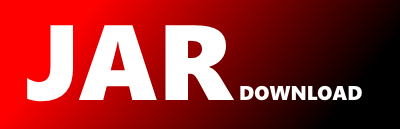
net.sf.cuf.ui.TableLayout2 Maven / Gradle / Ivy
The newest version!
package net.sf.cuf.ui;
import info.clearthought.layout.TableLayout;
import java.awt.Component;
import java.util.Map;
import java.util.HashMap;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* TableLayout2 adds symbolic column and row name support to TableLayout.
*/
public class TableLayout2 extends TableLayout
{
/** our regexp for the symbolic names */
protected static final Pattern PATTERN = Pattern.compile("(.+)\\s*\\((\\w+)\\)");
/** key= symbolic name as String, value= row or column index as String, both have a , at the end */
protected Map mSymbolicCR;
/** Symbolic name for TableLayout.FILL */
public static final String FILLTEXT = "fill";
/** Symbolic name for TableLayout.PREFERRED */
public static final String PREFERREDTEXT= "preferred";
/** Symbolic name for TableLayout.MINIMUM */
public static final String MINIMUMTEXT = "minimum";
/**
* Constructs an instance of TableLayout2. This TableLayout2 will
* have one row and one column.
*/
public TableLayout2()
{
super();
mSymbolicCR= new HashMap<>();
}
/**
* Constructs an instance of TableLayout.
*
* @param pColumns comma seperated columns description, a column may
* have a symbolic name attached in braces
* @param pRows comma seperated rows description, a row may
* have a symbolic name attached in braces
*/
public TableLayout2(final String pColumns, final String pRows)
{
this();
double[] columns= getCR(pColumns);
double[] rows = getCR(pRows);
setColumn(columns);
setRow (rows);
}
/**
* Small helper to extract a double[] from a string, symbolic names are detected
* and stored in our hashmap.
* @param pCR column or rows as string
* @return array of doubles
* @throws IllegalArgumentException if we find a symbolic name more than one time
*/
private double[] getCR(final String pCR)
{
Matcher m= PATTERN.matcher("");
String[] crsString= pCR.trim().split("\\s*,\\s*");
double[] crs = new double[crsString.length];
for (int i = 0; i < crsString.length; i++)
{
String crString= crsString[i];
m.reset(crString);
if (m.matches())
{
crString = m.group(1);
String symbolicName= m.group(2);
Integer index = i;
if (mSymbolicCR.containsKey(symbolicName))
{
throw new IllegalArgumentException("symbolic name "+
symbolicName+
" not uniq");
}
// Add to every symbolic name and index a comma to use comma as a separator
mSymbolicCR.put(symbolicName.concat(","), index.toString().concat(","));
}
if (crString.equalsIgnoreCase(FILLTEXT))
{
crs[i]= TableLayout.FILL;
}
else if (crString.equalsIgnoreCase(PREFERREDTEXT))
{
crs[i]= TableLayout.PREFERRED;
}
else if (crString.equalsIgnoreCase(MINIMUMTEXT))
{
crs[i]= TableLayout.MINIMUM;
}
else
{
crs[i]= Double.parseDouble(crString);
}
}
return crs;
}
/**
* Adds the specified component with the specified constraint to the layout.
* If the constraint is a string, it may be a symbolic name that was defined
* in the TableLayout2 constructor.
*
* @param pComponent component to add
* @param pConstraint indicates entry's position and alignment
*/
public void addLayoutComponent(final Component pComponent, final Object pConstraint)
{
Object constraint= pConstraint;
if (pConstraint instanceof String)
{
// replace all symbolic names
String constraintString = ((String)pConstraint);
// add a comma at the end as a separator, but don't forget to remove it later
constraintString = constraintString.concat(",");
// remove all spaces
constraintString = constraintString.replaceAll("\\s", "");
for (Map.Entry stringStringEntry : mSymbolicCR.entrySet())
{
Map.Entry entry = (Map.Entry) stringStringEntry;
constraintString = constraintString.replaceAll((String) entry.getKey(),
entry.getValue().toString());
}
// remove the the last comma
if (constraintString.endsWith(","))
{
constraint = constraintString.substring(0, constraintString.length() - 1);
}
}
super.addLayoutComponent(pComponent, constraint);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy