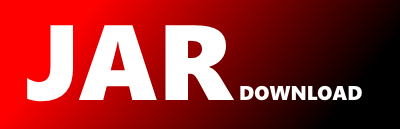
net.sf.cuf.state.ui.SwingTreeSelectionState Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.state.ui;
import javax.swing.JTree;
import javax.swing.event.TreeSelectionEvent;
import javax.swing.event.TreeSelectionListener;
import javax.swing.tree.TreePath;
import javax.swing.tree.TreeSelectionModel;
/**
* The SwingTreeSelectionState class models a State with a TreeSelectionModel
* of a JTree as the state source. The value of the state is determined by
* comparing the TreeSelectionsModels's selection with a user-defined TreePath.
* If the user-defined TreePath is contained in the TreeSelectionModel's
* selection, the state is true.
*/
public class SwingTreeSelectionState extends AbstractSwingState implements TreeSelectionListener
{
/** the selection we monitor, never null */
private TreeSelectionModel mTreeSelectionModel;
/** user provided path we check, default is null */
private TreePath mTreePath;
/**
* Creates a new state, using the selection model of the handed tree
* as its state source.
* If the selection contains null, then the
* state is true.
* @param pTree tree we take the selection model from, must not be null
*/
public SwingTreeSelectionState(final JTree pTree)
{
this(pTree, null);
}
/**
* Creates a new state, using the selection model of the handed tree
* as its state source.
* If the selection contains pTreePath, then the state is true.
* @param pTree tree we take the selection model from, must not be null
* @param pTreePath user-provided TreePath we check the selection against, may be null
*/
public SwingTreeSelectionState(final JTree pTree, final TreePath pTreePath)
{
super();
if (pTree== null)
{
throw new IllegalArgumentException("JTree must not be null");
}
init(pTree.getSelectionModel(), pTreePath);
}
/**
* Creates a new state, using the handed selection model as its state source.
* If the selection contains pTreePath, then the state is true.
* @param pSelectionModel the selection we monitor, must not be null
* @param pTreePath user-provided TreePath we check the selection against, may be null
*/
public SwingTreeSelectionState(final TreeSelectionModel pSelectionModel, final TreePath pTreePath)
{
super();
init(pSelectionModel, pTreePath);
}
/**
* Common init stuff of the constructors.
* @param pSelectionModel the selection we monitor, must not be null
* @param pTreePath TreePath we check the selection against, may be null
*/
private void init(final TreeSelectionModel pSelectionModel, final TreePath pTreePath)
{
if (pSelectionModel == null)
{
throw new IllegalArgumentException("tree selection model must not be null");
}
mTreePath = pTreePath;
mTreeSelectionModel= pSelectionModel;
// initially set the state according to selection model
mIsEnabled = getInternalState();
mTreeSelectionModel.addTreeSelectionListener(this);
}
/**
* Sets the TreePath we try to find in the tree selection.
* @param pTreePath user-provided TreePath we check the selection against
*/
public void setCompareContent(final TreePath pTreePath)
{
mTreePath = pTreePath;
checkStateChange();
}
/**
* Called whenever the value of the selection changes.
* @param pEvent the event that characterizes the change.
*/
public void valueChanged(final TreeSelectionEvent pEvent)
{
checkStateChange();
}
/**
* Check if mListIndex is selected, if mListIndex is NO_SELECTION, check if there is no selection.
* @return the state, true if the user provided index is contained in the list selection
*/
protected boolean getInternalState()
{
if ((mTreePath == null) && mTreeSelectionModel.isSelectionEmpty())
{
return true;
}
else
{
return mTreeSelectionModel.isPathSelected(mTreePath);
}
}
/**
* Set the source of the state as the reason for a change.
*/
protected void setReason()
{
mReason= mTreeSelectionModel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy