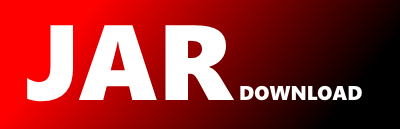
net.sf.cuf.ui.AutoCompletion Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.ui;
import javax.swing.ComboBoxEditor;
import javax.swing.ComboBoxModel;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.text.AttributeSet;
import javax.swing.text.BadLocationException;
import javax.swing.text.JTextComponent;
import javax.swing.text.PlainDocument;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.FocusAdapter;
import java.awt.event.FocusEvent;
import java.awt.event.FocusListener;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
/**
* This class helps for auto-completion of a JComboBox. It is in the
* public domain and taken from http://www.orbital-computer.de/JComboBox/.
*/
public class AutoCompletion extends PlainDocument
{
private JComboBox mComboBox;
private ComboBoxModel mModel;
private JTextComponent mEditor;
// flag to indicate if setSelectedItem has been called
// subsequent calls to remove/insertString should be ignored
private boolean mSelecting;
private boolean mHidePopupOnFocusLoss;
private boolean mHitBackspace;
private boolean mHitBackspaceOnSelection;
private KeyListener mEditorKeyListener;
private FocusListener mEditorFocusListener;
public AutoCompletion(final JComboBox pComboBox)
{
mComboBox= pComboBox;
mModel = pComboBox.getModel();
pComboBox.addActionListener(pEvent -> {
if (!mSelecting) highlightCompletedText(0);
});
pComboBox.addPropertyChangeListener(pEvent -> {
if ("editor".equals(pEvent.getPropertyName())) configureEditor((ComboBoxEditor) pEvent.getNewValue());
if ("model".equals(pEvent.getPropertyName())) mModel = (ComboBoxModel) pEvent.getNewValue();
});
mEditorKeyListener = new KeyAdapter()
{
public void keyPressed(final KeyEvent pEvent)
{
if (pComboBox.isDisplayable()) pComboBox.setPopupVisible(true);
mHitBackspace = false;
switch (pEvent.getKeyCode())
{
// determine if the pressed key is backspace (needed by the remove method)
case KeyEvent.VK_BACK_SPACE:
mHitBackspace = true;
mHitBackspaceOnSelection = mEditor.getSelectionStart() != mEditor.getSelectionEnd();
break;
// ignore delete key
case KeyEvent.VK_DELETE:
pEvent.consume();
pComboBox.getToolkit().beep();
break;
}
}
};
// Bug 5100422 on Java 1.5: Editable JComboBox won't hide popup when tabbing out
mHidePopupOnFocusLoss = System.getProperty("java.version").startsWith("1.5");
// Highlight whole text when gaining focus
mEditorFocusListener = new FocusAdapter()
{
public void focusGained(final FocusEvent pEvent)
{
highlightCompletedText(0);
}
public void focusLost(final FocusEvent pEvent)
{
// Workaround for Bug 5100422 - Hide Popup on focus loss
if (mHidePopupOnFocusLoss) pComboBox.setPopupVisible(false);
}
};
configureEditor(pComboBox.getEditor());
// Handle initially selected object
Object selected = pComboBox.getSelectedItem();
if (selected != null) setText(selected.toString());
highlightCompletedText(0);
}
public static void enable(final JComboBox pComboBox)
{
// has to be editable
pComboBox.setEditable(true);
// change the editor's document
new AutoCompletion(pComboBox);
}
void configureEditor(final ComboBoxEditor pNewEditor)
{
if (mEditor != null)
{
mEditor.removeKeyListener(mEditorKeyListener);
mEditor.removeFocusListener(mEditorFocusListener);
}
if (pNewEditor != null)
{
mEditor = (JTextComponent) pNewEditor.getEditorComponent();
mEditor.addKeyListener(mEditorKeyListener);
mEditor.addFocusListener(mEditorFocusListener);
mEditor.setDocument(this);
}
}
public void remove(int pOffs, final int pLen) throws BadLocationException
{
// return immediately when selecting an item
if (mSelecting) return;
if (mHitBackspace)
{
// user hit backspace => move the selection backwards
// old item keeps being selected
if (pOffs > 0)
{
if (mHitBackspaceOnSelection) pOffs--;
}
else
{
// User hit backspace with the cursor positioned on the start => beep
mComboBox.getToolkit().beep(); // when available use: UIManager.getLookAndFeel().provideErrorFeedback(mComboBox);
}
highlightCompletedText(pOffs);
}
else
{
super.remove(pOffs, pLen);
}
}
public void insertString(int pOffs, final String pStr, final AttributeSet pAttributeSet) throws BadLocationException
{
// return immediately when selecting an item
if (mSelecting) return;
// insert the string into the document
super.insertString(pOffs, pStr, pAttributeSet);
// lookup and select a matching item
Object item = lookupItem(getText(0, getLength()));
if (item != null)
{
setSelectedItem(item);
}
else
{
// keep old item selected if there is no match
item = mComboBox.getSelectedItem();
// imitate no insert (later on pOffs will be incremented by pStr.length(): selection won't move forward)
pOffs = pOffs - pStr.length();
// provide feedback to the user that his input has been received but can not be accepted
mComboBox.getToolkit().beep(); // when available use: UIManager.getLookAndFeel().provideErrorFeedback(mComboBox);
}
setText(item.toString());
// select the completed part
highlightCompletedText(pOffs + pStr.length());
}
private void setText(final String pText)
{
try
{
// remove all text and insert the completed string
super.remove(0, getLength());
super.insertString(0, pText, null);
}
catch (BadLocationException e)
{
throw new RuntimeException(e.toString());
}
}
private void highlightCompletedText(final int pStart)
{
mEditor.setCaretPosition(getLength());
mEditor.moveCaretPosition(pStart);
}
private void setSelectedItem(final Object pItem)
{
mSelecting = true;
mModel.setSelectedItem(pItem);
mSelecting = false;
}
private Object lookupItem(final String pPattern)
{
Object selectedItem = mModel.getSelectedItem();
// only search for a different item if the currently selected does not match
if (selectedItem != null && startsWithIgnoreCase(selectedItem.toString(), pPattern))
{
return selectedItem;
}
else
{
// iterate over all items
for (int i = 0, n = mModel.getSize(); i < n; i++)
{
Object currentItem = mModel.getElementAt(i);
// current item starts with the pattern?
if (currentItem != null && startsWithIgnoreCase(currentItem.toString(), pPattern))
{
return currentItem;
}
}
}
// no item starts with the pattern => return null
return null;
}
// checks if pStr1 starts with pStr2 - ignores case
private boolean startsWithIgnoreCase(final String pStr1, final String pStr2)
{
return pStr1.toUpperCase().startsWith(pStr2.toUpperCase());
}
private static void createAndShowGUI()
{
// the combo box (add/modify items if you like to)
final JComboBox comboBox = new JComboBox(new Object[]{"Ester", "Jordi", "Jordina", "Jorge", "Sergi"});
enable(comboBox);
// create and show a window containing the combo box
final JFrame frame = new JFrame();
frame.setDefaultCloseOperation(3);
frame.getContentPane().add(comboBox);
frame.pack();
frame.setVisible(true);
}
public static void main(final String[] pArgs)
{
javax.swing.SwingUtilities.invokeLater(AutoCompletion::createAndShowGUI);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy