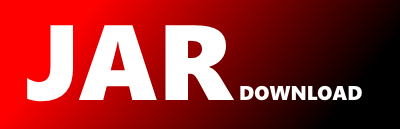
net.sf.cuf.ui.table.ContextMenuAction Maven / Gradle / Ivy
package net.sf.cuf.ui.table;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.SwingUtilities;
/**
* Base functionality for an entry in a {@link TableContextMenu}.
*
* This implementation delays the execution of the context menu action with {@link SwingUtilities#invokeLater(Runnable)}
* in order to avoid redraw problems when the popup menu is closed.
*
* @author Hendrik Wördehoff, sd&m AG
*/
public abstract class ContextMenuAction
implements ActionListener, Runnable
{
/**
* With this reference we can get information about the table we work on.
* The information is only guaranteed to be vaild while we are called by {@link TableContextMenu}.
*/
protected ContextMenuAdapter mAdapter = null;
/**
* This gets call by the {@link TableContextMenu} to give us access to information about the table we work on.
* @param pAdapter table information source
*/
public void initialize(final ContextMenuAdapter pAdapter)
{
if ( pAdapter == null )
throw new IllegalArgumentException("adapter must not be null");
if ( mAdapter != null )
throw new IllegalStateException("initialize must be called exactly once");
mAdapter = pAdapter;
}
/**
* Implementation of the {@link ActionListener} interface.
* We do not react on this event immediately. Instead we use {@link SwingUtilities#invokeLater(Runnable)}
* to complete the event processing before we take further steps in {@link #run}.
* This eliminates redraw problems in Swing.
* @param pEvent action event
*/
public void actionPerformed(final ActionEvent pEvent)
{
SwingUtilities.invokeLater(this);
}
/**
* Implementation of the {@link Runnable} interface.
* This will get called by {@link SwingUtilities#invokeLater(Runnable)} when everything is ready to
* for us to do our processing. This method will call {@link #performAction}.
*/
public void run()
{
performAction();
}
/**
* An identifier for this context menu action. This identifier is used by the {@link TableContextMenu}
* to get resource information from the {@link net.sf.cuf.ui.SwingDecorator}. The returned identifier
* should be prefixed with {@link ContextMenuAdapter#getContextMenuKennung} to ensure uniqueness.
*
* This method must be implemented by child classes.
* @return a I18N identifier for this context menu action
*/
public abstract String getKennung();
/**
* The {@link TableContextMenu} will call this method just before poping up the menu
* in order to enable/disable the menu entries.
* All the necessary information is available via {@link #mAdapter}.
*
* This method must be implemented by child classes.
* @return true
if this action is able to execute
*/
public abstract boolean isEnabled();
/**
* Here the processing takes place when the user selects this context menu entry.
* All the necessary information is available via {@link #mAdapter}.
*
* This method must be implemented by child classes.
*/
public abstract void performAction();
}