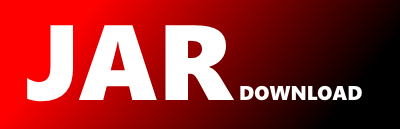
net.sf.cuf.ui.FlexibleGridLayout Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.ui;
import java.awt.*;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import com.jgoodies.forms.layout.FormLayout;
import com.jgoodies.forms.builder.DefaultFormBuilder;
/**
* ToDo: Kommentieren Wichtig: Immer eine Instanz eigene Instanz pro Container!
*/
public class FlexibleGridLayout implements LayoutManager2
{
private static final String DEFAULT_DATA_COLSPEC = "fill:pref:grow";
private static final String DEFAULT_COL_GAP = "10dlu";
public static final String LINE_BREAK = "LINE_BREAK";
public static final String NO_LINE_BREAK = "NO_LINE_BREAK"; // default
private DefaultFormBuilder mBuilder;
private boolean mAutoColumnBreakMode = false;
public FlexibleGridLayout(final JPanel panel)
{
init(panel);
}
private void init(final JPanel panel)
{
mBuilder = new DefaultFormBuilder(new FormLayout("", ""), panel);
}
public void setDefaultBreak(final int pDefaultBreak)
{
// TODO: temporär wird die Erzeugung des FormLayouts einfach hier
// gemacht,
// weil Methode nach "init" aufgerufen wird.
StringBuilder columnBuffer = new StringBuilder();
for (int i = 0; i < pDefaultBreak; i++)
{
String prefix = (i == 0) ? "" : ",";
columnBuffer.append(prefix).append(DEFAULT_DATA_COLSPEC + ", " + DEFAULT_COL_GAP);
}
// TODO: Sonderfall, falls Columns auf "0" gesetzt wird: Mindestens
// eine Spalte hinzuf�gen
if (pDefaultBreak == 0)
{
columnBuffer.append(DEFAULT_DATA_COLSPEC);
}
mBuilder = new DefaultFormBuilder(new FormLayout(columnBuffer.toString(), ""),
mBuilder.getPanel());
mAutoColumnBreakMode = true;
}
/**
* If the layout manager uses a per-component string, adds the component
* comp
to the layout, associating it with the string
* specified by name
.
*
* @param pName
* the string to be associated with the component
* @param pComponent
* the component to be added
*/
public void addLayoutComponent(final String pName, final Component pComponent)
{
addLayoutComponent(pComponent, pName);
}
/**
* Adds the specified component to the layout, using the specified
* constraint object.
*
* @param pComponent
* the component to be added
* @param pConstraints
* where/how the component is added to the layout.
*/
public void addLayoutComponent(final Component pComponent, final Object pConstraints)
{
if (pConstraints == null)
{
// Wenn die Zeilen nicht automatisch umgebrochen werden sollen,
// zusichern, dass f�r die neu hinzuzuf�gende Komponente ausreichend
// Spalten vorhanden sind.
if (!mAutoColumnBreakMode)
{
ensureEnoughColumnsForNewComp();
}
mBuilder.append(pComponent);
}
else if (NO_LINE_BREAK.equals(pConstraints))
{
// Wenn die Zeilen nicht automatisch umgebrochen werden sollen,
// zusichern, dass f�r die neu hinzuzuf�gende Komponente ausreichend
// Spalten vorhanden sind.
if (!mAutoColumnBreakMode)
{
ensureEnoughColumnsForNewComp();
}
mBuilder.append(pComponent);
}
else if (LINE_BREAK.equals(pConstraints))
{
// Wenn Zeile vor Spaltenende umgebrochen wird, Zeile bis zum
// Spaltenende
// ausdehnen...
if (mBuilder.getColumn() < mBuilder.getColumnCount())
{
mBuilder.append(pComponent, (mBuilder.getColumnCount() - mBuilder
.getColumn()));
}
else
{
// Hier m�ssen wir grunds�tzlich sicher stellen, dass gen�gend
// Spalten vorhanden sind, weil f�r mit LineBreak nacheinander
// hinzugef�gte Kopmonenten in der Regel keine Spaltenanzahl
// definiert ist...
ensureEnoughColumnsForNewComp();
// Zeile hinzuf�gen...
mBuilder.append(pComponent);
}
mBuilder.nextLine();
}
else
{
if (pComponent instanceof JScrollPane
|| (pComponent instanceof JPanel && containsScrollPane((JPanel) pComponent)))
{
// TODO: Feature wieder einbauen, wenn in JGoodies wieder vorhanden
//mBuilder.getLayout().getRowSpec(mBuilder.getRow()).setResizeWeight(1.0);
}
mBuilder.getLayout().addLayoutComponent(pComponent, pConstraints);
}
}
/**
* Checks if the handed panel contains a scroll pane.
* @param panel the panel to check
* @return true if the panel contains a scroll pane
*/
private boolean containsScrollPane(final JPanel panel)
{
Component[] components = panel.getComponents();
for (final Component component : components)
{
if (component instanceof JScrollPane)
return true;
}
return false;
}
/**
* Makes sure that there are enough columns in the layout for a new
* component to add. If there are not enough columns, two new columns are
* added: One for the new column, and one for the default column gap.
*/
private void ensureEnoughColumnsForNewComp()
{
if (mBuilder.getColumn() > mBuilder.getColumnCount())
{
mBuilder.appendColumn(DEFAULT_DATA_COLSPEC);
mBuilder.appendColumn(DEFAULT_COL_GAP);
}
}
/**
* Removes the specified component from the layout.
*
* @param pComponent
* the component to be removed
*/
public void removeLayoutComponent(final Component pComponent)
{
mBuilder.getLayout().removeLayoutComponent(pComponent);
}
/**
* Lays out the specified container.
*
* @param parent
* the container to be laid out
*/
public void layoutContainer(final Container parent)
{
mBuilder.getLayout().layoutContainer(parent);
}
/**
* Calculates the minimum size dimensions for the specified container, given
* the components it contains.
*
* @param parent
* the component to be laid out
* @see #preferredLayoutSize(Container)
*/
public Dimension minimumLayoutSize(final Container parent)
{
return mBuilder.getLayout().minimumLayoutSize(parent);
}
/**
* Calculates the maximum size dimensions for the specified container, given
* the components it contains.
*
* @see Component#getMaximumSize
* @see LayoutManager
*/
public Dimension maximumLayoutSize(final Container parent)
{
return mBuilder.getLayout().maximumLayoutSize(parent);
}
/**
* Calculates the preferred size dimensions for the specified container,
* given the components it contains.
*
* @param pParent
* the container to be laid out
*
* @see #minimumLayoutSize(java.awt.Container)
*/
public Dimension preferredLayoutSize(final Container pParent)
{
return mBuilder.getLayout().preferredLayoutSize(pParent);
}
/**
* Invalidates the layout, indicating that if the layout manager has cached
* information it should be discarded.
*/
public void invalidateLayout(final Container pTarget)
{
mBuilder.getLayout().invalidateLayout(pTarget);
}
/**
* Returns the alignment along the x axis. This specifies how the component
* would like to be aligned relative to other components. The value should
* be a number between 0 and 1 where 0 represents alignment along the
* origin, 1 is aligned the furthest away from the origin, 0.5 is centered,
* etc.
*/
public float getLayoutAlignmentX(final Container pTarget)
{
return mBuilder.getLayout().getLayoutAlignmentX(pTarget);
}
/**
* Returns the alignment along the y axis. This specifies how the component
* would like to be aligned relative to other components. The value should
* be a number between 0 and 1 where 0 represents alignment along the
* origin, 1 is aligned the furthest away from the origin, 0.5 is centered,
* etc.
*/
public float getLayoutAlignmentY(final Container pTarget)
{
return mBuilder.getLayout().getLayoutAlignmentY(pTarget);
}
public void setVgap(final int pDluGapSize)
{
// TODO JZE mBuilder.setLineGapSize(Sizes.dluY(pDluGapSize));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy