThreshold)
{
if (pModel == null)
{
throw new IllegalArgumentException("tree model must not be null");
}
mCountRootAsContent= pCountRootAsContent;
mTreeModel = pModel;
mThreshold = pThreshold;
// initially set the state according to selection model
mIsEnabled= getInternalState();
mTreeModel.addTreeModelListener(this);
}
/**
* Sets the threshold we compare again.
* @param pThreshold threshold of items in the tree
*/
public void setCompareContent(final int pThreshold)
{
mThreshold = pThreshold;
checkStateChange();
}
/**
* Callback method for our tree model.
* @param pEvent not used
*/
public void treeNodesChanged(final TreeModelEvent pEvent)
{
checkStateChange();
}
/**
* Callback method for our tree model.
* @param pEvent not used
*/
public void treeNodesInserted(final TreeModelEvent pEvent)
{
checkStateChange();
}
/**
* Callback method for our tree model.
* @param pEvent not used
*/
public void treeNodesRemoved(final TreeModelEvent pEvent)
{
checkStateChange();
}
/**
* Callback method for our tree model.
* @param pEvent not used
*/
public void treeStructureChanged(final TreeModelEvent pEvent)
{
checkStateChange();
}
/**
* Check if our model contains at least our threshold number of items.
* @return the state, true if the model contains enough items
*/
protected boolean getInternalState()
{
Object root= mTreeModel.getRoot();
if (mCountRootAsContent)
{
if (root == null)
{
return (0 >= mThreshold);
}
else
{
return ((1+mTreeModel.getChildCount(root)) >= mThreshold);
}
}
else
{
return (mTreeModel.getChildCount(root) >= mThreshold);
}
}
/**
* Set the source of the state as the reason for a change.
*/
protected void setReason()
{
mReason = mTreeModel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy